Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I'm trying to implement pure virtual functions from multiple classes in a derived class but when I try to compile I'm given an error that
I'm trying to implement pure virtual functions from multiple classes in a derived class but when I try to compile I'm given an error that states that my final derived class is an abstract class because it does not implement the pure virtual functions. please help me understand how my syntax is wrong. Included below are the header and cpp files
// // Created by Anthony Neher on 7/8/18. // #ifndef LAB_6_SHAPES_H #define LAB_6_SHAPES_H #includeusing namespace std; class Shape { public: virtual void Scale(float scaleFactor) = 0; virtual void Display() const = 0; }; class Shape2D : virtual public Shape { public: virtual float Area() const = 0; virtual void ShowArea() const = 0; bool operator>(const Shape2D &rhs) const; bool operator<(const Shape2D &rhs) const; bool operator==(const Shape2D &rhs) const; }; class Shape3D : virtual public Shape { public: virtual float Volume() const = 0; virtual void ShowVolume() const = 0; bool operator>(const Shape3D &rhs) const; bool operator<(const Shape3D &rhs) const; bool operator==(const Shape3D &rhs) const; }; class Square :public Shape2D{ public: float s; virtual void Scale(float scaleFactor); virtual void Display(); virtual float Area(); virtual void ShowArea(); Square(float side); Square(); }; class Rectangle :public Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float w; float h; Rectangle(); Rectangle(float width, float height); }; class Triangle :public Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float b; float h; Triangle(); Triangle(float base, float height); }; class Circle :public Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float r; Circle(); Circle(float radius); }; class Ellipse :public Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float a; float b; Ellipse(); Ellipse(float a, float b); }; class Trapezoid : Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float a; float b; float h; Trapezoid(); Trapezoid(float area, float base, float height); }; class Sector : Shape2D{ public: void Scale(float scaleFactor); void Display(); float Area(); void ShowArea(); float r; float theta; Sector(); Sector(float radius, float degrees); }; class TriangularPyramid : public Shape3D{ public: void Scale(float scaleFactor); void Display(); float Volume(); void ShowVolume(); TriangularPyramid(); TriangularPyramid(float b, float height, float h); private: Triangle *triangle; float h; }; class RectangularPyramid : Shape3D{ public: void Scale(float scaleFactor); void Display(); float Volume(); void ShowVolume(); Rectangle *rectangle; RectangularPyramid(); RectangularPyramid(float l, float w, float h); private: float h; }; class Cylinder : Shape3D{ public: void Scale(float scaleFactor); void Display(); float Volume(); void ShowVolume(); Circle *circle; float h; Cylinder(); Cylinder(float radius, float height); }; class Sphere : Shape3D{ public: void Scale(float scaleFactor); void Display(); float Volume(); void ShowVolume(); Circle *circle; float r; Sphere(); Sphere(float radius); }; #endif //LAB_6_SHAPES_H
/////////////////////////////////////////////////////////////////////////////////////////////////////
// // Created by Anthony Neher on 7/8/18. // #include "Shapes.h" bool Shape3D::operator==(const Shape3D &rhs) const { if(this->Volume() == rhs.Volume()){ return true; } return false; } bool Shape3D::operator<(const Shape3D &rhs) const { if(this->Volume() < rhs.Volume()){ return true; } return false; } bool Shape3D::operator>(const Shape3D &rhs) const { if(this->Volume()>rhs.Volume()){ return true; } return false; } bool Shape2D::operator>(const Shape2D &rhs) const { if(this->Area()>rhs.Area()){ return true; } return false; } bool Shape2D::operator<(const Shape2D &rhs) const { if(this->Area()return true; } return false; } bool Shape2D::operator==(const Shape2D &rhs) const { if(this->Area()==rhs.Area()){ return true; } return false; } Square::Square() { s = 0; } void Square::Scale(float scaleFactor) { s = scaleFactor * s; } void Square::Display() { } float Square::Area() { return s*s; } void Square::ShowArea() { cout<float side) { s = side; } Rectangle::Rectangle() { w=0; h=0; } Rectangle::Rectangle(float width, float height) { w = width; h = height; } void Rectangle::ShowArea() { cout<float Rectangle::Area() { return h*w; } void Rectangle::Display() { } void Rectangle::Scale(float scaleFactor) { w = w*scaleFactor; h = h*scaleFactor; } Triangle::Triangle() { b = 0; h = 0; } Triangle::Triangle(float base, float height) { b = base; h = height; } void Triangle::Scale(float scaleFactor) { b = b*scaleFactor; h = h*scaleFactor; } void Triangle::Display() { } float Triangle::Area() { return b*h/2; } void Triangle::ShowArea() { cout<float radius) { r = radius; } void Circle::ShowArea() { cout<float Circle::Area() { return r*r*3.14; } void Circle::Display() { } void Circle::Scale(float scaleFactor) { r = r * scaleFactor; } Ellipse::Ellipse() { a = 0; b = 0; } Ellipse::Ellipse(float a, float b) { this->a=a; this->b= b; } void Ellipse::Scale(float scaleFactor){ a = a*scaleFactor; b=b*scaleFactor; } void Ellipse::Display() { } float Ellipse::Area() { return (float)3.14*a*b; } void Ellipse::ShowArea() {cout<float area, float base, float height) { a = area; b = base; h = height; } float Trapezoid::Area() { float area = (a+b)*h/2; return area; } void Trapezoid::ShowArea() { cout<void Trapezoid::Scale(float scaleFactor) { a = a*scaleFactor; b=b*scaleFactor; h=h*scaleFactor; } void Trapezoid::Display() { } Sector::Sector() { r = 0; theta = 0; } Sector::Sector(float radius, float degrees) { r = radius; theta = (degrees/360)*2*3.14; } void Sector::Display() { } float Sector::Area() { return r*r*theta/2; } void Sector::ShowArea() {cout<void Sector::Scale(float scaleFactor) { r=r*scaleFactor; } TriangularPyramid::TriangularPyramid() { triangle = new Triangle(0,0); h=0; } TriangularPyramid::TriangularPyramid(float b, float height, float h) { triangle = new Triangle(b,height); this->h=h; } void TriangularPyramid::Scale(float scaleFactor) { h=h*scaleFactor; triangle->Scale(scaleFactor); } void TriangularPyramid::Display() { } float TriangularPyramid::Volume() { return triangle->Area()*h; } void TriangularPyramid::ShowVolume() { cout< new Rectangle(0,0); h=0; } RectangularPyramid::RectangularPyramid(float l, float w, float h) { rectangle=new Rectangle(l,w); this->h=h; } void RectangularPyramid::Display() { } void RectangularPyramid::Scale(float scaleFactor) { h=h*scaleFactor; rectangle->Scale(scaleFactor); } float RectangularPyramid::Volume() { return rectangle->Area()*h/3; } void RectangularPyramid::ShowVolume() { cout< new Circle(0); h = 0; } Cylinder::Cylinder(float radius, float height) { circle =new Circle(radius); h=height; } float Cylinder::Volume() { return circle->Area()*h; } void Cylinder::ShowVolume() { cout< void Cylinder::Scale(float scaleFactor) { h=h*scaleFactor; circle->Scale(scaleFactor); } void Cylinder::Display() { } Sphere::Sphere(){ r=0; circle = new Circle(0); } Sphere::Sphere(float radius) { r = radius; circle =new Circle(r); } void Sphere::Display() { } float Sphere::Volume() { return 4*circle->Area()*r/3; } void Sphere::ShowVolume() { cout< void Sphere::Scale(float scaleFactor) { r=scaleFactor*r; circle->Scale(scaleFactor); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
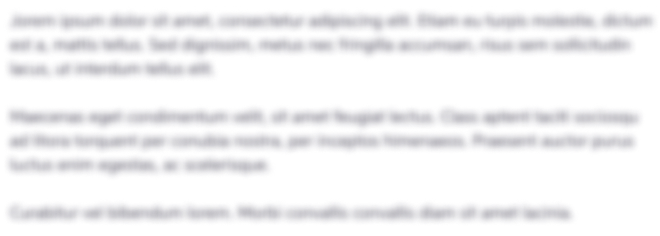
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started