Question
Implement a class Quiz that implements the Measurable interface. A quiz has a score and a letter grade (such as B+). Use the Data class
Implement a class Quiz that implements the Measurable interface. A quiz has a score and a letter grade (such as B+). Use the Data class of Exercise E10.1 to process an array of quizzes. Display the average score and the quiz with the highest score (both letter grade and score)--------- This is what my project is on. Right now most of it is done.
WHAT I NEED TO DO:
I need to get the average to work in the tester/runner class.
I cant figure out how to show the max with the correct grade letter
I am not allowed to use a rounder. I need to put .2%f in there somewhere I dont know where though
package measurable;
import java.text.DecimalFormat;
/*Implement a class Quiz that implements the Measurable interface. A quiz has a score
and a letter grade (such as B+). Use the Data class of Exercise E10.1 to process an array
of quizzes. Display the average score and the quiz with the highest score (both letter
grade and score).*/
public class Data
{
public static double average(Measurable[] objects)
{
double sum = 0;
for(Measurable obj : objects)
{
sum = sum + obj.getMeasure();
}
if(objects.length > 0)
{
return sum / objects.length;
}
else
{
return 0;
}
}
public static Measurable max(Measurable[] objects)
{
if(objects.length == 0)
{
return null;
}
else
{
Measurable max = objects [0];
for(Measurable score : objects)
{
if(max.getMeasure() > score.getMeasure())
{
score = max;
}
}
return max;
}
}
}
package measurable;
public class Quiz implements Measurable
{
private static String letterGrade;
private static double score;
public Quiz(double score, String letterGrade)
{
Quiz.score = score;
Quiz.letterGrade = letterGrade;
}
public double getMeasure()
{
return score;
}
public double getScore()
{
return score;
}
public String getLetterGrade()
{
return letterGrade;
}
public static String getLetterGrade(Measurable[] quizzes)
{
if(score >= 98)
{
letterGrade = "A+";
}
if(score >= 94 && score < 98)
{
letterGrade = "A";
}
if(score >= 90 && score < 94)
{
letterGrade = "A-";
}
if(score >= 88 && score < 90)
{
letterGrade = "B+";
}
if(score >= 84 && score < 88)
{
letterGrade = "B";
}
if(score >= 80 && score < 84)
{
letterGrade = "B-";
}
if(score >= 78 && score < 80)
{
letterGrade = "C+";
}
if(score >= 74 && score < 78)
{
letterGrade = "C";
}
if(score >= 70 && score < 74)
{
letterGrade = "C-";
}
if(score >= 68 && score < 70)
{
letterGrade = "D+";
}
if(score >= 64 && score < 68)
{
letterGrade = "D";
}
if(score >= 60 && score < 64)
{
letterGrade = "D-";
}
if(score < 60)
{
letterGrade = "F";
}
return letterGrade;
}
}
package measurable;
import java.text.*;
public class QuizRunner
{
public static void main(String args[])
{
//arraylist of quizzes with each having a score and letter grade
Measurable[] quizzes = new Measurable [6];
quizzes[0] = new Quiz(67, "D+");
quizzes[1] = new Quiz(75, "C");
quizzes[2] = new Quiz(80, "B-");
quizzes[3] = new Quiz(90, "A-");
quizzes[4] = new Quiz(95, "A");
quizzes[5] = new Quiz(72, "C-");
System.out.println("Average score is " + Data.average(quizzes) + " which is an average letter grade of a(n) " + Quiz.getLetterGrade(quizzes) + ".");
System.out.printf("The highest score is " + Data.max(quizzes) + " which is a(n) " + Quiz.getLetterGrade(quizzes) + ".");
//cast in notes for Data.max(quizzes)
}
}
package measurable;
public interface Measurable
{
double getMeasure();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
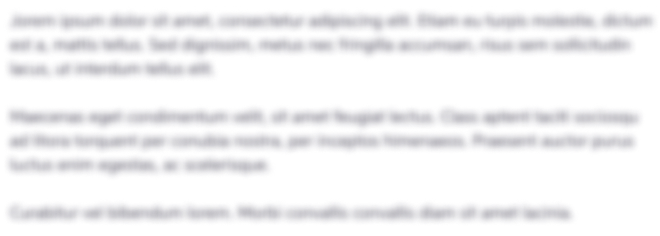
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started