Question
If someone could help me with this that would be awesome. These 2 programs go together and im not sure how that is done exactly.
If someone could help me with this that would be awesome. These 2 programs go together and im not sure how that is done exactly.
Design a class named Circle2D.java that contains the following specifications:
- Two double data fields named x and y that specify the center of the circle with set and get methods.
- A data field radius with a set and get method.
- A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius.
- A constructor that creates a circle with the specified x, y, and radius.
- A method getArea() that returns the area of the circle.
- A method getPerimeter() that returns the perimeter of the circle.
- A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See the Figure (a) below.
- A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See See the Figure (b) below.
- A method overlaps(Circle2D circle) that returns true if the specified circle overlaps with this circle. See the Figure (c) below.
FIGURE: (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another circle.
Below is the template code for Circle2D.java. You may edit the file as necessary to make the class work properly, but at the very least it must contain the constructors, methods and fields given below.
class Circle2D {
private double x, y;
private double radius;
public Circle2D() {
// Write your code here
}
public Circle2D(double x, double y, double radius) {
// Write your code here
}
public double getX() {
// Write your code here
}
public void setX(double x) {
// Write your code here
}
public double getY() {
// Write your code here
}
public void setY(double y) {
// Write your code here
}
public double getRadius() {
// Write your code here
}
public void setRadius(double radius) {
// Write your code here
}
public double getPerimeter() {
// Write your code here
}
public double getArea() {
// Write your code here
}
public boolean contains(double x, double y) {
// Write your code here
}
public boolean contains(Circle2D circle) {
// Write your code here
}
public boolean overlaps(Circle2D circle) {
// Write your code here
}
private static double distance(double x1, double y1, double x2, double y2) {
// Write your code here
}
}
Then create the TestCircle2D.java using the template code below for testing your Circle2D.java class.
public class TestCircle2D {
public static void main(String[] args) {
Circle2D c1 = new Circle2D(2, 2, 5.5);.
System.out.println("Area is " + c1.getArea());
System.out.println("Perimeter is " + c1.getPerimeter());
System.out.println(c1.contains(3, 3));
System.out.println(c1.contains(new Circle2D(4, 5, 10.5)));
System.out.println(c1.overlaps(new Circle2D(3, 5, 2.3)));
//For testing if two circles are touching each other: Circle2D c3 = new Circle2D(0, 0, 1); System.out.println(c3.overlaps(new Circle2D(2, 0, 1))); // True System.out.println(c3.contains(new Circle2D(2, 0, 1))); // False //For testing if two circles are identical: Circle2D c4 = new Circle2D(0, 0, 1); System.out.println(c4.overlaps(new Circle2D(0, 0, 1))); // True System.out.println(c4.contains(new Circle2D(0, 0, 1))); // True
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
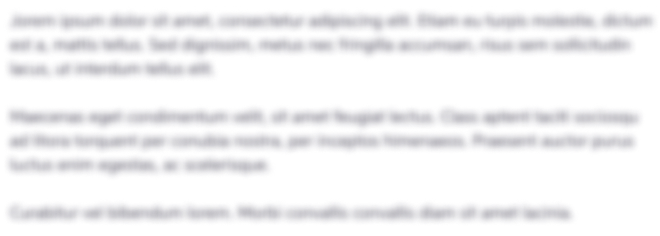
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started