Question
Implement a java class named Dog that contains information about a dog. The Dog class has the following instance variables (a.k.a. fields or data): The
Implement a java class named Dog that contains information about a dog. The Dog class has the following instance variables (a.k.a. fields or data):
The dog's name The breed The age of the dog
The Dog class will have methods to:
Create a new Dog (given a dog's name, breed and age) [constructor] Create a new Dog (from another Dog) [copy constructor - like cloning a dog] getName getBreed getAge setName setBreed setAge ageInHumanYears - calculates and returns the dog's age in human years, which is generally estimated as: (if the dog is <= 2 years old, multiply its age * 11, else, subtract the age - 2, multiply by 5 and add 22) equals - method to check if one Dog is the same as another by comparing all instance variables toString - method to turn a Dog into a string for display, e.g. display as "Dog [Name=Scooby, Breed=Great Dane, Age=7, Human Age=47]"
Once your Dog class is finished, please create a driver class named DogDemo with a main() method that does the following:
Creates a new Dog object named "scooby" with the following information: name = "Scooby" breed = "Great Dane" age = 7
Creates another Dog object named "myDog" by copying scooby.
Print both objects to the console.
Compare the two Dogs using the equals method. If the Dogs are equal, print the message "Both dogs are the same." Otherwise print the message "The dogs are different."
Now, change each of the fields for "myDog" with mutators (set...) to the following values (if you have a dog, feel free to use your pet's info below instead): name = "Toby" breed = "Terrier" age = 2
Print both objects to the console
Once more, compare the two Dogs using the equals method. If the Dogs are equal, print the message "Both dogs are the same." Otherwise print the message "The dogs are different."
Step by Step Solution
There are 3 Steps involved in it
Step: 1
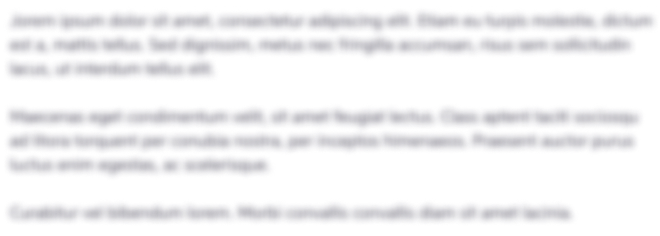
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started