Question
identify the functional & non functional requirements in this tic tac toe java code import java.util.ArrayList; import java.util.Arrays; import java.util.List; import java.util.Random; import java.util.Scanner; public
identify the functional & non functional requirements in this tic tac toe java code
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
import java.util.Scanner;
public class TicTacToe {
static ArrayList
static ArrayList
public static void main(String[] args) {
// TODO Auto-generated method stub
//create a game board
//printing game board
//3 spaces on the right & 3 spaces down (three rows and three columns)
//2D array of all symbols that we need for the tic tac toe
// symbols- char (characters)
char[][] gameBoard = {{' ', '|', ' ', '|',' '},
{'-', '+', '-', '+', '-'},
{' ', '|', ' ', '|', ' '},
{'-', '+', '-', '+', '-'},
{' ', '|', ' ', '|', ' '}};
printGameBoard(gameBoard);
// create a game loop
// get input from user
//store
//check if winner
while(true) {
Scanner scan = new Scanner(System.in);
System.out.println("Enter your Placement(1-9):");
int playerPos = scan.nextInt();
while(playerPositions.contains(playerPos) || cpuPositions.contains(playerPositions)) {
System.out.println ("position taken! Enter a correct position");
playerPos= scan.nextInt();
}
placePiece(gameBoard, playerPos, "player");
String result= checkWinner();
if (result.length() > 0) {
System.out.println(result);
break;
}
Random rand = new Random();
int cpuPos = rand.nextInt(9) + 1; //1-9
while(playerPositions.contains(cpuPos) || cpuPositions.contains(cpuPos)) {
cpuPos = rand.nextInt(9) + 1;
}
placePiece(gameBoard, cpuPos, "cpu"); //pas cpu position
printGameBoard(gameBoard);
result = checkWinner();
if (result.length() > 0) {
System.out.println(result);
break;
}
}
}
//print out the game board using 2 for loops
// for each row inside the game board
// for each char inside of the row
//print out symbol
// after each row print out line
//put for loop in method so it's not in the main method
public static void printGameBoard(char[][] gameBoard) {
for(char[] row : gameBoard) {
for (char c : row) {
System.out.print(c);
}
System.out.println();
}
}
public static void placePiece(char[][] gameBoard, int pos, String user) {
char symbol =' ';
if(user.equals("player")) {
symbol ='X';
playerPositions.add(pos);
} else if(user.equals("cpu")) {
symbol ='O';
cpuPositions.add(pos);
}
switch(pos) {
case 1:
gameBoard[0] [0] = symbol;
break;
case 2:
gameBoard[0] [2] = symbol;
break;
case 3:
gameBoard[0] [4] = symbol;
break;
case 4:
gameBoard[2] [0] = symbol;
break;
case 5:
gameBoard[2] [2] = symbol;
break;
case 6:
gameBoard[2] [4] = symbol;
break;
case 7:
gameBoard[4] [0] = symbol;
break;
case 8:
gameBoard[4] [0] = symbol;
break;
case 9:
gameBoard[4] [4] = symbol;
break;
default:
break ;
}
}
public static String checkWinner() {
List topRow= Arrays.asList(1, 2, 3);
List midRow= Arrays.asList(4, 5, 6);
List botRow = Arrays.asList(7, 8, 9);
List leftCol = Arrays.asList(1, 4, 7);
List midCol = Arrays.asList(2, 5, 8);
List rightCol= Arrays.asList(3, 6, 9);
List Cross1= Arrays.asList(1, 5, 9);
List Cross2= Arrays.asList(7, 5, 3);
List winning = new ArrayList
();
winning.add(topRow);
winning.add(midRow);
winning.add(botRow);
winning.add(leftCol);
winning.add(midCol);
winning.add(rightCol);
winning.add(Cross1);
winning.add(Cross2);
for(List l : winning) {
if(playerPositions.containsAll(l)) {
return "Congratulations you won!";
} else if (cpuPositions.containsAll(l)) {
return "CPU WIns!~ Sorry:(";
} else if(playerPositions.size() + cpuPositions.size() ==9) {
return "CAT!";
}
}
return "";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
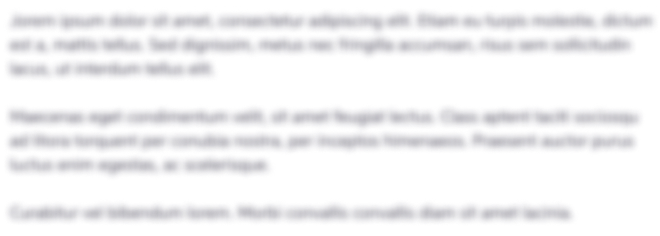
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started