Question
I'm attempting to code a program that takes the users input and creates a rectangle. The program will validate the users input is a valid
I'm attempting to code a program that takes the users input and creates a rectangle. The program will validate the users input is a valid rectangle and if so will output the Area and Perimeter. The first screenshot is the Test client and the second is the main. When I run Rectangle.java it compiles fine, but when I compile Test.java it says, "error: cannot find symbol boolean result = ValidRectangle (width, length);" It says the ValidRectangle, Area, and Perimeter could not be found. Help! Not sure why it does not automatically recognize the constructor in Rectangle.java
I've tried to google this error, but not finding much help. I thought that it would automatically recognize the constructor?
//Rectangle.java
import java.util.Scanner; public class Rectangle { /*Validates that the rectangle is acceptable*/ public static boolean ValidRectangle (double width, double length) { if (width + length > 30) return true; else return false; } /*Calculates the area of the rectangle*/ public static double Area (double width, double length) { return (width * length); } /*Calculates the perimeter of the rectangle*/ public static double Perimeter (double width, double length) { return (2 * (width + length)); }
}
//Test.java
import java.io.*; import java.util.Scanner; public class Test { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print ("Enter the width of the rectangle : "); double width = input.nextFloat(); System.out.print ("Enter the length of the rectangle : "); double length = input.nextFloat(); System.out.println ("Entered Width : "+width); System.out.println ("Entered length : "+length); boolean result = ValidRectangle (width, length); if(result==false) System.out.println("This is invalid rectangle. Try again..."); else { System.out.println("Area : "+Area (width, length)); System.out.println("Perimeter : "+Perimeter (width, length)); } } Exact Error: }Tool Output 1_cliant.-java:19: eTro: canno find ymbal boulea resul-ValidRectengle (wid legL) symbol mcthod validRectanglc (double, doublc) location: 1ass mehe rgh-Assignment.lclient. C:Usershayde Desktop\UC ciermonttuc Spring 2019\Prograring IIWeek 2 Rectanglereherghl assignmentl client.java: 26: exror: canno: find symbol 3ystem.out.printin Area+Area (width, length) ymbl: eLhod Area fdouble,duuble) location: class xeherghl Assiqnmentl Client .Users\hayde \ Desktop \UC Clermont\TC Spring 2019\Programming 11\Mosk 2\Roctanglehchc rghlAc51gnmentlClont.java:27: crror: cannot find syru yst.em.out.print.in"Perimeter: "+Perieter (width, length) syubol: method Perimeter (double,double) location: las5 mcherghl_assignmant.1_client. 3erors Tool completod vith oxit code 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
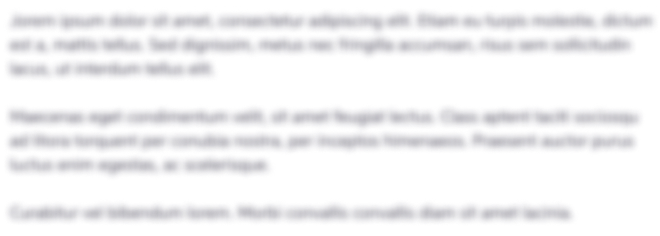
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started