Question
I'm working through Programming Challenge 8 for Chapter 13 in Starting out with Java Edition 6. My professor has requested that we use a multiple
I'm working through Programming Challenge 8 for Chapter 13 in Starting out with Java Edition 6. My professor has requested that we use a multiple interval selection list instead of check boxes for the workshop options. I am having trouble with the following areas:
1)How do I get the selected list items passed into an object/variable, assign the selected items a monetary value, and then total their values?
2)How do you a button that clears the selected content?
I've included my professor's instructions, what the GUI should like, and the code I've written. Thanks in advance for any help.
Instructions:
1) Break the program in reasonable classes and methods. Do not create only one class and write all statements in the main() method.
2) Save values given in the description in constants.
3) Should have a radio button selected by default
4) Multiple selections (contiguous and non-contiguous) in the list box are allowed. The program should be able to sum values from multiple selections. For more information about JCheckBox, read the book Chapter 12(12.6), P828-829, or JList, read the book Chapter 13(13.3), P864-868.
5) When the button Calculate Charges button is clicked, if no radio button and list item are selected, display a message, Please select type of registration and Please select workshop(s).
6) Should add a Clear button to clean all selections. You can use these methods, clearSelection()for the ButtonGroup object (not for an individual radio button) and the list, and setSelected() for the checkbox. Or you can check other methods in Java API documentation online.
7) Only create one listener class for multiple buttons to handle events.
8) Please notice that in this program, radio buttons, check box and list are used to get inputs, NOT used to trigger event. You only need to handle events triggered by the buttons.
My code:
//************************************************************** // Programmer: Jennifer Bailey // CTP 150 - Section 876 // Lab 7 //************************************************************* import javax.swing.*; import java.awt.*; import java.awt.event.*; import javax.swing.event.*; /** This class will create and display a description of the window. */ class Description extends JPanel { private JLabel description; //To display the description /** Constructor */ public Description() { //Create the label description = new JLabel("Select Registration Options"); //Add the label to the panel add(description); } } /** This class will create and display a radio button option for the user to choose if they're a student or general attendant. */ class AttendantType extends JPanel { public final double GENERAL = 895.0; //$895 for the general attendant public final double STUDENT = 495.0; //$495 for the student attendant private JRadioButton general; private JRadioButton student; private ButtonGroup attGroup; //radio button group for type of attenant /** Constructor */ public AttendantType() { //Create radio buttons general = new JRadioButton("General Registration", true); student = new JRadioButton("Student Registration"); //Group radio buttons attGroup = new ButtonGroup(); attGroup.add(general); attGroup.add(student); // Create a GridLayout manager. setLayout(new GridLayout(1, 2)); // Create a border. setBorder(BorderFactory.createTitledBorder("Registration Type")); // Add the radio buttons to this panel. add(general); add(student); } /** The getAttCost method returns the cost for the selected attendant registration. @return attCost One of the constants GENERAL or STUDENT will be returned. */ public double getAttCost() { double attCost = 0.0; if (general.isSelected()) attCost = GENERAL; else if (student.isSelected()) attCost = STUDENT; return attCost; } } /** This class will create and display a checkbox option for the user to choose if they want to attend an optional dinner and keynote speech. */ class DinnerSpeech extends JPanel { public final double DINNER = 30.0; //$30 per person cost for the dinner & speeach private JCheckBox dinnerSpeech; /** Contructor */ public DinnerSpeech() { //Create a Gridlayout manager setLayout(new GridLayout(1,0)); //Create checkbox dinnerSpeech = new JCheckBox("Dinner and Keynote Speech"); //Add checkbox to the panel add(dinnerSpeech); } /** The getDSCost method returns the cost for the dinner and keynote speech. @return attCost One of the constants GENERAL or STUDENT will be returned. */ public double getDSCost() { double keynoteCost = 0.0; if (dinnerSpeech.isSelected()) keynoteCost = DINNER; else keynoteCost = 0.0; return keynoteCost; } } /** This class creates the window for the workship registration */ public class EventCharge extends JFrame { public final double ECOMMERCE = 295.0; //cost of ecommerce workshop public final double FUTURE_WEB = 295.0; //cost of future of the web workshop public final double JAVA = 395.0; //cost of advanced java workshop public final double NET_SECURITY = 395.0; //cost of network security workshop private JList classList; //workshops //Create an array to hold the valus to be displayed in classList private String[] workshops = {"Introduction to E-commerce", "The Future of the Web", "Advanced Java Programming", "Network Security"}; private JButton calcButton; // To calculate the total cost private JButton clearButton; //To clear the user selections private JButton exitButton; // To exit the application private JPanel descriptionPanel; //Panel for the description private JPanel attPanel; //Panel for attendant type radio buttons private AttendantType type; private JPanel dinnerPanel; //Panel for the optional dinner speech private DinnerSpeech attendDinner; private JPanel workshopsPanel; //Panel for list of workshops private JPanel buttonPanel; //Panel for the buttons /** Constructor */ public EventCharge() { //Display a title setTitle("Conference Registration System"); //Specify an action for the close button setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //Create a BorderLayout manager setLayout(new BorderLayout()); //build the panels buildWorkshopsPanel(); buildButtonPanel(); //add panels to the content frame add(descriptionPanel, BorderLayout.NORTH); add(attPanel, BorderLayout.WEST); add(dinnerPanel, BorderLayout.CENTER); add(workshopsPanel, BorderLayout.EAST); add(buttonPanel, BorderLayout.SOUTH); // Pack and display the window. pack(); setVisible(true); } /** The buildWorkshopsPanel method will create and display a list that lets the user select multiple workshops either contiguously or non-contiguously */ public void buildWorkshopsPanel() { //create panel to hold the list workshopsPanel = new JPanel(); //create the list classList = new JList(workshops); //Set the selection mode classList.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION); //set the number of visible rows classList.setVisibleRowCount(4); //add list to the panel workshopsPanel.add(classList); } private void buildButtonPanel() { // Create the buttons calcButton = new JButton("Calculate Charges"); clearButton = new JButton("Clear"); exitButton = new JButton("Exit"); // Add an action listener to the buttons. calcButton.addActionListener(new CalcButtonListener()); clearButton.addActionListener(new ClearButtonListener()); exitButton.addActionListener(new ExitButtonListener()); //Put the buttons in their own panel. buttonPanel = new JPanel(); buttonPanel.add(calcButton); buttonPanel.add(clearButton); buttonPanel.add(exitButton); } /** CalcButtonListener is an action listener class for the calcbutton component. */ private class CalcButtonListener implements ActionListener { /** actionPerformed method @param e An ActionEvent object. */ public void actionPerformed(ActionEvent e) { double totalCost; //calculate the total charge totalCost = type.getAttCost() + attendDinner.getDSCost(); //+ total cost of workshops System.out.println("Attend cost: $" + type.getAttCost() + " Dinner cost: $" + attendDinner.getDSCost()+ //" Total Workshop Cost: $" + totalWorkshopCost + " Total cost: $" + totalCost); } } /** ClearButtonListener will handle clearing the info enter by the user when clicked */ private class ClearButtonListener implements ActionListener { /** actionPerformed method @param e An ActionEvent object. */ public void actionPerformed(ActionEvent e) { //write method to set attendant type to default setting attGroup.clearSelection(); //write method to uncheck dinner and speech checkbox attendDinner.setSelected(false); //write method to clear choices on workshop list } } /** ExitButtonListener is an action listener class for the exitButton component. */ private class ExitButtonListener implements ActionListener { /** actionPerformed method @param e An ActionEvent object. */ public void actionPerformed(ActionEvent e) { System.exit(0); } } /** The main method creates an instance of the EventCharge class and causes it to display the GUI window. */ public static void main(String[] args) { EventCharge person1 = new EventCharge(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
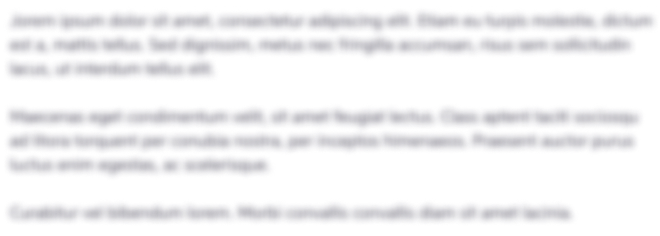
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started