Question
Implement a class Bank . This bank has two objects, checking and savings of type Account. Implement four member functions: deposit(double amount, string account) withdraw(double
Implement a class Bank. This bank has two objects, checking and savings of type Account. Implement four member functions:
deposit(double amount, string account) withdraw(double amount, string account) transfer(double amount, string account) print_balance() The account string is "S" or " C". For the deposit or withdrawal, it indicates which account is affected. For a transfer it indicates the account from which the money is taken; the money is automatically transferred to the other account.
Test your program by using the main function below.
#include "Bank.h" using namespace std;
int main() { Bank my_bank; cout << "Inital bank balances: ";
my_bank.print_balances(); /* set up empty accounts */
cout << "Adding some money to accounts: "; my_bank.deposit(1000, "S"); /* deposit $1000 to savings */ my_bank.deposit(2000, "C"); /* deposit $2000 to checking */ my_bank.print_balances();
cout << "Taking out $1500 from checking,and moving $200 from"; cout << " savings to checking. "; my_bank.withdraw(1500, "C"); /* withdraw $1500 from checking */ my_bank.transfer(200, "S"); /* transfer $200 from savings */ my_bank.print_balances();
cout << "Trying to transfer $900 from Checking. "; my_bank.transfer(900, "C"); my_bank.print_balances();
cout << "trying to transfer $900 from Savings. "; my_bank.transfer(900, "S"); my_bank.print_balances();
return 0;
}
Output
Initial bank balances: Checking account balance: 0 Savings account balance: 0 Adding some money to accounts: Checking account balance: 2000 Savings account balance: 1000 Taking out $1500 from checking,and moving $200 from savings to checking. Checking account balance: 700 Savings account balance: 800 Trying to transfer $900 from Checking. Checking account balance: 680 Savings account balance: 800 trying to transfer $900 from Savings. Checking account balance: 680 Savings account balance: 780
Partial code
#ifndef ACCOUNT_H #define ACCOUNT_H
class Account { private: double balance; public: Account(); Account(double amt); void deposit(double amt); void withdraw(double amt); double getBalance(); };
#endif
#include
#ifndef BANK_H #define BANK_H #include "Account.h" #include
class Bank { private: Account checking; Account savings; public: Bank(); void deposit(double amount, string account); void withdraw(double amount, string account); void transfer(double amount, string account); void print_balances(); };
#endif
#include
Bank::Bank() { checking = Account(); savings = Account(); }
void Bank::deposit(double amount, string account) { if (account == "C") { ________________________ } else if (account == ___________) { ____________________________ } }
void Bank::withdraw(double amount, string account) { ________________________________ }
void Bank::transfer(double amount, string account) { if (account == "C") { checking.withdraw(____________); _______________________________ } else if (account == ____________________) { _________________________________ } }
void Bank::print_balances() { cout << "Checking account balance: " << ________________ << endl; cout << "Savings account balance: " << ___________________________ << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
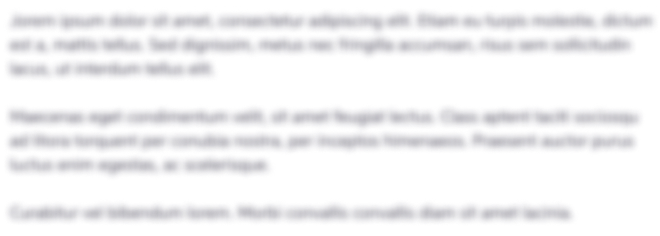
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started