Question
Implement a container class PriorityQueue (that is a subclass of the object class) supporting nine methods: __init__ which takes no parameter and initializes the object
Implement a container class PriorityQueue (that is a subclass of the object class) supporting nine methods:
__init__ which takes no parameter and initializes the object to an empty queue
insert which takes a number as a parameter and inserts the number into the queue, modifying the queue afterward to insure that the number is placed in the correct position
minimum which takes no parameters and returns the smallest number in the queue
removeMin which takes no parameters and removes the smallest number from the queue, returning the value removed
__len__ which takes no parameters and returns the size of the priority queue
__str__ which takes no parameters and returns a string representing the queue
__repr__ which takes no parameters and returns the canonical representation of the queue
__getitem__ which takes an index as a parameter and returns the queue item at that index without modifying the queue
__iter__ which implements iteration for the queue -- iteration should proceed from the smallest to the largest number in the queue
I suggest that you use a list to store the numbers in the priority queue. Your implementation must insure that the list is ordered after every operation (insertion or removal) so that the minimum number is stored in one end of the list. The following shows how the PriorityQueue class and its methods could be used. Note that in my implementation the queue is stored in non-decreasing order (i.e. with the minimum at the front of the queue). You can also write an implementation that stores the queue items in non-increasing order (i.e. with the minimum at the end of the queue). Either implementation is fine, although your choice will impact the way that you write the __getitem__ and __iter__ methods:
The version of the Person class developed for the second assignment allows the creation of people who have not yet been born since it does not have any error checking on the birth year used to create the object. For this question you will create a new exception class InvalidDate that will be raised in the constructor for the person class when a Person is created whose birth year is after the current year. Carefully examine the sample output below to ensure that you are providing the required information when raising the exception. Also note that this problem does not involve the use of any try-except blocks. The following shows the way that the new Person class and its methods would behave when given invalid parameters, with some parts of the output redacted to not give away the answer to the question:
>>> p1 = Person('Amber Settle', 1968) >>> p1 Person(Amber Settle, 1968) >>> print(p1) Amber Settle is 47 years old. >>> p2 = Person("Ohto Kurhila", 2017) Traceback (most recent call last): File "
For this question you will modify the processPeople() function written for the second assignment to handle the InvalidDate exceptions that the Person class now raises when given invalid dates. You should start from the function provided in the assignment template (which is a version written by Charley Grossman and modified by Amber Settle). The function should be modified so that when an InvalidDate exception occurs when creating an Person object the function skips that item in the file and prints a message indicating what happened. The message printed should be captured from the exception raised by the Person class. Solutions that recreate the information rather than using a proper except block to capture the information will not earn full credit. (Please ask if you don't know what I mean by this). Note that a correct solution to this function will contain no raise statements and no additional if-else statements. It should only include some additional try-except blocks.The following shows the function results on several functions provided in the assignment template zip file. The file file1.txt is the same as the one from the second assignment and contains no problematic years. The file file2.txt has some additional people in it, some of whom include invalid dates. The file file3.txt is empty. The file none.txt does not exist.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
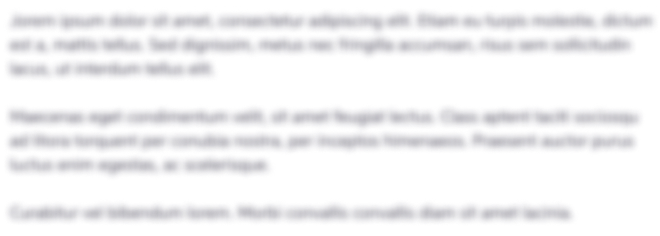
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started