Question
I'm in java one and need help with this code. please follow the givne directions and test your program to make sure it works as
I'm in java one and need help with this code. please follow the givne directions and test your program to make sure it works as it is required. I will apreciate your help.please follow stept by step the given directions. thank you.
For this assignment, you are going to write a Sudoku validator for 4x4 grids made up of 2x2 regions instead of 9x9 grids made up of 3x3 regions. Sudoku puzzles that use 4x4 grids only use the numerical digits 1 through 4 instead of 1 through 9.
Here are two correct 4x4 Sudoku grids:
3 | 2 | 4 | 1 |
4 | 1 | 3 | 2 |
1 | 4 | 2 | 3 |
2 | 3 | 1 | 4 |
4x4 Sudoku Example #1
1 | 2 | 3 | 4 |
3 | 4 | 1 | 2 |
2 | 1 | 4 | 3 |
4 | 3 | 2 | 1 |
4x4 Sudoku Example #2
Notice how each row, column and region has the individual numbers from 1 through 4 used only once. This means that the values used in each row, column and region must add up to 10 (1 + 2 + 3 + 4). This is how we will check our 4x4 Sudoku grids for this assignment.
Allow your user to enter their Sudoku grids row-by-row, separating each of the four values by a space and hitting ENTER at the end of the row.
When the user has entered all 16 values into the program, you should output validation checks for each region, row and column. An example run can be seen below with sample user input underlined.
Welcome to the Sudoku Checker v1.0!
This program checks simple, small, 4x4 Sudoku grids for correctness. Each column, row and 2x2 region contains the numbers 1 through 4 only once.
To check your Sudoku, enter your board one row at a time, with each digit separated by a space. Hit ENTER at the end of a row.
Enter Row 1: 3 2 4 1 Enter Row 2: 4 1 3 2 Enter Row 3: 1 4 2 3 Enter Row 4: 2 3 1 4
REG-1:GOOD REG-2:GOOD REG-3:GOOD REG-4:GOOD
ROW-1:GOOD ROW-2:GOOD ROW-3:GOOD ROW-4:GOOD
COL-1:GOOD COL-2:GOOD COL-3:GOOD COL-4:GOOD
SUDO:VALID
Your program does not have to check for the numbers 1 through 4 being used uniquely in each row, column and region. In other words, don't worry about validating their input to make sure they only enter the digits 1, 2, 3 or 4 and only enter them once per row, column and region. At this point in the semester, writing data validation like that would be incredibly painful.
You simply need to check that each row, column and region adds up to 10.
This simplistic type of checking will, however, allow some bad Sudoku grids to be validated as good. See two examples of this below. For this program, we will let these instances slide and not worry about them.
4 | 1 | 1 | 4 |
1 | 4 | 4 | 1 |
1 | 4 | 4 | 1 |
4 | 1 | 1 | 4 |
Invalid 4x4 Sudoku That Will Be Labeled as valid, Example #1
10 | 0 | 0 | 0 |
0 | 0 | 10 | 0 |
0 | 10 | 0 | 0 |
0 | 0 | 0 | 10 |
Invalid 4x4 Sudoku That Will Be Labeled as valid, Example #2
Rows, columns and regions are identified as found below:
ROW1 |
ROW2 |
ROW3 |
ROW4 |
C O L 1 | C O L 2 | C O L 3 | C O L 4 |
REGION 1 | REGION 3 |
REGION 2 | REGUON 4 |
When producing your output, you must first validate the regions, then the rows and then the columns. Each region, row and column validation should appear on a line by itself using the identifiers REG-1, REG-2, REG-3, REG-4, ROW-1, ROW-2, ROW-3, ROW-4, COL-1, COL-2, COL-3, and COL-4.
Each identifier will be followed immediately by a colon and that colon will be followed immediately by the word GOOD or the word BAD depending on if that row/column/region passes or fails validation.
The final line of output should be the keyword SUDO, followed immediately by a colon, followed immediately by either the word VALID or the word INVALID.
Please see the example run and run the sample program a number of times to make sure you understand how output is expected to be displayed for this program.
UML DIAGRAM FOR CLASS NetID_SudokuChecker Create a class based on your UNO NetID. Replace NetID in the following example with your UNO NetID.
NetID_SudokuChecker |
- grid : Integer[4][4] |
constructor NetID_SudokuChecker ( ) + displayGrid ( ) + getGrid ( ) + checkGrid ( ) |
DISCUSSION OF CLASS NetID_SudokuChekcer What follows is a short description of what each data member represents and what each method does:
DISCUSSION OF THE FILE NetID_SudokuChecker.java You will need to implement your class methods in the file NetID_SudokuChecker.java. This file should follow the basic outline here: import java.util.Scanner; public class NetID_SudokuChecker { // put private data members here // put constructor here // put getGrid() here // put displayGrid () here // put checkGrid() here } A MAIN / TEST PROGRAM (NetID_SudokuCheckerTest.java) To test your implementation of the NetID_SudokuChecker class, use the following code for NetID_SudokuCheckerTest.java,changing NetID to your UNO NetID. public class NetID_SudokuCheckerTest { public static void main ( String args[] ) { NetID_SudokuChecker sudChecker = new NetID_SudokuChecker(); sudChecker.displayGrid(); // this should display a 4x4 grid of all 0s sudChecker.getGrid(); sudChecker.displayGrid(); // this should display your 4x4 grid that you just entered sudChecker.checkGrid(); } } SUMMARY OF FILES You will need to create two files for this assignment: . NetID_SudokuChecker.java, which will be modeled after the code example seen at the top of this page. . NetID_SudokuCheckerTest.java, which will contain the code exactly as seen above. Essentially, you will type in the code exactly as seen in this assignment for both files. You will then plug in the appropriate code into the methods of the NetID_SudokuChecker.java file. DO NOT CHANGE THE NAMES OF ANY OF THE methods OR THE CLASS ITSELF!!!!! Goals Implement a moderately straightforward class that creates three methods (a constructor and two other methods) to implement a Sudoku- checking data type using a UML specification as a guideline for creation of the class. Points to Think About Try compiling your class often, after adding maybe five or ten lines of code to your program. By doing this, youre less likely to have dozens of errors to have to fix after compilation. You may wish to set up the instance variable and shells for the methods themselves first and compile that code without any real code inside any of the methods. You can also set up the Tester class and try compiling that against your compiled SudokuChecker class. Then, go back and start filling in code for each of the methods in the SudokuChecker class. Grading Notes >> Method documentation is required for all methods other than main()and you must follow the Program Coding Standards handout posted to Blackboard for this course. Points will be deducted for missing or incomplete method documentation. >> Your methods must behave as outlined in this assignment. >> Make sure your output follows the format you see in the example solution provided. A portion of your grade is for following this format exactly. If you messed up some of the output on your previous Sudoku solution, now is the time to fix it. Output Example Sample Run (user input is underlined) Welcome to the Sudoku Checker v1.0! This program checks simple, small, 4x4 Sudoku grids for correctness. Each column, row and 2x2 region contains the numbers 1 through 4 only once. To check your Sudoku, enter your board one row at a time, with each digit separated by a space. Hit ENTER at the end of a row. Here is the grid as I see it now: 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 EnterRow1:1 2 3 4 EnterRow2:3 4 2 1 EnterRow3:1 2 3 4 EnterRow4:4 3 2 1 Here is the grid as I see it now: 1 2 3 4 3 4 2 1 1 2 3 4 4 3 2 1 Thank you. Now checking ... REG-1:GOOD REG-2:GOOD REG-3:GOOD REG-4:GOOD ROW-1:GOOD ROW-2:GOOD ROW-3:GOOD ROW-4:GOOD COL-1:BAD COL-2:BAD COL-3:GOOD COL-4:GOOD SUDO:INVALID sample output run 2 Welcome to the Sudoku Checker v1.0! This program checks simple, small, 4x4 Sudoku grids for correctness. Each column, row and 2x2 region contains the numbers 1 through 4 only once. To check your Sudoku, enter your board one row at a time, with each digit separated by a space. Hit ENTER at the end of a row. Here is the grid as I see it now: 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 EnterRow1: 3 2 4 1 EnterRow2: 4 1 3 2 EnterRow3: 1 4 2 3 EnterRow4: 2 3 1 4 Here is the grid as I see it now: 3 2 4 1 4 1 3 2 1 4 2 3 2 3 1 4 Thank you. Now checking ... REG-1:GOOD REG-2:GOOD REG-3:GOOD REG-4:GOOD ROW-1:GOOD ROW-2:GOOD ROW-3:GOOD ROW-4:GOOD COL-1:GOOD COL-2:GOOD COL-3:GOOD COL-4:GOOD SUDO:VALID |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
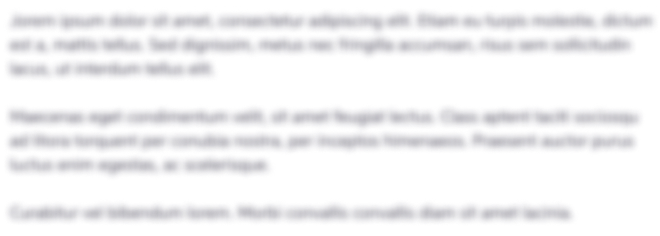
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started