Question
implement a looking-for-group queue for a game where players can be one of three roles (Defender, Hunter, and Bard) and each group consists of one
implement a looking-for-group queue for a game where players can be one of three roles (Defender, Hunter, and Bard) and each group consists of one Defender, one Hunter, and one Bard.
Create a new C++ source file named lfgqueue.cpp that implements the LFGQueue class declared in lfgqueue.h such that lfqueue.cpp and the provided files compile into a program that runs with no failed tests.
please use the code given below
/////////////////////////////////////////
(lfgqueue.h)
#ifndef LFGQUEUE_H #define LFGQUEUE_H #include "player.h" class LFGQueue { public: // Constructs a new empty queue LFGQueue(); // Returns the number of players in the queue. int size(); // Pushes a pointer to a player onto the back of the queue. void push_player(Player* p); // Returns a pointer to the frontmost player // with the specified role. // If no such player exists, returns nullptr. Player* front_player(Player::Role r); // Removes the frontmost player with the specified role. // If no such player exists, does nothing. void pop_player(Player::Role r); // Returns whether the queue contains a complete group // (a Defender, a Hunter, and a Bard). // // If the queue contains a complete group, the method // sets the first three elements of the array parameter // equal to the addresses of the frontmost: // 1. Defender (index 0) // 2. Hunter (index 1) // 3. Bard (index 2) bool front_group(Player** group); // Removes the frontmost Defender, Hunter, and Bard // from the queue. If some role has no player with that role, // then does nothing. void pop_group(); private: Player** players; int count; int capacity; }; #endif
////////////////////////////////////////////// (player.h)
#ifndef PLAYER_H #define PLAYER_H #include
/////////////////////////////////////////////////// (player.cpp)
#include "player.h" Player :: Player(string name, Role role) { _name = name; _role = role; } string Player :: name() { return _name; } Player::Role Player :: role() { return _role; }
/////////////////////////////////////////
(main.cpp)
#include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
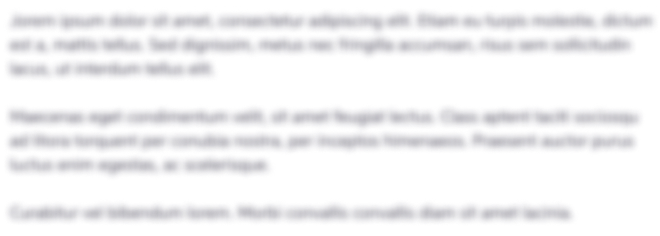
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started