Implement a Fraction class in Fraction.cpp with header Fraction.h including the following functions: Question 2.1: Constructor: Initialize a new Fraction. The format of the constructor
Implement a Fraction class in Fraction.cpp with header Fraction.h including the following functions:
Question 2.1: Constructor:
Initialize a new Fraction. The format of the constructor is Fraction(int numerator, int denominator).
Please remember to judge the legality. If its illegal (i.e. the denominator is 0), print Initialization Error
and assign a default value 0,1
Sample Run | Result |
//main1.cpp #include Fraction a(5, 0); std::cout << a << std::endl; return 0; } | Initialization Error 0 |
Question 2.2: Overload operator (<<): 1. Print the double / floating point value of (numerator / denominator)
Sample Run | Result |
//main2.cpp #include Fraction a(5, 0); std::cout << a << std::endl; Fraction b(1, 4); std::cout << b << std::endl; return 0; } | Initialization Error 0 0.25 |
Question 2.3: Overload operators (+, -) 1. Add an integer to or subtract an integer from a Fraction object.
Sample Run | Result |
//main3.cpp #include Fraction a(5, 1); std::cout << a << std::endl; a = a + 10; std::cout << a << std::endl; a = a - 3; std::cout << a << std::endl; return 0; } | 5 15 12 |
Question 2.4: Overload operators (<, > and ==)
Compare two Fraction objects.
We can assume that that the operators will apply on legal Fraction objects and the result of Fraction
objects are legal.
Sample Run | Result |
//main4.cpp #include Fraction a(10, 2); Fraction b(2, 1); if (a > b) { std::cout << "a is larger than b" << std::endl; } else if (a < b) { std::cout << "b is larger than a" << std::endl; } else { std::cout << "a is the same as b" << std::endl; } b = b + 100; | a is larger than b b is larger than a a is the same as b |
if (a > b) { std::cout << "a is larger than b" << std::endl; } else if (a < b) { std::cout << "b is larger than a" << std::endl; } else { std::cout << "a is the same as b" << std::endl; } a = a + 97; if (a > b) { std::cout << "a is larger than b" << std::endl; } else if (a < b) { std::cout << "b is larger than a" << std::endl; } else { std::cout << "a is the same as b" << std::endl; } return 0; } |
Question 2.5: Overload Increment and Decrement operators (++ and --):
Sample Run | Result |
//main5.cpp #include Fraction a(1, 3); std::cout << a << std::endl; std::cout << a++ << std::endl; std::cout << ++a << std::endl; std::cout << a << std::endl; std::cout << a-- << std::endl; std::cout << --a << std::endl; return 0; } | 0.333333 0.333333 2.33333 2.33333 2.33333 0.333333 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
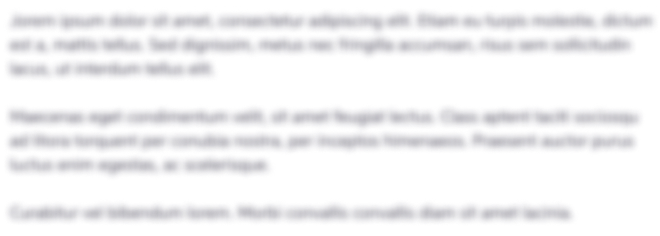
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started