Question
I wrote these three C-programs. The first one in bold searchs a linked list for a specific node specified by the user, the second program
I wrote these three C-programs. The first one in bold searchs a linked list for a specific node specified by the user, the second program deletes a node from a linked list specified by the user, and the third in bold inserts a new node at the beginning of the linked list. I need to somehow combine all of these programs into one single C-program which I am having trouble doing. Please help if you can.
#include
#include
struct node
{
int a;
struct node *next;
};
void generate(struct node **, int);
void search(struct node *, int);
void delete(struct node **);
int main()
{
struct node *head = NULL;
int key, num;
printf("Enter the number of nodes: ");
scanf("%d", &num);
printf(" Displaying the list ");
generate(&head, num);
printf(" Enter key to search: ");
scanf("%d", &key);
search(head, key);
delete(&head);
return 0;
}
void generate(struct node **head, int num)
{
int i;
struct node *temp;
for (i = 0; i < num; i++)
{
temp = (struct node *)malloc(sizeof(struct node));
temp->a = rand() % num;
if (*head == NULL)
{
*head = temp;
temp->next = NULL;
}
else
{
temp->next = *head;
*head = temp;
}
printf("%d ", temp->a);
}
}
void search(struct node *head, int key)
{
while (head != NULL)
{
if (head->a == key)
{
printf("key found ");
return;
}
head = head->next;
}
printf("Key not found ");
}
void delete(struct node **head)
{
struct node *temp;
while (*head != NULL)
{
temp = *head;
*head = (*head)->next;
free(temp);
}
}
#include
#include
// A linked list node
struct Node
{
int data;
struct Node *next;
};
void push(struct Node** head_ref, int new_data)
{
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void deleteNode(struct Node **head_ref, int position)
{
if (*head_ref == NULL)
return;
struct Node* temp = *head_ref
if (position == 0)
{
*head_ref = temp->next;
free(temp);
return;
}
for (int i=0; temp!=NULL && i temp = temp->next; if (temp == NULL || temp->next == NULL) return; struct Node *next = temp->next->next; free(temp->next); temp->next = next; } void printList(struct Node *node) { while (node != NULL) { printf(" %d ", node->data); node = node->next; } } int main() { struct Node* head = NULL; push(&head, 7); push(&head, 1); push(&head, 3); push(&head, 2); push(&head, 8); puts("Created Linked List: "); printList(head); deleteNode(&head, 4); puts(" Linked List after Deletion at position 4: "); printList(head); return 0; } #include #include struct Node{ int data; struct Node *next; }; struct Node* head; void Insert(int x) { struct Node* temp = (struct Node*)malloc(sizeof(struct Node)); temp->data=x; temp->next = head; head= temp; } void Print() { struct Node * temp= head; printf("List is: "); while(temp != NULL) { printf("%d ", temp->data); temp= temp->next; } printf(" "); } int main() { head =NULL; int n, i, x; printf("How many Numbers?: "); scanf("%d", &n); for(i= 0; i { printf("Enter the number: "); scanf("%d",&x); Insert(x); Print(); } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
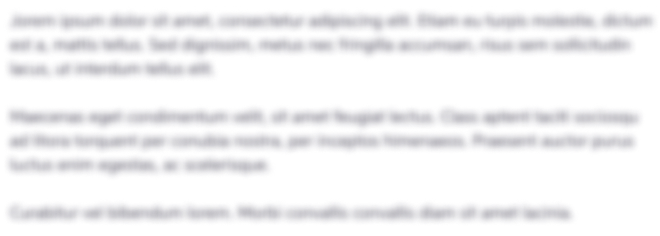
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started