Question
I'm supposed to add a car image.. How can I fix my code to do that on here? And lastly how can I add a
I'm supposed to add a car image.. How can I fix my code to do that on here?
And lastly how can I add a rectangular black background around my traffic lights (so that it looks more like a traffic light)?? I still need to see the pink background of course.
my car image: car.jpg
Here is my current code:
import java.awt.*; import javax.swing.*; import java.awt.event.*; import javax.swing.border.*;
public class TrafficLight extends JFrame implements ActionListener { JButton b1, b2, b3; Signal green = new Signal(Color.green); Signal yellow = new Signal(Color.yellow); Signal red = new Signal(Color.red);
public TrafficLight(){
super("Traffic Light"); /* We don't need to set any layout since by default Border Layout is set */ b1 = new JButton("STOP!"); b2 = new JButton("WAIT!"); b3 = new JButton("GO!"); b1.addActionListener(this); b2.addActionListener(this); b3.addActionListener(this); green.turnOn(false); yellow.turnOn(false); red.turnOn(true); JPanel p1 = new JPanel(new GridLayout(3,1)); p1.add(red); p1.add(yellow); p1.add(green); JPanel p2 = new JPanel(new FlowLayout()); p2.add(b1); p2.add(b2); p2.add(b3); getContentPane().add(p1, BorderLayout.CENTER); /*Adding Signals at the center of Border layout */ getContentPane().add(p2, BorderLayout.SOUTH); /*Adding Signals at the bottom of Border layout */ pack();
}
public static void main(String[] args){
TrafficLight tl = new TrafficLight(); tl.setVisible(true);
}
public void actionPerformed(ActionEvent e){
if (e.getSource() == b1){
green.turnOn(false); yellow.turnOn(false); red.turnOn(true);
} else if (e.getSource() == b2){
yellow.turnOn(true); green.turnOn(false); red.turnOn(false);
} else if (e.getSource() == b3){
red.turnOn(false); yellow.turnOn(false); green.turnOn(true);
}
}
}
class Signal extends JPanel{ Color on; int radius = 25; int border = 10; boolean change;
Signal(Color color){
on = color; change = true;
}
public void turnOn(boolean a){
change = a; repaint();
}
public Dimension getPreferredSize(){
int size = (radius+border)*2; return new Dimension( size, size );
}
public void paintComponent(Graphics g){
g.setColor( Color.pink ); g.fillRect(0,0,getWidth(),getHeight());
if (change){ g.setColor( on ); } else { g.setColor( on.darker().darker().darker() ); } g.fillOval( border,border,2*radius,2*radius ); }
class Car{ int year; String make; double speed;
public Car(int y,String m,double s){ this.year = y; this.make = m; this.speed = s; }
public double getSpeed(){ return this.speed; } public String getMake(){ return this.make; } public int getYear(){ return this.year; }
public void accelerate(){ this.speed+=1; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
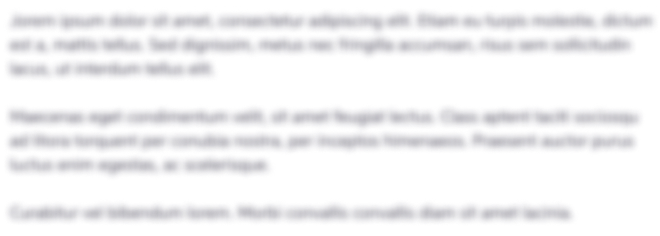
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started