Answered step by step
Verified Expert Solution
Question
1 Approved Answer
III. Maze Solver Go to https://www.cs.bu.edu/teaching/alg/maze/ and analyze the given algorithm Run the applet at the bottom of the page with the Algorithm box checked
- III. Maze Solver
- Go to https://www.cs.bu.edu/teaching/alg/maze/ and analyze the given algorithm
- Run the applet at the bottom of the page with the Algorithm box checked
- Implement the following maze search algorithm using recursion, use MazeSolver.java as the starting point (please notice that our algorithm does not unmark [x,y] if it is not in the solution path):
FIND-PATH(x, y)
- if ([x,y] outside maze) return false
- if ([x,y] is goal) return true
- if ([x,y] not open) return false
- mark [x,y] as part of solution path
- if (FIND-PATH(North of x,y) == true) return true
- if (FIND-PATH(East of x,y) == true) return true
- if (FIND-PATH(South of x,y) == true) return true
- if (FIND-PATH(West of x,y) == true) return true
- return false
public class MazeSolver { private char[][] maze; public MazeSolver(char[][] grid) { setMaze(grid); } public void setMaze(char[][] grid) { this.maze = new char[grid.length][]; for (int r = 0; r < grid.length; r++) { this.maze[r] = new char[grid[r].length]; for (int c = 0; c < grid[r].length; c++) this.maze[r][c] = grid[r][c]; } } private boolean findPath(int r, int c) { // TODO Project #5 return false; // THIS IS A STUB } private boolean isInsideMaze(int r, int c) { // TODO Project #5 return false; // THIS IS A STUB } private boolean isGoal(int r, int c) { return (this.maze[r][c] == 'G'); } private boolean isOpen(int r, int c) { // TODO Project #5 // ., S, or G would be considered open return false; // THIS IS A STUB }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
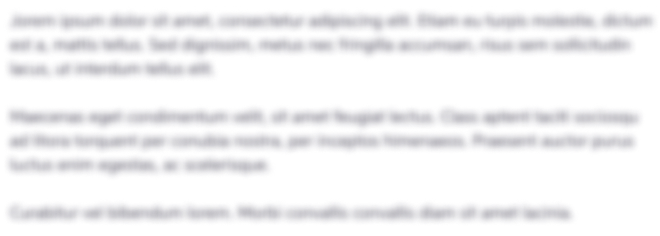
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started