Question
I'm having issues creating the generic item. If anyone could help me figure it out and explain it it would be great please! A1Work.java public
I'm having issues creating the generic item. If anyone could help me figure it out and explain it it would be great please!
A1Work.java
public class A1Work { /* * Create a generic Item
A1Driver.java
import java.util.Comparator;
public class A1Driver {
public static void main(String[] args) { System.out.println("----------"); System.out.println("kthLargest"); System.out.println("----------"); int[] arr = new int[] {1,2,3,4,5,6,7,8,9,10}; int k = 4; int correct = 7; int answer = A1Work.kthLargest(k, arr); System.out.println(((answer==correct)?"Correct":"Wrong") + " for k = "+k+" and correct = "+correct+" and your answer = "+answer); arr = new int[] {10,9,8,7,6,5,4,3,2,1}; k = 4; correct = 7; answer = A1Work.kthLargest(k, arr); System.out.println(((answer==correct)?"Correct":"Wrong") + " for k = "+k+" and correct = "+correct+" and your answer = "+answer); arr = new int[] {1,4,7,10,2,5,8,3,6,9}; k = 3; correct = 8; answer = A1Work.kthLargest(k, arr); System.out.println(((answer==correct)?"Correct":"Wrong") + " for k = "+k+" and correct = "+correct+" and your answer = "+answer); System.out.println("----------"); System.out.println("binaryOnes"); System.out.println("----------"); for(int i = 0; i < 10; i++) { answer = A1Work.binaryOnes(i); correct = Integer.bitCount(i); System.out.println(((answer==correct)?"Correct":"Wrong") + " for i = "+i+" and correct = "+correct+" and your answer = "+answer); }
System.out.println("-----------"); System.out.println("genericItem"); System.out.println("-----------"); //UNCOMMENT TO TEST /** / Item
Step by Step Solution
There are 3 Steps involved in it
Step: 1
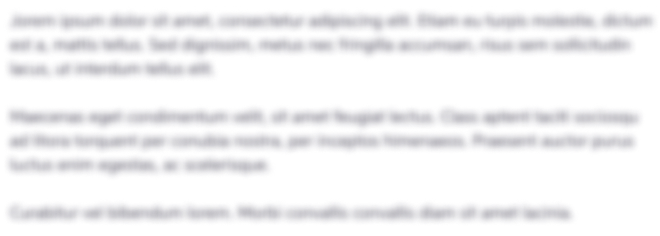
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started