Question
I'm stuck can't get this c++ code to run any idea where the error is? I'm suspecting that the problem is with the normalize function.
I'm stuck can't get this c++ code to run any idea where the error is? I'm suspecting that the problem is with the normalize function.
// Complete the following section // File Name: // Author: // Email Address: // Project Number: 2
//class Rational;
//private data: int n, (fraction numerator) and int d (fraction denominator).
//public interface: //constructors: //two int args, to allow setting rational to any legal value //one int arg, to construct rationals with arg numerator and denominator 1. //overload << and >> to allow writing to screen in form 325/430 //and reading it from the keyboard in the same format.
//Notes: either n or d may contain a negative quantity. //overload + - * / < <= > >= == //Put definitions in separate file for separate compilation
#include
class Rational { public: Rational(int numerator, int denominator); Rational(int numerator); // sets denominator to 1 Rational(); // sets numerator to 0, denominator to 1
friend Rational operator+(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend Rational operator-(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend Rational operator*(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend Rational operator/(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend bool operator<(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend bool operator<=(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend bool operator>(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend bool operator >=(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend bool operator ==(const Rational& rationalNum1, const Rational& rationalNum2);//complete the arguments); friend ostream& operator <<(ostream& outs, const Rational& rationalNum);//complete the arguments); friend istream& operator >>(istream& ins, Rational& rationalNum);//complete the arguments); private: int n; int d; }; void normalize(int &n, int &d); int gcd(int a, int b);
//Implementations of the members of class Rational.
//private members of class Rational // int n; // int d;
Rational::Rational(int numerator, int denominator) { normalize(numerator, denominator); n = numerator; d = denominator; }
//sets denominator to 1 Rational::Rational(int numerator) { //normalize(numer, denom); n = numerator; d = 1;//Complete the function }
// sets numerator to 0, denominator to 1 Rational::Rational() { n = 0; d = 1; //Complete the function }
Rational operator +(const Rational& rationalNum1, const Rational& rationalNum2) { Rational temp1; Rational temp2; Rational result; if (rationalNum1.d == rationalNum2.d) { result.n = rationalNum1.n + rationalNum2.n; result.d = rationalNum1.d; } else { temp1.n = rationalNum1.n * rationalNum2.d; temp1.d = rationalNum1.d * rationalNum2.d; temp2.n = rationalNum2.n * rationalNum1.d; temp2.d = rationalNum2.d * rationalNum1.d; result.n = temp1.n + temp2.n; result.d = temp1.d; } return result;//complete the arguments)
} Rational operator -(const Rational& rationalNum1, const Rational& rationalNum2) { Rational temp1; Rational temp2; Rational result; if (rationalNum1.d == rationalNum2.d) { if (rationalNum1.n > rationalNum2.n) { result.n = rationalNum1.n - rationalNum2.n; result.d = rationalNum1.d; } else { result.n = rationalNum2.n - rationalNum1.n; result.d = rationalNum1.d; } } else { temp1.n = rationalNum1.n * rationalNum2.d; temp1.d = rationalNum1.d * rationalNum2.d; temp2.n = rationalNum2.n * rationalNum1.d; temp2.d = rationalNum2.d * rationalNum1.d; if (temp1.n > temp2.n) { result.n = temp1.n - temp2.n; result.d = temp1.d; } else { result.n = temp1.n - temp2.n; result.d = temp1.d; } } return result; }
Rational operator *(const Rational& rationalNum1, const Rational& rationalNum2) { Rational temp; temp.n = rationalNum1.n * rationalNum2.n; temp.d = rationalNum1.d * rationalNum2.d; return temp; }
Rational operator/(const Rational& rationalNum1, const Rational& rationalNum2) { Rational temp; temp.n = rationalNum1.n * rationalNum2.d; temp.d = rationalNum1.d * rationalNum2.n; return temp; }
//precondition: all relational operators require d > 0 bool operator <(const Rational& rationalNum1, const Rational& rationalNum2) { bool temp; if ((rationalNum1.n * rationalNum2.d) < (rationalNum1.d * rationalNum2.n)) { temp = true; } else { temp = false; } return temp; }
bool operator <=(const Rational& rationalNum1, const Rational& rationalNum2) { bool temp; if (((rationalNum1.n * rationalNum2.d) < (rationalNum1.d * rationalNum2.n))||((rationalNum1.n * rationalNum2.d) == (rationalNum1.d * rationalNum2.n))) { temp = true; } else { temp = false; } return temp; }
bool operator >(const Rational& rationalNum1, const Rational& rationalNum2) { bool temp; if ((rationalNum1.n * rationalNum2.d) > (rationalNum1.d * rationalNum2.n)) { temp = true; } else { temp = false; } return temp; }
bool operator >=(const Rational& rationalNum1, const Rational& rationalNum2) { bool temp; if (((rationalNum1.n * rationalNum2.d) > (rationalNum1.d * rationalNum2.n))||((rationalNum1.n * rationalNum2.d) == (rationalNum1.d * rationalNum2.n))) { temp = true; } else { temp = false; } return temp; }
bool operator ==(const Rational& rationalNum1, const Rational& rationalNum2) { bool temp; if ((rationalNum1.n * rationalNum2.d) == (rationalNum1.d * rationalNum2.n)) { temp = true; } else { temp = false; } return temp; }
istream& operator >>(istream& in_str, Rational& right) { //Complete the function normalize(right.n, right.d); int topNumber; int bottomNumber; char one_char;
in_str >> topNumber; in_str >> one_char;
if (one_char == '/') { in_str >> bottomNumber; } else { bottomNumber = 1; } right.n = topNumber; right.d = bottomNumber;
return in_str; }
//The first parameter should not be const, the //second is read only and should be const.
ostream& operator <<(ostream& out_str, const Rational& right) { int topNumber; int bottomNumber; topNumber = right.n; bottomNumber = right.d; normalize(topNumber,bottomNumber); out_str << topNumber << '/' << bottomNumber; return out_str; }
//postcondition: return value is gcd of the absolute values //of m and n depends on function int abs(int); declared in //cstdlib
int gcd(int a, int b) { if (a == 0) return b; if (b == 0) return a; if (a == b) return a; if (a > b) return gcd(a-b, b); return gcd(a, b-a); }
//postcondition: n and d (to be numerator and denominator //of a fraction)have all common factors removed, and d > 0.
void normalize(int& n, int& d) { while (d > 0){ int divisor; divisor = gcd(n,d); n = n/divisor; d = d/divisor;
}
// remove common factors: //Complete the function
//fix things so that if the fraction is 'negative' //it is n that carries the sign. If both n and d are //negative, each is made positive. //Complete the function }
// Testing part is included in the main function. You do not need to write them int main() { cout << "Testing declarations" << endl; cout << "Rational x, y(2), z(-5,-6), w(1,-3), k(12,20);" << endl; Rational x, y(2), z(-5,-6), w(1,-3), k(12,20); cout << "x = " << x << ", y = " << y << ", z = " << z << ", w = " << w << ", k = " << k<< endl;
cout << "Testing >> overloading: Enter " << "a fraction in the format " << "integer_numerator/integer_denominator" << endl; cin >> x; cout << "You entered the equivalent of: " << x << endl; cout << z << " - (" << w << ") = " << z - w << endl;
cout << "Testing the constructor and normalization routines: " << endl; y =Rational(-128, -48); cout << "y =Rational(-128, -48) outputs as " << y << endl; y =Rational(-128, 48); cout << "y =Rational(-128, 48)outputs as " << y << endl; y = Rational(128,-48); cout << "y = Rational(128, -48) outputs as " << y << endl; Rational a(1,1); cout << "Rational a(1,1); a outputs as: " << a << endl; Rational ww = y*a; cout << y << " * " << a << " = " << ww << endl;
w = Rational(25,9); z = Rational(3,5); cout << "Testing arithmetic and relational " << " operator overloading" << endl; cout << w << " * " << z << " = " << w * z << endl; cout << w << " + " << z << " = " << w + z << endl; cout << w << " - " << z << " = " << w - z << endl; cout << w << " / " << z << " = " << w / z << endl;
cout << w << " < " << z << " = " << (w < z) << endl; cout << w << " < " << w << " = " << (w < w) << endl; cout << w << " <= " << z << " = " << (w <= z) << endl; cout << w << " <= " << w << " = " << (w <= w) << endl;
cout << w << " > " << z << " = " << (w > z) << endl; cout << w << " > " << w << " = " << (w > w) << endl; cout << w << " >= " << z << " = " << (w >= z) << endl; cout << w << " >= " << w << " = " << (w >= w) << endl;
w = Rational(-21,9); z = Rational(3,5); cout << w << " * " << z << " = " << w * z << endl; cout << w << " + " << z << " = " << w + z << endl; cout << w << " - " << z << " = " << w - z << endl; cout << w << " / " << z << " = " << w / z << endl; cout << w << " < " << z << " = " << (w < z) << endl; cout << w << " < " << w << " = " << (w < w) << endl; cout << w << " <= " << z << " = " << (w <= z) << endl; cout << w << " <= " << w << " = " << (w <= w) << endl;
cout << w << " > " << z << " = " << (w > z) << endl; cout << w << " > " << w << " = " << (w > w) << endl; cout << w << " >= " << z << " = " << (w >= z) << endl; cout << w << " >= " << w << " = " << (w >= w) << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
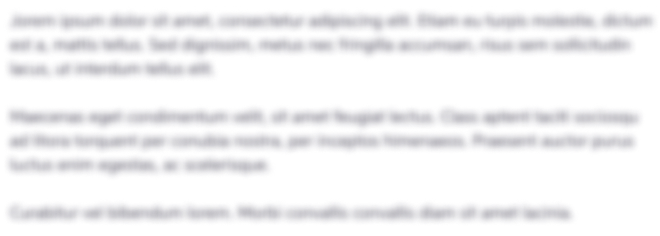
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started