Question
#ifndef BST_H #define BST_H // Representation of an element in the tree struct Node { int val; // Value of the node Node *left; //
#ifndef BST_H
#define BST_H
// Representation of an element in the tree
struct Node {
int val; // Value of the node
Node *left; // Pointer to the left node
Node *right; // Pointer to the right node
Node *parent; // Pointer to the parent node
};
class BST {
// Public Definitions
public:
enum TraversalOrder { InOrderTrav, PreOrderTrav, PostOrderTrav };
// Public Functions/Variables
public:
BST(); // Constructor
virtual ~BST(); // Destructor
void Insert(int toInsert);
void Delete(int toDelete);
void Print(enum TraversalOrder);
// Private Functions/Variables
private:
Node *root;
Node* Search(int toFind); // Searche for a node in the tree
Node* Successor(Node *curr); // Find the successor of the given node
Node* Minimum(Node *curr); // Find the minimum node of the given subtree
Node* Maximum(Node *curr); // Find the minimum node of the given subtree
void Transplant(Node *u, Node *v); // Replace the subtree rooted at node u with the subtree rooted at node v
void InOrder(Node *curr); // Inorder traversal
void PreOrder(Node *curr); // Preorder traversal
void PostOrder(Node *curr); // Postorder traversal
};
#endif
--------------------------------------------------------------------
cpp file:
#include
using namespace std;
/**************************************************************** * CONSTRUCTOR * Creates an empty binary tree ****************************************************************/ BST::BST() { }
/**************************************************************** * DESTRUCTOR * Free all memory used by current tree ****************************************************************/ BST::~BST() { // Write code to remove and deallocate all nodes in the tree }
void BST::Insert(int toInsert) { // Write your code here }
void BST::Delete(int toDelete) { // Write your code here }
void BST::Transplant(Node *u, Node *v) { // Write your code here }
Node *BST::Successor(Node *x) { // Write your code here }
Node *BST::Minimum(Node *x) { // Write your code here }
Node *BST::Maximum(Node *x) { // Write your code here }
Node *BST::Search(int toFind) { // Write your code here }
void BST::Print(TraversalOrder Order) { if(Order==InOrderTrav) InOrder(root); else if(Order==PreOrderTrav) PreOrder(root); else if(Order==PostOrderTrav) PostOrder(root); }
void BST::PreOrder(Node *x) { // Write your code here }
void BST::InOrder(Node *x) { // Write your code here }
void BST::PostOrder(Node *x) { // Write your code here }
-------------------------------------------
main.cpp
#include
#include
#include "BST.h"
using namespace std;
int main(int argc,char **argv) {
// Create an empty Binary Search Tree
BST Tree;
// Using a fixed size buffer for reading content is not always safe,
// but ok here because we know how our input has to be:
char line[100];
// Main loop
while ( std::cin.getline(line,100) ) {
string str(line);
if ( str.size()==0 ) continue;
if ( str[0]=='e' ) return 0;
// Use cerr if you want to always print to the console, because cout
// will be redirected to the output file when calling the Grade05 script:
// cerr
if ( str.substr(0,2)=="in" ) {
Tree.Print(BST::InOrderTrav);
cout
}
else {
if ( str.substr(0,3)=="pre" ) {
Tree.Print ( BST::PreOrderTrav );
cout
}
else {
if (str.substr(0,4) == "post") {
Tree.Print(BST::PostOrderTrav);
cout
}
else {
int key;
stringstream convert_stm(str.substr(2, str.size()-1));
if ( !(convert_stm>>key) ) key = -1;
if (str.substr(0,1) == "i") Tree.Insert(key);
else if (str.substr(0,1) == "d") Tree.Delete(key);
}
}
}
}
return 0;
}
Description In this lab your goal is to implement standard operations on binary search trees, including insert and delete. See section 12.3 in the textbook. A sample class structure with empty methods, is given in the support code. You can either use the given class structure or create your own. In this assignment the keys are integers. You will use Grade04 to test your code. Your execution file name must be "BST.exe". Refer to the previous lab assignments for instructions on how to use the grading tool The input contains one of the following commands on a line i key: Insert key into the BST. For example, "i 2" means "Insert key 2 into the BST" d key: Delete key from the BST. For example, "d 2" means "Delete key 2 from the BST" Do nothing if the BST does not contain the key Output all keys in preorder post: Output all keys in postorder in: Output all keys in inorder Finish your program Examples of input and output Input 1 i 5 i 7 d 7 d 7 pre d 1 postStep by Step Solution
There are 3 Steps involved in it
Step: 1
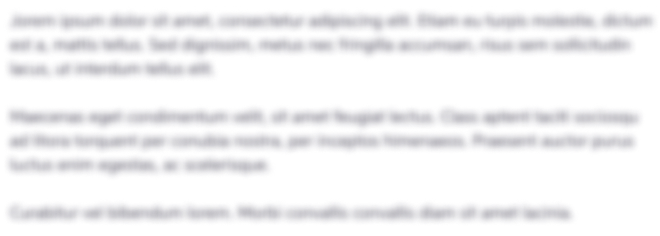
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started