Question
I'll add the code at the bottom. In java, create an Exception class named TooManyCritterException, which will extend Exception. The TooManyCritterException should have two constructors:
I'll add the code at the bottom.
In java, create an Exception class named "TooManyCritterException," which will extend Exception. The TooManyCritterException should have two constructors:
1) public TooManyCritterException(String message) -- This constructor should call the class Exception with the String parameter.
2) public TooManyCritterException(int AntNumber, intDoodlebugNumber, int Gridsize) -- This constructor has three paramters. If you use this constroctor to creat an instance of TooManyCritterException, e. e.getMessage() will output "Trying to assign [placeholder for AntNumber] ants, [placeholder for DoodlebugNumber] doodlebugs, to the world with the size of [placeholder for the world size]"
Use TooManyCritterException to replace the Exception created already. Use the second constructor to create the new Exception.
Make the main method:
1) Create a world with grid size 4x4
2) Use scanner to take a user's inputs for the and number and the doodlebug number. If the user inputs a too large number of critters (ant number + doodlebug number), throw the exception, and let the main method catch it, then let the user try again.
Sample output if user input was "10, 10, 4" : "Trying to assign 10 ants, 10 doodlebugs, to the world with the size of 4."
import java.util.Random; abstract public class Critter { protected World myWorld; // know in which world the critter lives protected int x, y; // Position in the world public void move(){ Random random = new Random(); int x_direction = random.nextInt(2); int y_direction = random.nextInt(2); inner_move(x_direction, y_direction); } private void inner_move(int x_direction, int y_direction) { int newX = x + x_direction; int newY = y + y_direction; if (myWorld.OutOfBound(newX, newY)){ return; } if (myWorld.getCell(newX, newY) == null) { myWorld.setCell(newX, newY, this); myWorld.setCell(x, y, null); this.x = newX; this.y = newY; } } abstract public void display(); public Critter(World world, int rowIndex, int columnIndex){ myWorld = world; x = rowIndex; y = columnIndex; } } -------------------------------------- public class Doodlebug extends Critter { public Doodlebug(World world, int rowIndex, int columnIndex) { super(world, rowIndex, columnIndex); } public void move(){ super.move(); } @Override public void display() { System.out.print("D "); } } ---------------------------------- import java.util.Random; public class Ant extends Critter { public Ant(World world, int rowIndex, int columnIndex) { super(world, rowIndex, columnIndex); } public void move(){ super.move(); } @Override public void display() { System.out.print("A "); } } ----------------------------------- import java.util.Random; public class World { private Critter[][] grid; // Array of organisms for each getCell public int getGridSize(){return grid.length;} public Critter getCell(int i, int j){ return grid[i][j]; } public void setCell(int x, int y, Critter c) { this.grid[x][y] = c; } public World(int gridsize){ grid = new Critter[gridsize][gridsize]; for(int i = 0; i < gridsize; i++){ for(int j = 0; j < gridsize; j++){ grid[i][j] = null; } } } public void Display(){ // loop through the 2-dimensional array: grid for (int i = 0; i < grid.length; i++){ // for each row of the grid // loop through each cell in the row for (int j = 0; j < grid[i].length; j++){ if (grid[i][j] == null){ // if the cell [i, j] is empty (==null) // print ". " System.out.print(". "); } else { // if the cell is not empty // call the method display of this cell's Critter instance grid[i][j].display(); } } // end of each row, print a newline character System.out.println(); } } public void AssignCritters(int antNumber, int doodlebugNumber) throws Exception { int totalNumber = antNumber + doodlebugNumber; // we assume that grid is a square if (totalNumber > grid.length * grid.length) { throw new Exception("Too many critters in this small world!"); } AssignCritters_inner(antNumber, "Ant"); AssignCritters_inner(doodlebugNumber, "Doodlebug"); } private void AssignCritters_inner(int critterNumber, String critterType) { Random random = new Random(); for (int i = 0; i < critterNumber; i++){ int rowIndex = random.nextInt(grid.length); int columnIndex = random.nextInt(grid[rowIndex].length); if(grid[rowIndex][columnIndex] == null){ if (critterType.equals("Ant")){ grid[rowIndex][columnIndex] = new Ant(this, rowIndex, columnIndex); } else if (critterType.equals("Doodlebug")){ grid[rowIndex][columnIndex] = new Doodlebug(this, rowIndex, columnIndex); } } else { i --; } } } public void oneStepSimulation(){ for (int i = 0; i < grid.length; i++){ for (int j = 0; j < grid.length; j++){ if (grid[i][j]!=null){ grid[i][j].move(); } } } } public boolean OutOfBound(int x, int y) { if (x >=0 && x < grid.length // x is in bound && y >= 0 && y < grid[x].length // y is in bound ){ return false; // NOT out of bound } else { return true; // YES, out of bound } } } ----------------------------------- public class Main { public static void main(String[] argv){ World world = new World(2); try { world.AssignCritters(2, 3); } catch (Exception e){ System.out.println(e.getMessage()); return; } finally { System.out.println("I'm in the finally block!"); } world.Display(); System.out.println(" "); world.oneStepSimulation(); world.Display(); } } -------------------------------- public class TooManyCritterException extends Exception { public TooManyCritterException(String message ) { Exception e = new TooManyCritterException(message); } public TooManyCritterException( int AntNumber, int DoodlebugNumber, int GridSize) { // Exception e = new TooManyCritterException("Trying to assign"+[AntNumber]+" ants, "+[DoodlebugNumber]+"doodlebugs, to the world with the size of "+[GridSize]); // System.out.println(e.getMessage()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
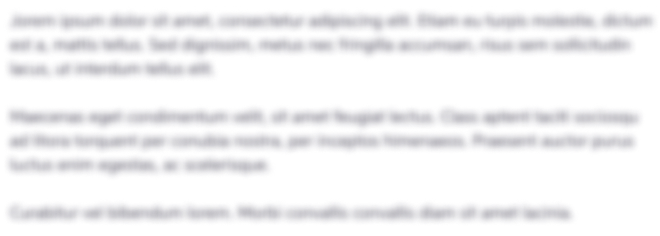
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started