Question
I'm stuck on a C++ problem. I have to build a singly-linked-list from an array and then remove a node from the linked-list. To remove
I'm stuck on a C++ problem. I have to build a singly-linked-list from an array and then remove a node from the linked-list. To remove the node I MUST pass the node one prior to the node I want to delete to a function(ex: If the linked-list holds {1, 2, 3, 4, 5} and I want to delete the node containing 3, I MUST pass the node containing 2 to the function removeAfterSingle). My code will delete from anywhere in the middle without issue, but I can't free the memory of the deleted node and I can't delete the first or last nodes. This is my code:
#include
If I uncomment delete[](temp); in the removeAfterSingle function then my IDE (Visual Studio) says there's a problem with the line if(ptr.next->num != NULL) in the while loop of main.
I've tried using if/else statements in the removeAfterSingle function to delete the last node but that just leads to nothing being deleted.
I'm haven't even begun to see how to remove the first node.
I'm not sure if I'm passing the node to function in the best way. I tried creating a pointer to a node and just passing the pointer like I would any other pointer but that never seemed to work correctly.
Please help!!!
Update: I think I'm good on the freeing the memory. Where I really need the help is when I need to delete the first and/or last nodes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
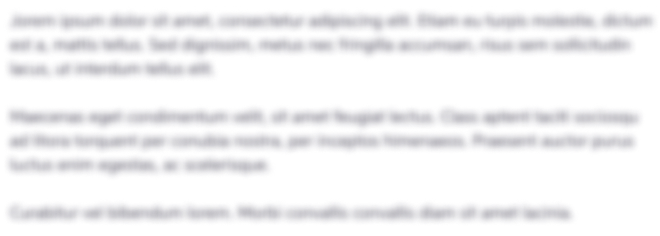
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started