Question
Im using c++, what's another way of writing this program out with out using the this ptr.. explanations would be great, also any feedback on
Im using c++, what's another way of writing this program out with out using the "this ptr".. explanations would be great, also any feedback on my code would be great! thank you
#include
#include
class Person
{
private:
std::string firstName;
std::string lastName;
int age;
std::string gender;
public:
// Default constructor
Person() {}
// Parameterized constructor
Person(std::string &firstName, std::string &lastName, int age, std::string &gender)
{
this->firstName = firstName;
this->lastName = lastName;
this->age = age;
this->gender = gender;
}
// Copy constructor {
Person(Person &other)
{
this->firstName = other.firstName;
this->lastName = other.lastName;
this->age = other.age;
this->gender = other.gender;
}
// Move constructor
Person(Person &&obj)
{
Person(obj);
// Call obj's destructor
obj.~Person();
}
// Destructor
~Person()
{
// Call std::string destructor
this->firstName.~basic_string();
this->lastName.~basic_string();
this->gender.~basic_string();
this->firstName = nullptr;
this->lastName = nullptr;
this->age = NULL;
this->gender = nullptr;
}
// Getters and setters.
std::string getFirstName() { return this->firstName; }
std::string getLastName() { return this->lastName; }
int getAge() {return this->age; }
std::string getGender() { return this->gender; }
// Setters
void setFirstName(std::string &firstName) {
this->firstName = firstName;
}
void setLastName(std::string &lastName) {
this->lastName = lastName;
}
void setAge(int age) {
this->age = age;
}
void setGender(std::string &gender) {
this->gender = gender;
}
// Overload copy operator
Person& operator=(Person &other) noexcept {
this->firstName = other.firstName;
this->lastName = other.lastName;
this->age = other.age;
this->gender = other.gender;
return *this;
}
// Overload move operator
Person& operator=(Person &&other) {
*this = other;
// Delete other
other.~Person();
return *this;
}
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
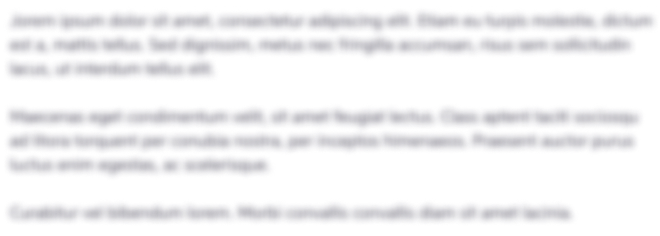
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started