Question
Implement a class 'KitKat' that represents a KitKat candy bar.. Each KitKat object will keep track of the flavor of the candy bar as well
Implement a class 'KitKat' that represents a KitKat candy bar.. Each KitKat object will keep track of the flavor of the candy bar as well as the number of fingers (sections) remaining in the bar. Implementation details:
creating and displaying objects
- the constructor ( __init__ ) accepts two optional arguments
- flavor, a str that defaults to 'Milk Chocolate'
- number of fingers remaining, an int that defaults to 4. (Assume that any argument given is non-negative, no data validation is required.)
2.__repr__ returns a str version of the KitKat as formatted below, note that single quotes appear around the brand
---------------------------------------------------------------------------------------------------------------
>>> candy = KitKat() # using both defaults
>>> candy
KitKat('Milk Chocolate', 4)
>>> candy = KitKat('Green Tea',3) # flavor and fingers both specified
>>> candy KitKat('Green Tea', 3)
>>> candy = KitKat('Mint') # flavor specified, fingers default to 4
>>> candy
KitKat('Mint', 4)
eating
The 'eat' method allows one to eat one or more fingers/sections of a KitKat . Details:
- accepts one optional argument, the number of fingers to eat
- defaults to 1 if no argument is supplied
- otherwise, you may assume this is a positive integer
2.reduces the current number of fingers by the number eaten
- Any attempt to eat more fingers than the KitKat currently has, will consume only those that are there. (i.e., fingers cannot go negative)
3.returns a list containing copies of the flavor, one per finger that was actually eaten.
---------------------------------------------------------------------------------------------------------------
>>> candy = KitKat('Green Tea',4)
>>> candy.eat() # defaults to 1
['Green Tea']
>>> candy
KitKat('Green Tea', 3)
>>> candy.eat(4) # try to eat 4, but only 3 there, so eat 3
['Green Tea', 'Green Tea', 'Green Tea']
>>> candy KitKat('Green Tea', 0)
>>> candy.eat() # nothing left to eat
[]
>>> candy
KitKat('Green Tea', 0)
comparisons
Two KitKat objects are compared by comparing the number of fingers available, for these comparisons the flavor is ignored. There are two comparisons supported:
- '==' - this method must be named __eq__ , it accepts two KitKat objects and returns True if and only if they currently have the same number of fingers.
- '>' - this method must be named __gt__ , it accepts two KitKat objects and returns True if the first KitKat has strictly more fingers than the second KitKat .
- You may not have written __gt__ before. Structurally the code is identical to __eq__ , the only difference is that the Boolean expression inside the method is different, as described above.
---------------------------------------------------------------------------------------------------------------
>>> KitKat('Green Tea', 3) == KitKat('Mint', 3)
True
>>> KitKat('Green Tea', 3) == KitKat('Mint', 2)
False >>> KitKat('Green Tea', 3) > KitKat('Mint', 3)
False
>>> KitKat('Green Tea', 3) > KitKat('Mint', 2)
True
>>> KitKat('Green Tea', 2) > KitKat('Mint', 4)
False
Step by Step Solution
There are 3 Steps involved in it
Step: 1
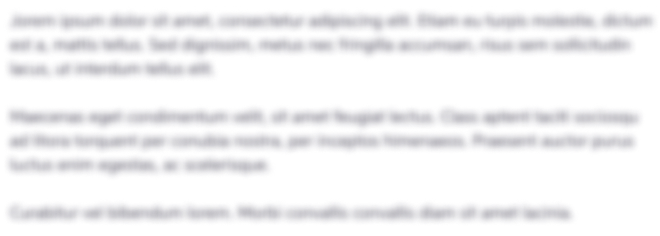
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started