Question
I'm attempting to make a calculator program that acts like a more advanced one, in that after answering an equation, if a new one is
I'm attempting to make a calculator program that acts like a more advanced one, in that after answering an equation, if a new one is started it carries over the previous answer. If someone cold help me complete the following code, that'd be great
The MyQueue class is implemented by using array, which requires an initial size. To let MyQueue dynamically grow or shrink its size, the enqueue() and dequeue() should have the ability to resize the array when it is necessary. Here is the dynamic size changing policy: - enqueue() increases the capacity to twice of the current size if the array becomes full. - dequeue() decreases the capacity to half of the current size if the number of elements is less than of the total capacity.
package application;
public class MyQueue {
private T[] arr; //used to store data into this array in a queue manner
private int total; //the total number of elements in the queue private int first; //the location of the first element in the queue private int rear; //the location of the next available element (last one's next)
//Default constructor, by default the capacity is two elements of type T public MyQueue() { arr = (T[]) new Object[2]; }
//Resize the MyQueue to the capacity as the input argument specifies private void resize(int capacity) { T[] tmp = (T[]) new Object[capacity]; //Implementation here... // copy items from arr[first...total) to tmp[0...total) // Note: first item could be anywhere in the array
// update arr, firt, rear } //Check if the queue is empty: if empty, returns true; otherwise returns false public boolean isEmpty() { //Implementation here... return false; }
//Add one element "ele" into the queue //Attention: (1) if the current queue is full, you need to resize it to twice of the current size. // (2) if the "rear" is already pointing to the end of the array, but there is available space // in the beginning, you need to "loop" the rear position. Recall operator "%". public void enqueue(T ele) { //Implementation here... }
//Delete the first (oldest) element from the queue and return this element. //Below is just an example code, you need to modify it. //Attention: (1) To save space, if the current number of elements is less than or equal to 1/4 of the // the capacity, shrink the capacity to 1/2 (half) of the original size. // (2) If the "first" is pointing to the end of the array, but there is available space // in the beginning, you need to consider "loop" the first position. Recall operator "%". public T dequeue() { //Implementation here... return null; } }
Also, I need help completing this postfix method
/* Process the postfix expression to compute the final value */ private String processPostfix(MyQueue postfix) { //Implementation here... //Below is just a start, you need to fill the values for final_value String final_value = ""; return final_value; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
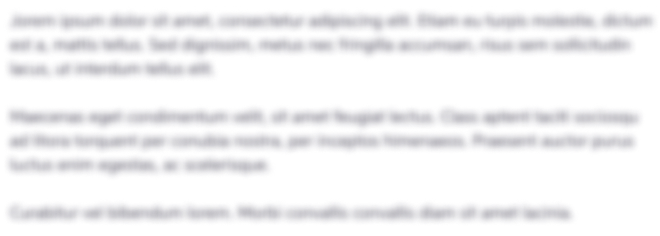
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started