Question
ICS 141. Programming with Objects Program 4 Due: 3/11/2018 Name: Points: 40 Goal: To write a program, that uses abstract classes and interfaces; uses text
ICS 141. Programming with Objects
Program 4
Due: 3/11/2018
Name:
Points: 40
Goal: To write a program, that uses abstract classes and interfaces; uses text menu to interact with users; to learn the temporary reference pointer casting (please refer to Week 4 lecture notes for detailed description); and to learn how to format output numbers in Java.
Program:
Write a program relates to personal bank accounts. I will explain what this means more below. Your program should use multiple classes, use abstract classes and interfaces, and use number output format (with Dollar signs) to display dollar amount with accuracy to two digits after the decimal point.
Bank Accounts
A bank account is either a checking account or a savings account. Some of the operations on these account types are the same, others are different. Each type of account has an owner name (represented by a string), and a balance. The balance of the account begins as $0.00, and can increase or decrease in well-defined ways.
For any bank account, you can deposit a sum of money. When you deposit an amount of money m, the balance in the account goes up by m. For any bank account, you can withdraw money; when you withdraw an amount n, the balance goes down by n. If you implement this feature using a method, then the method should return the amount you actually received. If you attempt to withdraw more money than is in the account, withdraw all the money in the account and set the balance to zero.
Additionally, for a savings account (but not a checking account), you can earn interest. If the interest rate is i (expressed as a fraction, so 1% = 0.01, for instance), then if there was m amount of money in the account before interest accrues, then the amount of money after crediting interest is m(1+i). The interest rate is 6% for savings account. The interest and the tax are calculated only once at the end of each run using the balance at the time when calculation is performed (the method is getYearEndBalance()).
Taxes
Savings accounts are a form of Taxable Entity. Taxable Entities have a fixed tax rate (20% of interest earned, not 20% of the total; we assume that only the interest is subject to taxation). You should model this entity as a Java interface named TaxableEntity. This interface contains just one constant: TAXRATE = 0.20. When calculate the final balance for a savings account, you should include interest earned (rate is 0.06) first and then have the tax deducted.
User Interface (UI) Requirement
This application will use a text oriented menu and Javas JOptionPanes popup windows to accept users inputs. I will supply the menu code and you are free to use it in other applications. The following is a brief description on the UI used in this program. First, the application will display a list of actions available (using plain text) as shown below:
Personal Banking System Menu
Make a Selection on:
1. Create a checking account
2. Create a savings account
3. Deposit Money
4. Get balance
5. Withdraw Money
6. Get yearend balance
Choose one (q to quit):
If a user chooses a valid action item (an integer number between 1 and 6), the program will perform the action selected (then redisplay the menu again); if a user selects an invalid selection, the program will display the message:
Invalid selection, Please choose again
Then, it will redisplay the menu; if a user chooses quit (typing the letter q), the program will display the message:
Bye!
Then, it will exit.
Design
Expect to have one application driver class TestAccountApplication. One abstract class Account. Two concrete classes: CheckingAccount and SavingsAccount. Both CheckingAccount and SavingsAccount are children of the abstract class Account. And one interface TaxableEntity that the SavaingsAccount class implements. In the following, I will briefly introduce some highlights of the design.
The application driver class TestAccountApplication handles all the UI stuff; the code presented in this class gives a text menu example that is representative in any applications that uses a text menu. The application class supplies the framework for our application and contains a special method called main(). This method is the entry point of our application.
In UML notation, an abstract class name should be italic. An interfaces name should be italic and its name should contain the annotation <
<
Comparable
ComparableRectangle
Class diagram
To help you see the overall structure of the application, we give you the class diagram in the space below:
TaxableEntity
<
TAXRATE=0.20
Account
CheckingAccount
name: String; type: String
balance: double
SavingsAccount
TestAccountApplication
SavingsAccount()
interestAmount()
taxAmount()
toString()
getYearEndBalance()
INTERESTRATE=0.06
CheckingAccount()
toString()
getYearEndBalance()
main()
Account(); deposit()
withdraw()
getBalance(); getType(); getName()
toString()
As you can see, the Account is an abstract class and contains one abstract method toString() in addition to other methods. In UML, an abstract class or an abstract method is represented with italic name (in our case, Account and toString() are italicized). The abstract Account class contains two constructors (shown only the parameter-less one). One constructor takes no parameter; the other takes three parameters. It also has six methods and one of them is an abstract method. The method toString() is defined as abstract method since we want all of its subclass to override it. The other five methods are regular (in Java, an abstract class can contain both regular and abstract methods) and can be used directly by its subclasses.
The interface TaxableEntity is used by the class SavingsAccount. This interface contains only a constant TAXRATE that is initialized to 0.20. Even though, in most instances, an interface will contain both constants and abstract methods.
There are two concrete working classes: CheckingAccount and SavingsAccount. Both classes are the children of the abstract class Account. There are two methods in the CheckingAccount class: toString() and getYearEndBalance(). toString() overrides the one in its parent. Thus it is polymorphic. The method getYearEndBalance() is non-polymorphic and exits only in the child classes (if invoking this method through a base pointer, we will need the temporary reference pointer casting). The SavingsAccount is more complicated than the CheckingAccount class. It contains four methods and one of them toString() is abstract. You are given the complete code for the CheckingAccount and are asked to write the code for SavingsAccount. When writing SavingsAccount, please refer to the above class diagram for number of methods to write (you should not repeat those methods that already exist in its parent unless they are necessary).
Required Work
In this assignment, we use scaffolded method. Thus, you are given large portion of code and will be asked to write portions of missing code according to the design and specific requirements in each individual section.
Supplied Partial Code
To help you get started, we give you following lists of code (you can cut and paste them to Eclipse):
1. The TestAccountApplication
The main functionality of the above application class is to interact with the user. It uses System.in.read() to accept users inputs from the keyboard and uses JOptionPanes popup windows to accept users inputs from a popup window. Thus, we include the swing package. It uses currency format. Thus, we include the NumberFormat package. It also offers the menu logic to interact with the user. Please note (in case 3) how the temporary reference point casting is used to call the parent method deposit(). List 1 gives the partial code for this class.
List 1: partial code for TestAccountApplication
-----------------------------------------------------------------------------------
import javax.swing.*;
import java.text.NumberFormat;
public class TestAccountApplication {
public static void main(String[] args) throws java.io.IOException {
String strName, strType, strAmt;
double amount;
NumberFormat fmt1 = NumberFormat.getCurrencyInstance();
char choice, ignore;
Object[] objArray = new Object[4];
int i = 0, index = 0;
for(;;) {
do {
System.out.println("Personal Banking System Menu");
System.out.println("Make a Selection on: ");
System.out.println(" 1. Create a checking account");
System.out.println(" 2. Create a savings account");
System.out.println(" 3. Deposit Money");
System.out.println(" 4. Get balance");
System.out.println(" 5. Withdraw Money");
System.out.println(" 6. Get yearend balance");
System.out.println();
System.out.println("Choose one (q to quit): ");
choice = (char) System.in.read();
// the effect of following do-while loop is to delay the processing
// until the user hits a return (then throw away all the characters
// except the first one).
do {
ignore = (char) System.in.read();
} while(ignore != ' ');
if(choice < '1' | choice > '6' & choice != 'q') {
System.out.println("Invalid selection, Please choose again ");
}
} while(choice < '1' | choice > '6' & choice != 'q');
if(choice == 'q') {
System.out.println("Bye!");
break;
}
System.out.println(' ');
switch(choice) {
case '1':
strName = JOptionPane.showInputDialog("Enter an account name:");
CheckingAccount cac = new CheckingAccount(strName, "checking", 0);
objArray[index++] = cac;
System.out.println("A new checking account is created and added to the system.");
break;
case '2':
// supply the missing code. In this case, the code will create a new
// SavingsAccount object and store it to the objArray. It should be
// similar to the code in case 1.
case '3':
strType = JOptionPane.showInputDialog(
"Enter an account type (checking/savings):");
strName = JOptionPane.showInputDialog("Enter an account name:");
for(i=0; i if(((Account)objArray[i]).getType().equals(strType) && ((Account)objArray[i]).getName().equals(strName)){ strAmt = JOptionPane.showInputDialog("Enter the amount to deposit:"); double m = Double.parseDouble(strAmt); ((Account)objArray[i]).deposit(m); System.out.println(strAmt + " is deposited into " + strName + " " + strType + " account"); break; } } if(i == index) { System.out.println("No " + strType + " account for " + strName); } break; case '4': strType = JOptionPane.showInputDialog( "Enter an account type (checking/savings):"); strName = JOptionPane.showInputDialog("Enter an account name:"); for(i=0; i if(((Account)objArray[i]).getType().equals(strType) && ((Account)objArray[i]).getName().equals(strName)){ double bl = ((Account)objArray[i]).getBalance(); System.out.println("The balance for " + strName + " in " + strType + " is: " + fmt1.format(bl)); break; } } if(i == index) { System.out.println("No " + strType + " account for " + strName); } break; case '5': // supply the missing code. In this case, the user wants to withdraw // money. Thus the code should first get information about the account // type; user name; and the amount to withdraw. The code will search // the objArray to see if the account exist? If exists, the code will call the // withdraw() method in the parent and return the result. Otherwise, // The code should output a message saying No ??? account for ??? // Here the ??? should be replaced by whatever the user inputed. You can // refer to case 4 when writing the code for this case. case '6': // supply the missing code. In this case, the user selected the action // of Get the yearend balance. } } } } ------------------------------------------------------------------------------------- In case 4, retrieving the balance, the output of the balance is formatted currency number with a prefix dollar sign followed by a double number with two digits after the decimal point. This is achieved by using the format object that is created from the CurrencyInstance. We use the NumberFormat object by importing the java.text.NumberFormat package first. You can get more information on how to use it by review the materials in week 4 lecture notes. 2. The Account Class The main functionality of the Account class is to serve as a central location for features that are common to all types of accounts. Thus it is defined as an abstract class. List 2 gives the partial code for this class. List 2: partial code for Account ----------------------------------------------------------------------------------- public abstract class Account { protected String name; protected String type; protected double balance; public Account(){ name = null; type = null; balance = 0.0; } public Account(String name, String type, double bl){ this.name = name; this.type = type; balance = bl; } public void deposit(double m){ balance = balance + m; } public double withdraw(double n){ //supply the missing code. The required behaviors are: // 1) the balance goes down by n and return the amount you actually received. // 2) If you attempt to withdraw more money than is in the account, withdraw // all the money in the account and set the balance to zero. } public String getName() {return name;} public String getType() {return type;} public double getBalance() {return balance;} public abstract String toString(); } ------------------------------------------------------------------------------------- 3. The CheckingAccount Class The CheckingAccount is one of the children that inherit Acount. When writing SavingsAccount, you can reference the code given here. List 3 gives the complete code for this class. List 3: complete code for CheckingAccount ----------------------------------------------------------------------------------- public class CheckingAccount extends Account { public CheckingAccount(String name, String type, double bl){ super(name, type, bl); } public double getYearEndBalance() { return getBalance(); } public String toString() { return getName() + ", " + getType() + ", " + getBalance(); } } ------------------------------------------------------------------------------------- This class gives an example of how to user super() to call a parent class constructor. In this case, we called the parents constructor that accepts 3 parameters. Output Requirements Your programs correctness will be judged on the output results. Thus, it is important to use the given test cases in some of output requirements. You will lose points if using different test data. Finishing Your Assignment You are asked to use Eclipse to finish this assignment. Here is what you need to do to finish the assignment. First, you create a new project (ICS141Program4) for this assignment. Second, build the project shell by cut and paste the code given to you (make sure you eliminate all the hidden characters after pasting (for double quotes, you need to delete and retype it inside Eclipse)). Third, you supply the missing code. Specifically, you need to supply the code for cases 2, 5, and 6 in the class TestAccountApplication; supply the withdraw() method in the class Account; supply the code for the SavingsAccount class; supply the code for the interface TaxableEntity. Finally, you run the program and do some screen captures (or record the output text from Eclipses output console window) of the resulting outputs. SCORE YOUR POINTS BY FINISHING THE FOLLOWING Important, the code you supplied in the following must match the given class diagram (for example, the name of classes and the name of methods must be the same; so do the relationships among these classes). 1. Cut and paste your Java source code for the withdraw() method in the abstract class Account in the space below (4 pts for finishing the missing code in withdraw()): Answer: 2. Cut and paste the entire code for the TestAccountApplication in the space below after you have finished code for case 2, 5, and 6. (10 pts): Answer: 3. Cut and paste your Java source code for class SavinsAccount in the space below. When implementing this class, you can refer to the code of CheckingAcount. This class has 4 methods (excluding constructors): interestAmount(), taxAmount(), getYearEndBalance(), and toString(). This class defines a constant INTERESTRATE = 0.6. Also, it inherits Account class and implements the TaxableEntity interface. Thus the first two lines of code should be: public class SavingsAccount extends Account implements TaxableEntity{ final double INTERESTRATE = 0.06; The interestAmount() should return a double value that represents the interest earned based on the balance at hand; this method should not change the balance. The taxAmount() method should return a double value that represents the tax amount based on the value return by interestAmount; this method should not change the balance. The getYearEndBalance() method should return a double value that represents the returned value of (balance + interest tax). This method should not change the balance. The toString() method is exactly the same as the one in CheckingAccount. (6 pts): Answer: 4. Cut and paste your Java source code for interface TaxableEntity in the space below (4 pts): 5. Overall Correctness Test (Scenario 1): you are asked to do a sequence of operations according the given test case; then either do a SCREEN CAPTURE or transcript the outputs from Eclipses output console window to the following designated space. Test Case 1 (a test case has two sets of data: one set of input data, the other set of known output data) You must use the following input test data: Create a checking account with name = Michael. Deposit $500. Deposit $4000. Withdraw $1500. Get balance Expected output (layout needs not be exact): Checking account information for Michael Name: Michael Balance: $3000.00 In the space blow, record the program output (either screen capture or text transcript; you only need to give the data for the last two steps) (6 pts): Answer: 6. Overall Correctness Test (Scenario 2 (assume start from afresh)): you are asked to do a sequence of operations according the given test case; then either do a SCREEN CAPTURE or transcript the outputs from Eclipses output console window to the following designated space. Test Case 2 (a test case has two sets of data: one set of input data, the other set of known output data (you figure out on your own)) You must use the following input test data: Create a checking account with name = aecy. Create a savings account with name = decy. Deposit $100 to decys savings account. Expected output (layout needs not be exact): Get yearend balance for decys savings account. In the space blow, record the program output (either screen capture or text transcript; you only need to give the data for the last two steps) (10 pts): Answer: Tips on how to capture an active window screen: a. you make a window active by click its title bar. b. hold Alt key and hit the Print Screen key (PrtSc). The Print Screen key should be in the top row of the keyboard, towards the right. If you want to capture the entire screen, just press the Print Screen key. c. Open a Word document. Move the cursor to the place where you want the screen capture to be inserted. Press Ctrl+V (hold Ctrl key and hit V). Turn in: This assignment (you must use this problem template) with all the boldfaced sections finished to the D2L dropbox.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
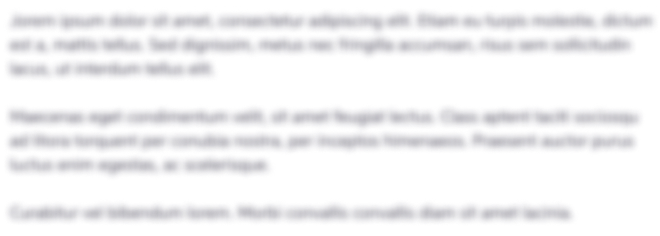
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started