Question
I'm learning Object-Oriented-Programming and using Java language. I've already created 4 class: Team, Game, BadmintonGame and SoccerGame. class Team public class Team { protected String
I'm learning Object-Oriented-Programming and using Java language. I've already created 4 class: Team, Game, BadmintonGame and SoccerGame.
class Team
public class Team { protected String nation; // Nation name protected int points; // Points of that nation // Initialise nation name public Team(String nation) { this.nation = nation; } // Returns string containing team information public String toString() { return "Nation: "+nation+" Points: "+points; } }
class Game
public abstract class Game { // Names of nations competing private String nation1; private String nation2; // Initialise names Game(String nation1, String nation2) { this.nation1 = nation1; this.nation2 = nation2; } // Sets names of both nations public void setNames(String nation1, String nation2){ this.nation1 = nation1; this.nation2 = nation2; } // Accessor methods public String Nation1(){ return nation1; } public String Nation2(){ return nation2; } // Returns a string containing names of both nations public String toString() { return "Nation 1: "+nation1+" Nation2: "+nation2; } // abstract method points public abstract int points(String nation); }
class BadmintonGame
public class BadmintonGame extends Game { private String set1, set2, set3; private String score; // Constructor to initialise nations BadmintonGame(String nation1, String nation2) { super(nation1, nation2); } // Constructor to initialise nations, sets and score BadmintonGame(String nation1, String nation2, String set1, String set2, String set3, String score) { super(nation1, nation2); this.set1 = set1; this.set2 = set2; this.set3 = set3; this.score = score; } // Accessor methods public String getSet1() { return set1; } public String getSet2() { return set2; } public String getSet3() { return set3; } public String getScore() { return score; } // Mutator methods public void setSet1(String set) { set1 = set; } public void setSet2(String set) { set2 = set; } public void setSet3(String set) { set3 = set; } public void setScore(String value) { score = value; } // Returns a string containing information about this game public String toString() { return super.toString()+" "+"Set 1: "+set1+" Set 2: "+set2+" Set 3: "+set3+" Score: "+score; } // Points method calculates the points of the given nation public int points(String nation) { int val1 = Integer.parseInt(score.substring(0,1)); int val2 = Integer.parseInt(score.substring(2)); if(nation.equals(super.Nation1())) { // If nation 1 wins if(val1 > val2) return 2; else return 0; } else { // If nation 2 wins if(val1 < val2) return 2; else return 0; } } }
class SoccerGame
public class SoccerGame extends Game { private String score; // Constructor to initialise nations SoccerGame(String nation1, String nation2) { super(nation1, nation2); } // Constructor to initialise nations and score SoccerGame(String nation1, String nation2, String score) { super(nation1, nation2); this.score = score; } // Accessor method public String getScore() { return score; } // Mutator method public void setScore(String value) { score = value; } // Returns a string containing information about this game public String toString() { return super.toString()+" "+"Score: "+score; } // Calculates points for a given nation public int points(String nation) { // for score format 6-3 i.e. goals int idx = score.indexOf('-'); int val1 = Integer.parseInt(score.substring(0, idx)); int val2 = Integer.parseInt(score.substring(idx+1)); if(val1 == val2) return 1; if(nation.equals(super.Nation1())) { // If nation 1 wins if(val1 > val2) return 3; else return 0; } else { // If nation 2 wins if(val1 < val2) return 3; else return 0; } } }
MY QUESTION IS:
By using single round-robin format.Please create GameTest class that uses text input-output files.
A round-robin format means there is no elimination of the teams whereby all teams will play with each other teams in turn
input filename : games.txt output filenames : badminton.txt, soccer.txt
Note that the actual input-output filenames may be different, so you need to prompt for the user filenames and not hardcoded all the filenames in your program.
1st line of games.txt will be the overall number of countries participating. 2nd line if games.txt will show the names of all the countries participating. The rest of the input file consist of the games and their results. (Note that each line can differ because it depends on the particular sport.)
Next create 2 arrays of Team to hold the data for these countries.
Team[] badmintonTeams = new Team[n]; Team[] soccerTeams = new Team[n];
Then create an array of Game to hold the games data for both sports. From there, create objects of either BadmintonGame or SoccerGame and calculate and total the points for each team in each sport.
badminton.txt file will store the results of each badminton game and print the winner of the badminton sport. (the country and the total points scored)
soccer.txt file will store the results of each soccer game and print the winner of the soccer sport. (the country and the total points scored)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
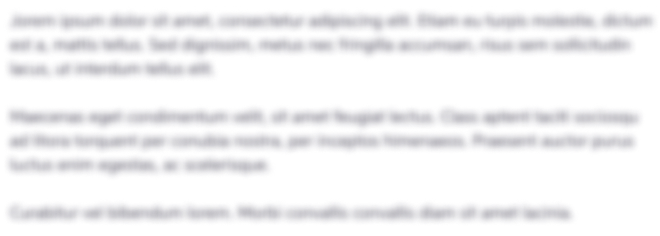
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started