Question
I'm having trouble with PostfixTester.java and PostfixEvaluator.java. I think my LinkedStack.java is correct but it may need fixing. All the code for PostfixTester and PostfixEvaluator
I'm having trouble with PostfixTester.java and PostfixEvaluator.java. I think my LinkedStack.java is correct but it may need fixing. All the code for PostfixTester and PostfixEvaluator is not required but some of it may help with the solution.
**PLEASE MAKE SURE OUTPUT IS THE SAME AS EXAMPLE OUPUT**
PostfixTester.java
import java.util.Scanner;
public class PostfixTester { /** * Reads and evaluates multiple postfix expressions. */ public static void main(String[] args) { String expression, again; int result; Scanner in = new Scanner(System.in); do { PostfixEvaluator evaluator = new PostfixEvaluator(); System.out.println("Enter a valid post-fix expression one token " + "at a time with a space between each token (e.g. 5 4 + 3 2 1 - + *)"); System.out.println("Each token must be an integer or an operator (+,-,*,/)"); expression = in.nextLine();
result = evaluator.evaluate(expression); System.out.println(); System.out.println("That expression equals " + result);
System.out.print("Evaluate another expression [Y/N]? "); again = in.nextLine(); System.out.println(); } while (again.equalsIgnoreCase("y")); } }
PostfixEvaluator.java
import java.util.Stack; import java.util.Scanner;
public class PostfixEvaluator { private final static char ADD = '+'; private final static char SUBTRACT = '-'; private final static char MULTIPLY = '*'; private final static char DIVIDE = '/';
private Stack
/** * Sets up this evalutor by creating a new stack. */ public PostfixEvaluator() { stack = new Stack
/** * Evaluates the specified postfix expression. If an operand is * encountered, it is pushed onto the stack. If an operator is * encountered, two operands are popped, the operation is * evaluated, and the result is pushed onto the stack. * @param expr string representation of a postfix expression * @return value of the given expression */ public int evaluate(String expr) { int op1, op2, result = 0; String token; Scanner parser = new Scanner(expr);
while (parser.hasNext()) { token = parser.next();
if (isOperator(token)) { op2 = (stack.pop()).intValue(); op1 = (stack.pop()).intValue(); result = evaluateSingleOperator(token.charAt(0), op1, op2); stack.push(new Integer(result)); } else stack.push(new Integer(Integer.parseInt(token))); }
return result; }
/** * Determines if the specified token is an operator. * @param token the token to be evaluated * @return true if token is operator */ private boolean isOperator(String token) { return ( token.equals("+") || token.equals("-") || token.equals("*") || token.equals("/") ); }
/** * Peforms integer evaluation on a single expression consisting of * the specified operator and operands. * @param operation operation to be performed * @param op1 the first operand * @param op2 the second operand * @return value of the expression */ private int evaluateSingleOperator(char operation, int op1, int op2) { int result = 0;
switch (operation) { case ADD: result = op1 + op2; break; case SUBTRACT: result = op1 - op2; break; case MULTIPLY: result = op1 * op2; break; case DIVIDE: result = op1 / op2; }
return result; } }
LinearNode.java
public class LinearNode
LinkedStack.java
public class LinkedStack
/** * Creates an empty stack. */ public LinkedStack() { count = 0; top = null; }
/** * Adds the specified element to the top of this stack. * @param element element to be pushed on stack */ public void push(T element) { LinearNode
temp.setNext(top); top = temp; count++; }
/** * Removes the element at the top of this stack and returns a * reference to it. * @return element from top of stack * @throws EmptyCollectionException if the stack is empty */ public T pop() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack");
T result = top.getElement(); top = top.getNext(); count--; return result; } /** * Returns a reference to the element at the top of this stack. * The element is not removed from the stack. * @return element on top of stack * @throws EmptyCollectionException if the stack is empty */ public T peek() throws EmptyCollectionException { if (isEmpty())
throw new EmptyCollectionException("stack");
return top.getElement(); }
/** * Returns true if this stack is empty and false otherwise. * @return true if stack is empty */ public boolean isEmpty() { return top == null; } /** * Returns the number of elements in this stack. * @return number of elements in the stack */ public int size() { return count; }
/** * Returns a string representation of this stack. * @return string representation of the stack */ public String toString() { LinearNode
String result = "";
while(temp != null) {
result = result + temp.getElement()+" ";
temp = temp.getNext(); } return result; } }
StackADT.java
public interface StackADT
EmptyCollectionException.java
public class EmptyCollectionException extends RuntimeException { public EmptyCollectionException(String collection) { super("The " + collection + " is empty."); } }
Create a new Java project called "Lab2". Complete the implementation of the LinkedStackStep by Step Solution
There are 3 Steps involved in it
Step: 1
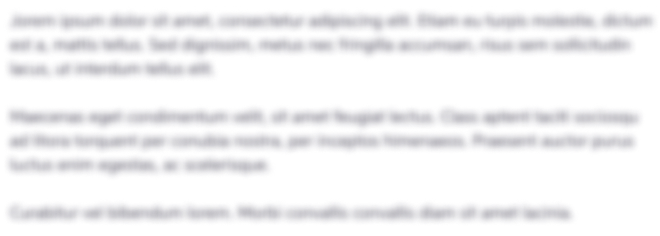
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started