Question
I'm having trouble finding the error in my code it plays a game that has some rules i am getting a slightly different output please
I'm having trouble finding the error in my code it plays a game that has some rules i am getting a slightly different output please help.
Your task is to write a program that plays the game of life. This game is a computer simulation of the life and death events in a population of single-cell organisms. Each position in a two-dimensional grid (Petri dish) can support one cell. The program determines whether each position will be able to support life in the next generation.
The grid of cells is represented by a boolMatrix object. In other words, you are writing a program that USES the boolMatrix class. No changes should be made to the code in boolmatrix.h or boolmatrix.cpp! (You'll be adding documentation to those files, of course, but no changes to the code unless you are correcting an error.) The starting grid is generation 0 and is read from a supplied input file. Most positions in the grid have 8 neighbors like the center square in a tic-tac-toe game. The four corner positions have only 3 neighbors each. The remaining positions around the edge of the grid have 5 neighbors each. The rules for the state of each position in the next generation of the grid are as follows:
If the cell is currently empty:
If the cell has exactly three living neighbors, it will come to life in the next generation.
If the cell has any other number of living neighbors, it will remain empty.
If the cell is currently living:
If the cell has one or zero living neighbors, it will die of loneliness in the next generation.
If the cell has four or more living neighbors, it will die of overcrowding in the next generation.
If the cell has two or three neighbors, it will remain living.
All births and deaths occur simultaneously. This point is critical to the correct result.
///Main.ccp
#include
#include
#include "boolmatrix.h"
#include
using namespace std;
static const int NUM_ROWS = 20;
static const int NUM_COLS = 20;
void firstgen(boolMatrix& generation);
void getNextGen(boolMatrix& generation, int liveNeighbors, int row, int col);
void fateDeadCell(boolMatrix& generation, int liveNeighbors, int row, int col);
void fateLiveCell(boolMatrix& generation, int liveNeighbors, int row, int col);
void checkOutBounds(int row, int col, int& liveNeighbors);
void printResults(boolMatrix& generation);
int tallyLiveNeighbors(const boolMatrix& generation, int& liveNeighbors, int row, int col);
int main(int numGen,int liveNeighbors,int row,int col)
{
boolMatrix generation;
cout << "How many generations in total?: ";
cin >> numGen;
firstgen(generation);
generation.print();
cout << endl << "Total alive in row 10 = " << generation.rowCount(10) << endl;
cout << "Total alive in col 10 = " << generation.colCount(10) << endl;
cout << "Total alive = " << generation.totalCount() << endl << endl;
for (int count = 1; count < numGen; count++)
{
getNextGen(generation, liveNeighbors, row, col);
printResults(generation);
}
system("pause");
return 0;
}
void firstgen(boolMatrix& gen)
{
ifstream infile("input.txt");
assert(infile);
int row, col;
infile >> row >> col;
while (infile) {
gen.set(row, col, true);
infile >> row >> col;
}
infile.close();
}
void getNextGen(boolMatrix& gen, int liveNeigh, int row, int col)
{
liveNeigh = 0;
for (int row = 0; row < NUM_ROWS; row++)
{
for (int col = 0; col < NUM_COLS; col++)
{
if (gen.get(row, col) == false) {
fateDeadCell(gen, liveNeigh, row, col);
}
else if (gen.get(row, col) == true) {
fateLiveCell(gen, liveNeigh, row, col);
}
}
}
}
int tallyLiveNeighbors(boolMatrix& gen, int& liveNeigh, int row, int col)
{
if (gen.get(row - 1, col - 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row - 1, col) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row - 1, col + 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row, col - 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row, col + 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row + 1, col - 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row + 1, col) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
else if (gen.get(row + 1, col + 1) == true) {
liveNeigh++;
checkOutBounds(row, col, liveNeigh);
}
return liveNeigh;
}
void fateDeadCell(boolMatrix& gen, int liveNeigh, int row, int col)
{
tallyLiveNeighbors(gen, liveNeigh, row, col);
if (liveNeigh == 3) {
gen.set(row, col, true);
}
else if ((liveNeigh < 3) || (liveNeigh > 3)) {
gen.set(row, col, false);
}
}
void fateLiveCell(boolMatrix& gen, int liveNeigh, int row, int col)
{
tallyLiveNeighbors(gen, liveNeigh, row, col);
if ((liveNeigh <= 1) || (liveNeigh >= 4)) {
gen.set(row, col, false);
}
else if ((liveNeigh == 2) || (liveNeigh == 3)) {
gen.set(row, col, true);
}
}
void checkOutBounds(int row, int col, int& liveNeigh)
{
if (((row - 1) < 0) || ((row + 1) > NUM_ROWS) || ((col - 1)< 0) || ((col + 1) < NUM_COLS))
{
liveNeigh--;
}
}
void printResults(boolMatrix& gen)
{
gen.print();
cout << "Total alive in row 10 = " << gen.rowCount(10) << endl;
cout << "Total alive in col 10 = " << gen.colCount(10) << endl;
cout << "Total alive = " << gen.totalCount() << endl << endl;
}
///boolmatrix.ccp
#include #include "boolmatrix.h" #include
int boolMatrix::totalCount(){
int total = 0;
for (int row = 0; row < NUM_ROWS; row++) {
for (int col = 0; col < NUM_COLS; col++) {
if (matrix1[row][col] == true) {
total++;
}
}
}
return total;
}
using namespace std;
int boolMatrix::neighborCount(int row, int col)const {
int total = 0;
int initialrow = row - 1;
if (initialrow <= 0)
initialrow = 0;
int lastrow = row + 1;
if (lastrow <= 0)
lastrow = 0;
int initialcol = 0;
if (initialcol <= 0)
initialcol = 0;
int lastcol = col + 1;
if (lastcol <= 0)
lastcol = 0;
for (int i = initialrow; i <= lastrow; i++) {
for (int j = initialcol; j <= lastcol; j++) {
if (i == row && j == col)
continue;
if (get(row, col) == true)
total++;
}
}
return total;
}
int boolMatrix::rowCount(int row)const{
assert(row >= 0 && row < NUM_ROWS);
int count = 0;
for (int col = 0;col < NUM_COLS;col++) {
if (matrix1[row][col]== true)
count++;
}
return count;
}
int boolMatrix::colCount(int col)const {
assert(col >= 0 && col < NUM_COLS);
int count = 0;
for (int row = 0;row < NUM_ROWS;row++) {
if (matrix1[row][col]== true)
count++;
}
return count;
}
bool boolMatrix::get(int row,int col)const{
assert(row >= 0 && row < NUM_ROWS);
assert(col >= 0 && row < NUM_COLS);
return matrix1[row][col];
}
void boolMatrix::set(int row,int col,bool set ){
assert(row >= 0 && row < NUM_ROWS);
assert(col >= 0 && row < NUM_COLS);
matrix1[row][col] = set;
}
boolMatrix::boolMatrix() {
for (int row = 0; row < NUM_ROWS; row++) {
for (int col = 0; col < NUM_COLS; col++) {
matrix1[row][col] = false;
}
}
}
void boolMatrix::print() {
for (int row = 0; row < NUM_ROWS; row++){
cout << endl;
for (int col = 0; col < NUM_COLS; col++) {
if (matrix1[row][col])
cout << "*";
else cout << " ";
}
}
}
///boolmatrix.h
class boolMatrix {
public:
boolMatrix();
int totalCount();
int neighborCount(int row, int col)const;
int colCount(int col)const;
int rowCount(int row)const;
bool get(int row, int col)const;
void set(int row,int col,bool set );
void print();
static const int NUM_ROWS = 20;
static const int NUM_COLS = 20;
private:
bool matrix1[NUM_ROWS][NUM_COLS];
};
//////////////Output I get
How many generations in total?: 5
* * * * * * * * * * *** * * * ** * * * * * * ** * *** ** ** ** ** * * * * * * * * * ** ** * * * *** * ** * * * *** * ** * ** ***** * * * * * * * * * ** * * * ** * * * * ** * Total alive in row 10 = 3 Total alive in col 10 = 4 Total alive = 100
Assertion failed: row >= 0 && row < NUM_ROWS, file c:\users\charles.brown\source epos\project18\project18\boolmatrix.cpp, line 66
////Correct output
01234567890123456789 0 1 * * 2 * * *** * 3 * **** * 4 * * * ** 5 * * ***** * 6 ** * * *** * 7 * *** * * 8 **** 9 * ****** 10* * * 11 * * ** * 12** **** *** 13 * *** ** * 14 * * * *** 15 ** *** ** ** 16 * * ** 17 18 19 Total alive in row 10 = 3 Total alive in col 10 = 1 Total alive = 95
Step by Step Solution
There are 3 Steps involved in it
Step: 1
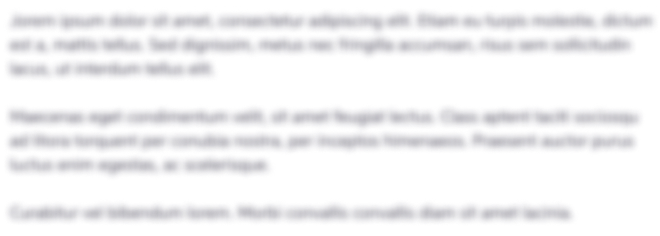
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started