Question
Implement a crazy calculator that supports integer operands like 1, 64738, and -42 as well as the (binary) integer operators +, -, * and /.
Implement a crazy calculator that supports integer operands like 1, 64738, and -42 as well as the (binary) integer operators +, -, * and /. Your program should be called CrazyCalc and work as follows:
You will need to create an empty stack using your BackwardStack class. This stack will hold integer values. At any time, the stack becomes full it must expand to make room for more integers. The program must repeatedly accept input from the user based off their selection from a menu (must be a separate method). The choices given by the menu are the four operations listed above along with # which indicates the user wants to enter a number, ? which indicates the user wants to see what is in the stack and finally an x or X which indicates the user is done.
If the user enters an integer, you push that integer onto the stack. If the user enters a valid operator, you pop two integers off the stack, perform the requested operation, and push the result back onto the stack. You will also need to print in standard format what calculation was done. i.e. 4 + 3 = 7
The user can continue entering input as long as they want and as stated before they will enter x or X when they are finished.
*** Must use switch statement to check what was selected in menu ***
*** If the want to do an operation but there are not two values in the stack must ask to enter another value (or two) in order to perform operation ***
BACKWARD STACK PROGRAM IS AS FOLLOWS
import java.util.*;
public class BackwardStack {
private int[] backStack = new int[20]; //initial size is 20 private int size = 0; public int theSize(){ return size; } public boolean isEmpty() { if(size == 0){ System.out.println("The stack is empty"); return true; } else if(size > 0) System.out.println("Stack is not empty"); return false; } public boolean thePush(int value) { if(size == backStack.length) {
makeBigger(); } for (int s= size; s>0; --s){ backStack[s] = backStack[s-1]; } backStack[0] = value; size++; System.out.println("the push is working"); return true; } /* public boolean thePush(Stack
makeBigger(); } for (int m= size; m>0; --m){ backStack[m] = backStack[m-1]; stack.push(backStack); } size++; return true; } */ public boolean thePop(){ if(size == -1){ return false; } else{ for(int m = size; m < size; ++m){ //backStack[m] = backStack[m+1]; } size--; return true; } } public String printStack(){ String stackOutput = (Arrays.toString(backStack)); return stackOutput; } /*public boolean printStack(int value){ int index = findSomething(value); if(index == -1){ return false; } else{ System.out.println(Arrays.toString(backStack)); } return true; } */ private boolean makeBigger() { int[] newBackStack = new int[(int)(size*1.5)]; for(int t=0; t private int findSomething(int value) { for(int z=size-1; z>=0; z--) { if(backStack[z] == value); return z; } return -1; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
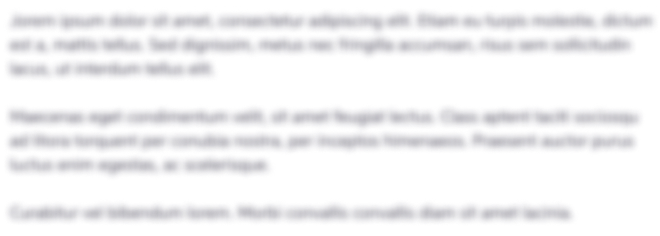
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started