Answered step by step
Verified Expert Solution
Question
1 Approved Answer
#ifndef COURSES_COURSES_H #define COURSES_COURSES_H #include #include #include #include #include #include enum class CompletionStatus {INCOMPLETE, WITHDRAWAL, COMPLETE}; class CourseRecord { public: CourseRecord(std::string name, double mark, int
#ifndef COURSES_COURSES_H
#define COURSES_COURSES_H
#include
#include
#include
#include
#include
#include
enum class CompletionStatus {INCOMPLETE, WITHDRAWAL, COMPLETE};
class CourseRecord {
public:
CourseRecord(std::string name, double mark, int yearTaken, CompletionStatus status)
: name(std::move(name)), mark(mark), yearTaken(yearTaken), status(status) {
}
std::string getName() const { return name; }
double getMark() const { return mark; }
int getYearTaken() const { return yearTaken; }
CompletionStatus getStatus() const { return status; }
std::string toString() const {
using namespace std::string_literals;
return "Course: "s + name + "; mark: " + std::to_string(mark)
+ "; year:" + std::to_string(yearTaken)
+ "; status:" + displayStatus(status);
}
static std::string displayStatus(CompletionStatus status) {
switch(status) {
case CompletionStatus::INCOMPLETE: return "Incomplete";
case CompletionStatus::WITHDRAWAL: return "Withdrawal";
case CompletionStatus::COMPLETE: return "Complete";
}
}
private:
std::string name;
double mark;
int yearTaken;
CompletionStatus status;
};
/*
* Represents results of courses taken by a student.
*/
class Courses {
public:
Courses(const std::vector& courseRecordsIn) {
for (auto rec: courseRecordsIn) {
courseRecords.insert({rec.getName(), rec});
}
}
/* Return the average marks per year for the given courses.
* Only include completes for the year.
*/
std::map averageSomeCoursesPerYear(
const std::set& courseNames) const {
std::map averagesPerYear;
// Complete this method
return averagesPerYear;
}
void print() const {
std::cout << "Courses: " << std::endl;
for (auto entry : courseRecords) {
std::cout << entry.second.toString() << std::endl;
}
}
private:
std::map courseRecords;
};
#endif
HELP ME TO COMPLETE THIS CODE
Step by Step Solution
There are 3 Steps involved in it
Step: 1
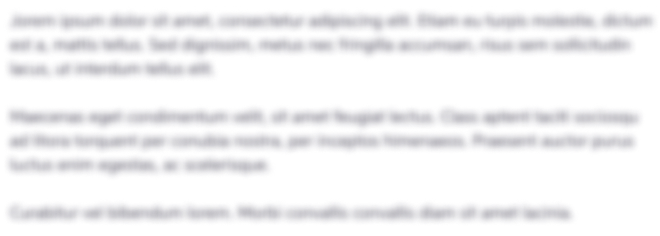
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started