Question
I wrote this code but there are some memory leaks. I need it debugged so that there are none (half of the code are screenshots
I wrote this code but there are some memory leaks. I need it debugged so that there are none (half of the code are screenshots and the rest was pasted because of the word count)
bool RemoveTail()
{
if (tail == nullptr)
return false;
Node *ptr = tail;
if(tail->prev != nullptr)
{
tail = tail->prev;
tail->next = nullptr;
}
else
{
tail = nullptr;
head = nullptr;
}
delete ptr;
ptr = nullptr;
length--;
return true;
}
bool RemoveAt(const unsigned int index)
{
if(index >= length || index
return false;
unsigned int ctr = 0;
Node *ptr = head;
while (ptr != nullptr && ctr
{
ptr = ptr->next;
ctr++;
}
if (ptr == nullptr)
{
return false;
}
else
{
if(ptr->prev != nullptr)
ptr->prev->next = ptr->next;
if(ptr->next != nullptr)
ptr->next->prev = ptr->prev;
delete ptr;
ptr = nullptr;
length--;
return true;
}
}
void AddNodesHead(const T arr[], const unsigned int count)
{
for (unsigned int i = 0; i
{
AddHead(arr[i]);
length++;
}
}
void AddNodesTail(const T arr[], const unsigned int count)
{
for (unsigned int i = 0; i
{
AddTail(arr[i]);
length++;
}
}
void InsertBefore(Node *node, const T data)
{
Node *front = head, *back = tail;
Node *ptr;
if (front == nullptr && back == nullptr)
{
AddHead(data);
return;
}
if (node == nullptr)
return;
while (front
{
if (front == node)
{
ptr = front;
break;
}
else if (back == node)
{
ptr = back;
break;
}
front = front->next;
back = back->prev;
}
Node *new_node = new Node;
new_node->data = data;
ptr->prev->next = new_node;
new_node->prev = ptr->prev;
new_node->next = ptr;
ptr->prev = new_node;
length++;
}
void InsertAfter(Node *node, const T data)
{
Node *front = head, *back = tail;
Node *ptr = nullptr;
if (front == nullptr && back == nullptr)
AddHead(data);
if (node == nullptr)
return;
while (front
{
if (front == node)
{
ptr = front;
break;
}
else if (back == node)
{
ptr = back;
break;
}
front = front->next;
back = back->prev;
}
Node *new_node = new Node;
new_node->data = data;
ptr->next->prev = new_node;
new_node->next = ptr->next;
new_node->prev = ptr;
ptr->next = new_node;
length++;
}
Node* GetNode(const unsigned int index)
{
Node *ptr = head;
for (unsigned int i = 0; i
{
ptr = ptr->next;
}
return ptr;
}
Node* Find(const T val)
{
Node *front = head, *back = tail;
Node *ptr = nullptr;
if (front == nullptr && back == nullptr)
{
return nullptr;
}
while (front
{
if (front->data == val)
{
ptr = front;
break;
}
else if (back->data == val)
{
ptr = back;
break;
}
front = front->next;
back = back->prev;
}
return ptr;
}
void InsertAt(const T data, const unsigned int index)
{
Node *ptr = head;
if (index > length)
return;
else if (index == 0)
AddHead(data);
else if (index == length - 1)
AddTail(data);
else
{
for (unsigned int i = 0; i
{
ptr = ptr->next;
}
Node *new_node = new Node;
ptr->prev->next = new_node;
new_node->prev = ptr->prev;
new_node->next = ptr;
ptr->prev = new_node;
}
}
void FindAll(std::vector
{
Node *ptr = head;
while(ptr != nullptr)
{
if(ptr->data == search_val)
nodes.push_back(ptr);
ptr = ptr->next;
}
}
T& operator[](const unsigned int index)
{
Node *ptr = head;
for (unsigned int i = 0; i
{
ptr = ptr->next;
}
return ptr->data;
}
private:
unsigned int length;
Node* head, *tail;
};
#endif
#ifndef LINKEDLIST H #define LINKEDLIST.H #includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
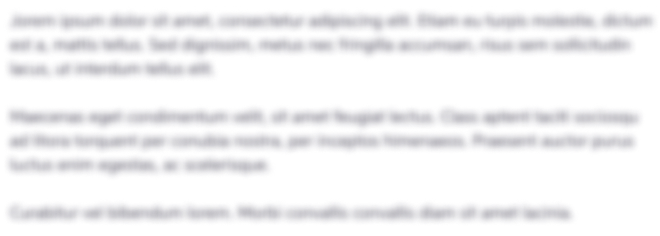
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started