Question
Implement a MIPS assembly language program that defines main, readArray and removeEvenOdd procedures. The readArray takes an array of integers as its parameter, reads in
Implement a MIPS assembly language program that defines main, readArray and removeEvenOdd procedures. The readArray takes an array of integers as its parameter, reads in integers from a user to fill the array and also print each value as long as it is with the number of elements specified by the parameter "howMany" and "length". The removeEvenOdd procedure takes parameters of an array of integers, its length, and even value, an asks a user how many integers to read in and calls the readyArray procedure. Then it should go through the array to see if each number is even (divisible by 2) if the parameter even is 0. In that case, such even integer in the array is changed to 0. If the parameter even is 1, then it changes every odd integer in the array to 0. Then it prints out the updated content of the array. The main procedure asks a user to specify whether to remove even or odd numbers by asking them to enter an integer. Then it calls the removeEvenOdd procedure. It repeats these two steps twice. Please see the following C program to understand how it should work. If your program causes an infinite loop, press Control and 'C' keys at the same time to stop it. Name your source code file assignment6.s.
The following shows how it looks like in a C program:
//The readArray procedure reads integers from user input //and prints each element in the array void readArray(int array[], int length, int howMany) { int num, i = 0; printf("The array content: "); while (i < howMany && i < length) { printf("Enter an integer: "); //read an integer from a user input and store it in num1 scanf("%d", &num); array[i] = num; //print numbers printf("%d ", array[i]); i++; } return; } //The removeEvenOdd sets each even integer in the array to 1, //if the parameter "even" is 0, //and sets each odd integer in the array to 0, //if the parameter "even" is not 0. void removeEvenOdd(int array[], int length, int even) { int i = 0; int howMany = 0; printf("Specify how many numbers should be stored in the array (at most 8): "); scanf("%d", &howMany); readArray(array, length, howMany); while (i < howMany && i < length) { if (even == 0 && array[i]%2 == 0) { array[i] = 1; } else if (even != 0 && array[i]%2 != 0) { array[i] = 0; } i++; } if (even == 0) printf("After removing even integers "); else printf("After removing odd integers "); i = 0; while (i < howMany && i < length) { printf("%d ", array[i]); i++; } } // The main calls the removeEvenOdd // procedure twice with different arrays void main() { int arraysize = 8; int numbers[arraysize]; int i, even; printf("Enter zero to remove even numbers or non-zero to remove odd numbers "); scanf("%d", &even); removeEvenOdd(numbers, arraysize, even); printf("Enter zero to remove even numbers or non-zero to remove odd numbers "); scanf("%d", &even); removeEvenOdd(numbers, arraysize, even); return; }
The following is a sample output (user input is in bold):
Enter zero to remove even numbers or non-zero to remove odd numbers 0 Specify how many numbers should be stored in the array (at most 8): 8 Enter an integer: 1 Enter an integer: -12 Enter an integer: 53 Enter an integer: -4 Enter an integer: 5 Enter an integer: 32 Enter an integer: 1 Enter an integer: 7 The array content: 1 -12 53 -4 5 32 1 7 After removing even integers 1 1 53 1 5 1 1 7 Enter zero to remove even numbers or non-zero to remove odd numbers 1 Specify how many numbers should be stored in the array (at most 8): 5 Enter an integer: 1 Enter an integer: -5 Enter an integer: -2 Enter an integer: 3 Enter an integer: 2 The array content: 1 -5 -2 3 2 After removing odd integers 0 0 -2 0 2
--------------------------------------------------
What to turn in:
programs should be well commented.
Each procedure needs to have a header using the following format:
############################################################################ # Procedure readArray # Description: ----- # parameters: $a0 = address of array # return value: $v0 = length # registers to be used: $s3 and $s4 will be used. ############################################################################
Step by Step Solution
There are 3 Steps involved in it
Step: 1
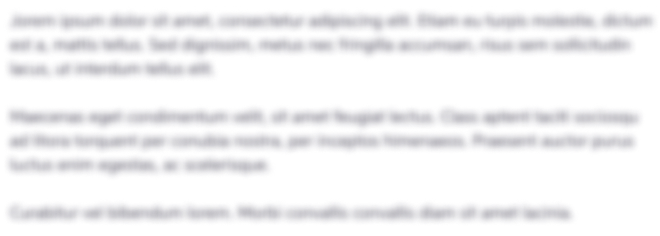
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started