Question
I wrote some code for this problem, but I can't seem to figure it out. Can someone help? _______________________________________________________________________________________________________________ Main Class: import java.util.*; import static
I wrote some code for this problem, but I can't seem to figure it out. Can someone help?
_______________________________________________________________________________________________________________
Main Class:
import java.util.*;
import static java.lang.System.*;
public class Main
{
public static void main(String[] args)
{
//A A A A B V S E A S A A
HistoList test = new HistoList();
test.addLetter('A');
test.addLetter('A');
test.addLetter('A');
test.addLetter('A');
test.addLetter('B');
test.addLetter('V');
test.addLetter('S');
test.addLetter('E');
test.addLetter('A');
test.addLetter('S');
test.addLetter('A');
test.addLetter('A');
System.out.println(test);
//A B C
test = new HistoList();
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
System.out.println(test);
//A B C A B C A B C A B C A B C
test = new HistoList();
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
test.addLetter('A');
test.addLetter('B');
test.addLetter('C');
System.out.println(test);
}
}
__________________________________________________________________________________________________________________
HistoList Class:
import java.util.*;
import static java.lang.System.*;
public class HistoList
{
private HistoNode front;
public HistoList( )
{
front = null;
}
//addLetter will add a new node to
//the front for let if let does not exist
//addLetter will bump up the count if let already exists
public void addLetter(char let)
{
}
//returns the index pos of let in the list if let exists
public int indexOf(char let)
{
return -1;
}
//returns a reference to the node at spot
private HistoNode nodeAt(int spot)
{
HistoNode current=null;
return current;
}
//returns a string will all values from list
public String toString()
{
String output = "";
return output;
}
}
__________________________________________________________________________________________________________________
HistoNode Class:
public class HistoNode
{
private char letter;
private int letterCount;
private HistoNode next;
public HistoNode(char let, int cnt, HistoNode n)
{
letter=let;
letterCount=cnt;
next=n;
}
public char getLetter()
{
return letter;
}
public int getLetterCount()
{
return letterCount;
}
public HistoNode getNext()
{
return next;
}
public void setLetter(char let)
{
letter=let;
}
public void setLetterCount(int cnt)
{
letterCount=cnt;
}
public void setNext(HistoNode n)
{
next = n;
}
}
__________________________________________________________________________________________________________________
Instructions:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
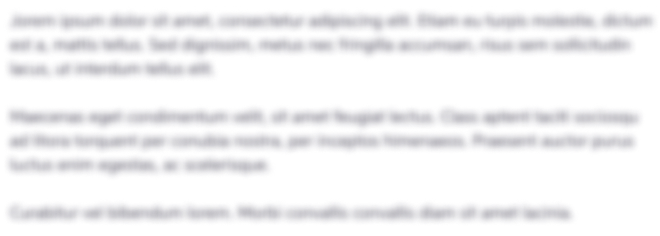
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started