Question
//Implement a Java Deque class using Generics and a circular array. //Need help on implementing on iterator import java.util.Deque; import java.util.NoSuchElementException; public class deque implements
//Implement a Java Deque class using Generics and a circular array. //Need help on implementing on iterator
import java.util.Deque; import java.util.NoSuchElementException;
public class deque
private Node
private Node
private int size;
public deque() {
head = null;
tail = null;
size = 0;
}
@Override // void addFirst(E e)-Inserts the specified element at the front of this deque. public void addFirst(E element) { Node
if (head == null) {
head = node;
tail = node;
} else {
node.setNext(head);
head.setPrev(node);
head = node;
}
size++;
}
@Override //void addLast(E e)-Inserts the specified element at the end of this deque. public void addLast(E element) {
Node
if (tail == null) {
head = node;
tail = node;
} else {
tail.setNext(node);
node.setPrev(tail);
tail = node;
}
size++;
}
@Override //E removeFirst()-Retrieves and removes the first element of this deque. public E removeFirst() { if (head == null) {
throw new NoSuchElementException("Deque is empty!");
}
Item item = head.getItem();
head = head.getNext();
if (head == null) {
tail = null;
} else {
head.setPrev(null);
}
size--;
return item; }
@Override //E removeLast()-Retrieves and removes the last element of this deque. public E removeLast() { if (tail == null) {
throw new NoSuchElementException("Deque is empty!");
}
Item item = tail.getItem();
tail = tail.getPrev();
if (tail == null) {
head = null;
} else {
tail.setNext(null);
}
size--;
return item;
}
//int size()-Returns the number of elements in this deque. public int size() { return size;
}
//Iterator
public static void main(String[] args) {
deque
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
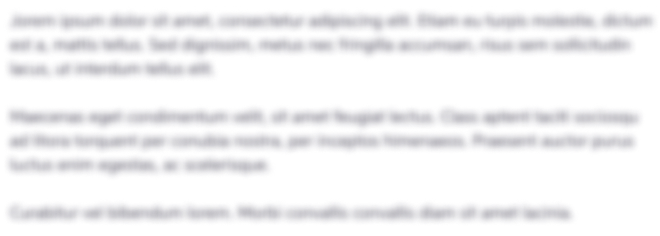
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started