Question
I'm trying to write a java program I need to write a program that will simulate the penny drop game, a box has six marked
I'm trying to write a java program
I need to write a program that will simulate the penny drop game, a box has six marked slots and the #6 slot is slightly larger. For 2 or more players, each player gets 12 Pennies. The object of the game is to be the first to get rid of all your pennies. Here are the rules:
1. Determine who goes first by each player rolling the single dice cube. Highest # goes first; then play proceeds in a clockwise direction.
2. Player rolls the die and must put a penny in the game board slot corresponding to the number shown on the die.
3. If the player rolls a number that already has a penny in it, they must take all of the pennies showing on the board and then the turn goes to the next player.
4. A player must take at least one roll, but can take as many rolls as they want until they get stuck as in rule #3.
5. Hole number 6 lets the penny go inside the box so you can roll as many as 6s as possible without penalty
6. Play continues until a player runs out of pennies and is declared the winner.
The pennies are redistributed to start the next game.
This is what I have so far
import java.util.Scanner; import java.util.Random;
public class PennyDropGame {
public static void main(String[] args) { // TODO Auto-generated method stub //We want to create a program that will simulate the Penny Drop Game Scanner kbd = new Scanner(System.in); System.out.print("Please Enter number of players "); int num = kbd.nextInt();
int i = 2, // 2 or more players up to 6 largestPlayer = 1, maxRand = (int) (Math.random() * 6 + 1), rand; System.out.println("Player1: "+ maxRand); while(i <= num) { rand = (int) (Math.random() * 6 + 1); System.out.println("Player" + i + ": "+ rand); if(rand > maxRand){ maxRand = rand; largestPlayer = i; } i++; if (largestPlayer == 7) System.out.println( "Too many players!" ); }
System.out.println("player "+largestPlayer+" goes first "); Random r = new Random(); int result = r.nextInt(6); // gives result 0-5 result = result + 1; System.out.println("player "+largestPlayer+ " rolled a " + result); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
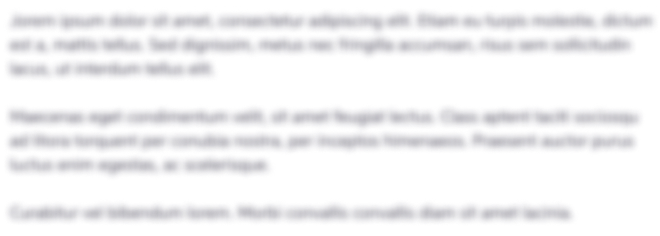
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started