Question
Implement a method to convert a tree to a string. Your HW8Tree class must include the BinaryNode.java code. Your HW8Tree class must have an instance
Implement a method to convert a tree to a string.
Your HW8Tree
Your HW8Tree
a public boolean add(E item, boolean[] left) method to add values to the tree. Starting from the root, at each node, the method must go down to the left if the corresponding position in the array is true, and otherwise must go down to the right. Once a null pointer is reached, the item must be added to the tree in place of the null pointer.
It is an error if the array of booleans is too short. In this case, the add method should throw a RuntimeException.
a public List
a public String toString() method that converts the tree to a string, in the same way that folders are printed: the root is first, followed by the children of the node indented 4 spaces, the grandchildren indented 8 spaces, and so on.
Each of these methods must use recursion to traverse the tree. In each case, the recursion may be in a helper method.
For example, consider a tree with root "hello". "hello" has two children, "foo" and "bar". "foo" has children "world", and "baz", and "bar" has children "bug" and "loop".
The toString method converts the tree to the following string:
hello foo world baz bar bug loop
The above example shows what the string looks like when it is printed. As a string, it actually looks like this:
"hello foo world baz bar bug loop "
As many of you already know, " " is the newline character in Java.
Calling toList should return a list with contents world, foo, baz, hello, bug, bar, loop. Only this order correctly reflects an in-order traversal.
To add the string "fum" below and to the right of "bug", we would call
boolean[] lefts = { false, true, false}; HW8Tree myTree = new HW8Tree (); // fill in the tree until it matches the example above, then myTree.add ("fum", lefts);
__________________________________________________________________________________________________
==============================BinaryNode.java=================================
private static class BinaryNode{ private T item; private BinaryNode left; private BinaryNode right; /** * constructor to build a node with no subtrees * @param the value to be stored by this node */ private BinaryNode(T value) { item = value; left = null; right = null; } /** * constructor to build a node with a specified (perhaps null) subtrees * @param the value to be stored by this node * @param the left subtree for this node * @param the right subtree for this node */ private BinaryNode(T value, BinaryNode l, BinaryNode r) { item = value; left = l; right = r; } }
Not sure if this will help, but what I have previously:
import lab.solution.BinaryTree;
===============================Main.java===============================
public class Main {
/** * Write the function in the BinaryTree class to determine whether or not a given binary tree is a max or min heap */ public static void main(String args[]) { //True BinaryTree bt = new BinaryTree(); bt.add(50); bt.add(20); bt.add(25); bt.add(13); bt.add(12); bt.add(15); bt.add(2); System.out.println(bt.isMaxHeap());
//False BinaryTree bt1 = new BinaryTree(); bt1.add(50); bt1.add(20); bt1.add(25); bt1.add(26); bt1.add(12); bt1.add(15); bt1.add(2); System.out.println(bt1.isMaxHeap());
//False BinaryTree bt2 = new BinaryTree(); bt2.add(50); bt2.add(20); bt2.add(25); bt2.add(13); bt2.add(12); bt2.add(15); bt2.add(51); System.out.println(bt2.isMaxHeap());
//False BinaryTree bt3 = new BinaryTree(); bt3.add(50); bt3.add(20); bt3.add(25); bt3.add(31); bt3.add(12); bt3.add(15); bt3.add(2); System.out.println(bt3.isMaxHeap());
//What do you think this should return?? :) //What if we made equal cases not allowed? (IE. A parent node 30 with child node 30 is wrong for a max heap) BinaryTree bt4 = new BinaryTree(); bt4.add(50); bt4.add(20); bt4.add(25); //This parent bt4.add(13); bt4.add(12); bt4.add(15); bt4.add(25); //Has this child System.out.println(bt4.isMaxHeap()); //Try writing a set of test cases for minHeap tests BinaryTree bt5 = new BinaryTree(); bt5.add(2); bt5.add(11); bt5.add(13); bt5.add(15); bt5.add(25); bt5.add(31); bt5.add(50); System.out.println(bt5.isMinHeap()); }
}
==================================BinaryTree.java===============================
import java.util.LinkedList; import java.util.Queue;
public class BinaryTree { private Node
/** * Implement this function. * @return true if if our tree is a Max Heap, false otherwise. */ public boolean isMaxHeap() { Node
}
==============================Node.java==============================
public class Node
private Node
________________________________________________________________________________________________
RuntimeException: (http://docs.oracle.com/javase/8/docs/api/java/lang/RuntimeException.html)
List interface: (http://docs.oracle.com/javase/8/docs/api/java/util/List.html)
Please briefly comment what parts of the code does, Thanks.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
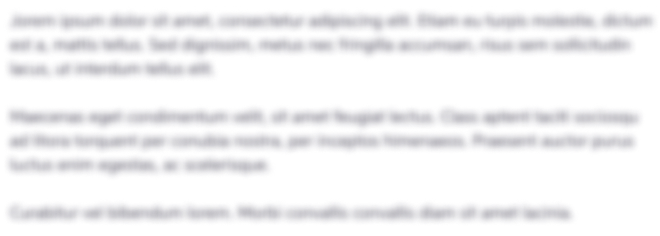
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started