Question
I'm in need helping implement Code Tictactoe Java. I have three classes need to implement . The last picture is the output Thank you so
I'm in need helping implement Code Tictactoe Java. I have three classes need to implement . The last picture is the output Thank you so much
The Model class . Prefer the pictures for all the explanation code Thanks
public class TicTacToeModel { private TicTacToeSquare[][] board; private boolean xTurn; private int dimension; public TicTacToeModel() { this(TicTacToe.DEFAULT_DIMENSION); } public TicTacToeModel(int dimension) { this.dimension = dimension; xTurn = true; board = new TicTacToeSquare[dimension][dimension];
/* Initialize board (fill with TicTacToeSquare.EMPTY) */ // INSERT YOUR CODE HERE } public boolean makeMark(int row, int col) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! } private boolean isValidSquare(int row, int col) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! } private boolean isSquareMarked(int row, int col) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! } public TicTacToeSquare getSquare(int row, int col) { // INSERT YOUR CODE HERE return null; // this is a stub; you should remove it later! } public TicTacToeState getState() { // INSERT YOUR CODE HERE return null; // this is a stub; you should remove it later! } private boolean isMarkWin(TicTacToeSquare mark) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! } private boolean isTie() { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! } public boolean isGameover() { return TicTacToeState.NONE != getState(); } public boolean isXTurn() { return xTurn; } public int getDimension() { return dimension; } @Override public String toString() { StringBuilder output = new StringBuilder(); // INSERT YOUR CODE HERE return output.toString(); } }
The View class
public class TicTacToeView { private final Scanner keyboard; public TicTacToeView() { keyboard = new Scanner(System.in); } public TicTacToeMove getNextMove(boolean isXTurn) { // INSERT YOUR CODE HERE (refer to the example output on Canvas!) return null; // this is a stub; you should remove it later!
} public void showInputError() {
System.out.println("Entered location is invalid, already marked, or out of bounds.");
} public void showResult(String result) {
System.out.println(result + "!");
} public void showBoard(String board) { System.out.println(" " + board); } }
Class Controller
public class TicTacToeController {
private final TicTacToeModel model; private final TicTacToeView view; public TicTacToeController(int dimension) {
model = new TicTacToeModel(dimension); view = new TicTacToeView(); }
public void start() { /* MAIN LOOP (repeats until game is over) */
// INSERT YOUR CODE HERE /* Display Results and Exit */
view.showBoard(model.toString());
view.showResult(model.getState().toString()); }
}
Square class :
public enum TicTacToeSquare { /*X*/ X("X"), /* O*/ O("O"), /* Empty Square*/ EMPTY(" "); private String message; private TicTacToeSquare(String msg) { message = msg; } @Override public String toString() { return message; } }
The State Class :
public enum TicTacToeState { /* The game is over, and X is the winner */ X("X"), /* The game is over, and O is the winner */ O("O"), /*The game has ended in a tie (no winner)*/ TIE("TIE"), /*The game is still in progress (no winner)*/ NONE("NONE"); private String message; private TicTacToeState(String msg) { message = msg; } @Override public String toString() { return message; } }
Output picture
Returns the current content and state of the Tic-Tac-Toe game board as a formatted String. This method must use a {@link StringBuilder) to compose the output String, which should not include any empty lines.
*Here is an example of how the output for a 3x3 game board should * appear (also see the example output on Canvas):
* 012 0 1 X 2 X
* @return the representation of the Tic-Tac-Toe game board * asee StringBuilder @Override public String toString() { 225 StringBuilder output = new StringBuilder(); 226 229 222 227 229 228 320 229 // INSERT YOUR CODE HERE 230 return output.toString(); 231 232 } 233 234 } 2 3 import java.util.Scanner; 4 5 6 * TicTacToeView implements a console-based View for the Tic-Tac-Toe game. 7 8 * Cauthor Your Name Oversion 1.0 9 19 public class TicTacToeView ( 11 12 13 private final Scanner keyboard; 14 15 16 17 * Constructor. This version of the Tic-Tac-Toe game uses the console for *I/0, so a (@link Scanner) connected to standard input is initialized to * receive the user's input from the console keyboard. Output will be * directed to standard output using the (@link System#out} output stream. 18 public TicTac ToeView() { keyboard = new Scanner(System.in); 19 20 21 22 23 24 25 26 27 28 29 30 31 } * Prompt the current player to enter his or her next move. Use iXTurn to * display an appropriate prompt for the current player. This method should: * receive the player's input (a row and column, separated by spaces), use * these values to initialize a new (@link TicTacToeMove) object, and then * return this object to the caller. 32 33 34 35 20 36 37 38 * @param isxturn a Boolean representing the current player: true if X, or * false if o * @return a {alink TicTacToeMove) value representing the player's * input *@ * see TicTacToeMove 59 public TictactoeMove getNextMovel boolean 1sxTurn) { 41 42 43 // INSERT YOUR CODE HERE (refer to the example output on Canvas!) return null; // this is a stub; you should remove it later! 45 } This method displays a descriptive error message if there was a problem * with an attempt to enter a move. This can happen if the specified * location is invalid, already marked, or out of bounds. public void showInputError() { 49 50 51 52 53 54 55 56 56 57 58 59 60 61 System.out.println("Entered location is invalid, already marked, or out of bounds."); } 62 63 * This method prints the final result of the Tic-Tac-Toe game to the * console: the current state, appended by an exclamation point, on a line by * itself. The result is provided as a string by the {@link TictactoeModel} * class's (@link TicTacToeModel#getState()} method; this method simply * outputs it to the console.) * 64 65 66 67 * @param result the result of the game, from {@link TicTacToeModel#getState()} public void showResult(String result) { 68 69 79 System.out.println( result + "!"); 71 } 72 73 74 76 /** * This method prints the current Tic-Tac-Toe game board to the console, * prepended by two blank lines. (The content of the game board is provided * as a String by the {@link TictactoeModel#toString()} method of the * {@link TictactoeModel} class; this method simply outputs it to the * console.) 78 79 80 * 81 82 * @param board the content of the game board, from {@link TicTacToeModel#toString()} * @see TicTacToeModel */ public void showBoard(String board) { 83 84 85 86 System.out.println(" " + board); 87 88 } 89 99 } 7 8 * @version 1.0 */ public class TictactoeController { 9 10 11 private final TicTacToeModel model; private final TicTacToeView view; 12 13 14 15 /** * Constructor. * Uses specified dimension to initialize Model and View 16 17 18 19 * @param dimension The dimensions/em> (width and height) of the new * Tic-Tac-Toe board. */ public TicTacToeController(int dimension) { 20 21 22 model = new TicTacToeModel(dimension); view = new TicTacToeView(); 23 24 25 } 26 /** 27 28 29 30 31 32 33 34 35 36 37 38 * Implements the main game loop, which repeats until the game is over. * This method should use {@link TicTacToeModel#isGameover()} to check if * the game is over; as long as the game is not over, the main loop should:
*- *
- Display the board using the {@link TicTacToeView#showBoard (java.lang. String)} method, *
- Get the next move from player using the {@link TicTacToeView#getNextMove(boolean)} method, *
- Attempt to make the player's mark using the {@link TicTacToeModel#makeMark(int, int)) method. * 01> *
- Display the board using the {@link TicTacToeView#showBoard (java.lang. String)} method, *
- Get the next move from player using the {@link TicTacToeView#getNextMove(boolean)} method, *
- Attempt to make the player's mark using the {@link TicTacToeModel#makeMark(int, int)) method. * 01> *
If the attempt failed, the {@link TicTacToeView#showInputError()} method * should be called to display an error message. The player should then be * prompted to enter another move until the attempt is successful.
*After the game is over, use the {@link TictactoeView&showBoard( java.lang.String)} method * to show the final state of the game board, and the {@link TicTac ToeView#showResult(java.lang.String)} method * to show the final result: either X or 0 wins, or a tie condition.
*/ 39 40 41 42 43 44 public void start() { 45 46 /* MAIN LOOP (repeats until game is over) */ 47 48 // INSERT YOUR CODE HERE 49 /* Display Results and Exit */ 50 51 52 view.showBoard (model.toString()); 53 54 view.showResult(model.getState().toString()); 55 } 56 57 58 } 012 0 1 2 Player 1 (x) Move: Enter the row and column numbers, separated by a space: 1 1 012 0 1 2 Player 2 (0) Move: Enter the row and column numbers, separated by a space: 0 1 012 0 1 X 2 Player 1 (x) Move: Enter the row and column numbers, separated by a space: 0 0 012 O xo 1 x 2 Player 2 (0) Move: Enter the row and column numbers, separated by a space: 1 2 012 1 XO 2 Player 1 (X) Move: 2 3 4 * TicTac ToeModel implements the Model for the Tic-Tac-Toe game. 5 6 7 8 9 * @author Your Name * aversion 1.0 */ public class TicTacToeModel { 19 11 12 12 * The contents of the Tic-Tac-Toe game board * private TicTacToeSquare] Il board; 14 15 16 17 18 19 * XTurn is true if X is the current player, or false if O is the current * player 29 private boolean xTurn; * The dimension (width and height) of the game board 21 22 23 24 25 26 27 28 29 private int dimension * Default Constructor (uses the default dimension) public TicTac ToeModel() { 30 31 32 this(Tictactoe. DEFAULT_DIMENSION); 34 } 35 36 37 Constructor (uses specified dimension) 39 40 41 42 43 * @param dimension The dimensions/em> (width and height) of the new * Tic-Tac-Toe board. */ public TicTacToeModel(int dimension) { /* Initialize dimension; X goes first */ 44 45 46 this.dimension dimension; xTurn = true; 47 48 /* Create board as a 2D TicTacToeSquare array */ 49 50 51 52 53 54 board = new TicTacToeSquare (dimension) (dimension); /* Initialize board (fill with TicTacToeSquare.EMPTY) */ // INSERT YOUR CODE HERE 1 55 56 57 58 59 60 61 62 63 64 # Use isValidSquare(int, int) to check if the specified square is in range, * and use 1sSquareMarked int, int) to check if the square is currently * empty. If both conditions are satisfied, create a mark in the square for * the current player, then toggle xTurn from true to false (or vice-versa) * to switch to the other player before returning TRUE. Otherwise, return * FALSE 65 F 66 67 68 * @param row the row (Y coordinatel of the square * @param col the column (x coordinate) of the square * areturn a Boolean value representing the result of the attempt to 68 * @return a Boolean value representing the result of the attempt to * make this mark: true if the attempt was successful, and false otherwise * see TicTac ToeSquare public boolean makeMark(int row, int col) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! 69 70 71 72 73 74 75 76 77 78 79 BO 81 82 83 84 } * Checks if the specified square is within range (that is, within the bounds * of the game board). * @param row the row (Y coordinate) of the square * @param col the column (x coordinatel of the square * @return a Boolean value: true if the square is in range, and false * if it is not 85 86 87 88 89 90 private boolean isValid Square(int row, int col) { // INSERT YOUR CODE HERE 91 92 93 return false; // this is a stub; you may need to remove it later! 94 95 } 96 97 * Checks if the specified square is marked. 98 99 190 191 192 * @param row * @param col * @return * if it is not the row (Y coordinate) of the square the column (X coordinate) of the square a Boolean value: true if the square is marked, and false 103 104 105 196 private boolean isSquareMarked(int row, int col) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! 197 109 108 109 110 111 112 113 174 } * Returns a {@link TicTacToeSquare) object representing the content of the * specified square of the Tic-Tac-Toe board. 115 116 117 +10 118 * @param row the row (Y coordinate) of the square y ) * @param col the column (X coordinate) of the square * @return the content of the specified square * @see Tictactoesquare 119 120 121 122 public TicTacToeSquare getSquare(int row, int col) { // INSERT YOUR CODE HERE return null; // this is a stub; you should remove it later! 123 124 125 126 127 128 129 130 131 132 133 134 * Use isMarkWin() to determine if X or o is the winner, if the game is a * tie, or if the game is still in progress. Return the current state of the * game as a (@link TicTacToeState} object. 12 134 135 * @return * @see the current state of the Tic-Tac-Toe game TicTacToeState 136 137 138 139 public TicTacToeState getState() { 140 // INSERT YOUR CODE HERE 141 142 return null; // this is a stub; you should remove it later! 143 ) 144 145 146 * Check the squares of the Tic-Tac-Toe board to see if the specified player * is the winner. 1 148 140 149 150 151 * @param mark the mark representing the player to be checked (X or 0) * @return true if the specified player is the winner, or false if not * see TicTac ToeSquare 152 153 154 private boolean isMarkWin(TicTacToeSquare mark) { // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! 155 156 157 158 159 160 161 162 163 } * Check the squares of the board to see if the Tic-Tac-Toe game is currently * in a tie state. 164 165 166 167 168 true if the game is currently a tie, or false otherwise * @return * private boolean is Tie() { 169 // INSERT YOUR CODE HERE return false; // this is a stub; you may need to remove it later! 170 171 172 173 174 174 175 } 176 177 178 179 * Uses {@link #getState() getState) to checks if the Tic-Tac-Toe game is * currently over, either because a player has won or because the game is * in a tie state. 180 * @return true if the game is currently over, or false otherwise 181 182 183 184 185 public boolean isGameover() { return TicTacToeState. NONE != getStatel); 186 } 187 188 189 190 191 * Getter for xTurn. * currently over, either because a player has won or because the game is * in a tie state. 178 179 180 181 182 183 * @return true if the game is currently over, or false otherwise public boolean isGameover() { 184 185 186 return TicTacToeState. NONE != getState(); 187 } 188 189 190 * Getter for xTurn. 191 192 193 * @return true if X is the current player, or false if 0 is the current * player */ public boolean isXTurn() { return xTurn; } 194 195 196 197 198 109 100 199 20 200 201 202 203 204 205 /** * Getter for dimension. * @return the dimensions/em> (width and height) of the Tic-Tac-Toe * game board */ public int getDimension() { 207 209 208 209 return dimension; 210 } 211 212 213 214 215 216 217 218 219 220 221 222 223 224 *Returns the current content and state of the Tic-Tac-Toe game board as a formatted String. This method must use a {@link StringBuilder) to compose the output String, which should not include any empty lines.
*Here is an example of how the output for a 3x3 game board should * appear (also see the example output on Canvas):
* 012 0 1 X 2 X
* @return the representation of the Tic-Tac-Toe game board * asee StringBuilder @Override public String toString() { 225 StringBuilder output = new StringBuilder(); 226 229 222 227 229 228 320 229 // INSERT YOUR CODE HERE 230 return output.toString(); 231 232 } 233 234 } 2 3 import java.util.Scanner; 4 5 6 * TicTacToeView implements a console-based View for the Tic-Tac-Toe game. 7 8 * Cauthor Your Name Oversion 1.0 9 19 public class TicTacToeView ( 11 12 13 private final Scanner keyboard; 14 15 16 17 * Constructor. This version of the Tic-Tac-Toe game uses the console for *I/0, so a (@link Scanner) connected to standard input is initialized to * receive the user's input from the console keyboard. Output will be * directed to standard output using the (@link System#out} output stream. 18 public TicTac ToeView() { keyboard = new Scanner(System.in); 19 20 21 22 23 24 25 26 27 28 29 30 31 } * Prompt the current player to enter his or her next move. Use iXTurn to * display an appropriate prompt for the current player. This method should: * receive the player's input (a row and column, separated by spaces), use * these values to initialize a new (@link TicTacToeMove) object, and then * return this object to the caller. 32 33 34 35 20 36 37 38 * @param isxturn a Boolean representing the current player: true if X, or * false if o * @return a {alink TicTacToeMove) value representing the player's * input *@ * see TicTacToeMove 59 public TictactoeMove getNextMovel boolean 1sxTurn) { 41 42 43 // INSERT YOUR CODE HERE (refer to the example output on Canvas!) return null; // this is a stub; you should remove it later! 45 } This method displays a descriptive error message if there was a problem * with an attempt to enter a move. This can happen if the specified * location is invalid, already marked, or out of bounds. public void showInputError() { 49 50 51 52 53 54 55 56 56 57 58 59 60 61 System.out.println("Entered location is invalid, already marked, or out of bounds."); } 62 63 * This method prints the final result of the Tic-Tac-Toe game to the * console: the current state, appended by an exclamation point, on a line by * itself. The result is provided as a string by the {@link TictactoeModel} * class's (@link TicTacToeModel#getState()} method; this method simply * outputs it to the console.) * 64 65 66 67 * @param result the result of the game, from {@link TicTacToeModel#getState()} public void showResult(String result) { 68 69 79 System.out.println( result + "!"); 71 } 72 73 74 76 /** * This method prints the current Tic-Tac-Toe game board to the console, * prepended by two blank lines. (The content of the game board is provided * as a String by the {@link TictactoeModel#toString()} method of the * {@link TictactoeModel} class; this method simply outputs it to the * console.) 78 79 80 * 81 82 * @param board the content of the game board, from {@link TicTacToeModel#toString()} * @see TicTacToeModel */ public void showBoard(String board) { 83 84 85 86 System.out.println(" " + board); 87 88 } 89 99 } 7 8 * @version 1.0 */ public class TictactoeController { 9 10 11 private final TicTacToeModel model; private final TicTacToeView view; 12 13 14 15 /** * Constructor. * Uses specified dimension to initialize Model and View 16 17 18 19 * @param dimension The dimensions/em> (width and height) of the new * Tic-Tac-Toe board. */ public TicTacToeController(int dimension) { 20 21 22 model = new TicTacToeModel(dimension); view = new TicTacToeView(); 23 24 25 } 26 /** 27 28 29 30 31 32 33 34 35 36 37 38 * Implements the main game loop, which repeats until the game is over. * This method should use {@link TicTacToeModel#isGameover()} to check if * the game is over; as long as the game is not over, the main loop should:
*- *
If the attempt failed, the {@link TicTacToeView#showInputError()} method * should be called to display an error message. The player should then be * prompted to enter another move until the attempt is successful.
*After the game is over, use the {@link TictactoeView&showBoard( java.lang.String)} method * to show the final state of the game board, and the {@link TicTac ToeView#showResult(java.lang.String)} method * to show the final result: either X or 0 wins, or a tie condition.
*/ 39 40 41 42 43 44 public void start() { 45 46 /* MAIN LOOP (repeats until game is over) */ 47 48 // INSERT YOUR CODE HERE 49 /* Display Results and Exit */ 50 51 52 view.showBoard (model.toString()); 53 54 view.showResult(model.getState().toString()); 55 } 56 57 58 } 012 0 1 2 Player 1 (x) Move: Enter the row and column numbers, separated by a space: 1 1 012 0 1 2 Player 2 (0) Move: Enter the row and column numbers, separated by a space: 0 1 012 0 1 X 2 Player 1 (x) Move: Enter the row and column numbers, separated by a space: 0 0 012 O xo 1 x 2 Player 2 (0) Move: Enter the row and column numbers, separated by a space: 1 2 012 1 XO 2 Player 1 (X) MoveStep by Step Solution
There are 3 Steps involved in it
Step: 1
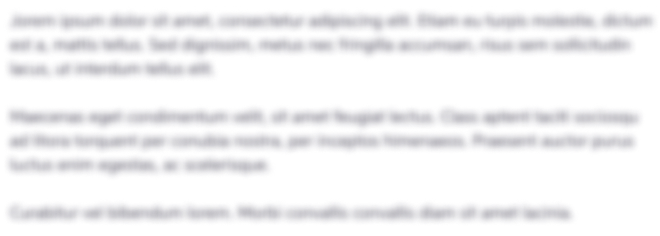
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started