Question
I wrote the following c++ program with the intent to enable operations on complex numbers and to enable input and output of complex numbers through
I wrote the following c++ program with the intent to enable operations on complex numbers and to enable input and output of complex numbers through the overloaded << and >> operators and to overload the multiplication * and division / operators. It has errors that i have not been able to solve. Please fix the errors in MS Visual Studio and return the code that is correct and able to run the program with no problems and attach photos of a sample output. I need to be able to copy and paste it into MSVS and have it run correctly. Thank you.
#include "stdafx.h"
#include
#ifndef COMPLEX_H
#define COMPLEX_H
class Complex
{
public:
explicit Complex(double = 0.0, double = 0.0);
Complex operator+(const Complex&) const;
Complex operator-(const Complex&) const;
Complex operator*(const Complex&) const;
Complex operator/(const Complex&) const;
Complex operator<<(const Complex&) const;
Complex operator>>(const Complex&) const;
friend ostream& operator << (ostream &out, const Complex &c);
friend istream& operator >> (istream &in, Complex &c);
std::string toString() const;
private:
double real;
double imaginary;
};
#endif
#include
#include "Complex.h"
using namespace std;
Complex::Complex(double realPart, double imaginaryPart):real{realPart}, imaginary{imaginaryPart}{}
Complex Complex::operator+(const Complex& operand2) const {
return Complex{ real + operand2.real, imaginary + operand2.imaginary };}
Complex Complex::operator-(const Complex& operand2) const {
return Complex(real - operand2.real, imaginary - operand2.imaginary);}
Complex Complex::operator*(const Complex& operand2) const {
return Complex(real * operand2.real, imaginary * operand2.imaginary);}
Complex Complex::operator/(const Complex& operand2) const {
return Complex(real / operand2.real, imaginary / operand2.imaginary);}
ostream& operator<<(ostream& out, Complex &operand2) {
out << operand2.real << " " << operand2.imaginary << endl;
return out.
};
istream& operator<<(istream& in, Complex &operand2){
in >> operand2.real;
in >> operand2.imaginary;
return in.
};
string Complex::toString() const {
return "("s + to_string(real) + ", "s + to_string(imaginary) + ")"s;
}
#include
#include "Complex.h"
using namespace std;
int main()
{
Complex x;
Complex y{ 1.0, 2.0 };
Complex z{ 3.0, 4.0 };
cout << "x: " << x.toString() << " y: " << y.toString() << " z: " << z;
x = y + z;
cout << "x = y + z: " << x.toString() << "=" << y.toString() << "+" << z.toString() << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
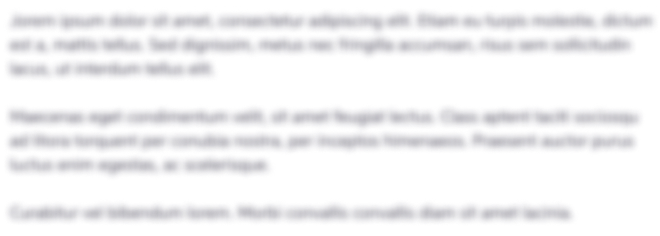
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started