Question
Implement a simple calculator with basic four operations: +, -, *, /. It should run as follows: Input: 1 + 2 Result: 3 Write the
Implement a simple calculator with basic four operations: +, -, *, /. It should run as follows:
Input: 1 + 2
Result: 3
Write the program with an exception handler that deals with illegal operations. Your program should display a message that informs the user of the error before exiting.
For example: Input: 1 / 0
Result: Division by zero is illegal I
nput: A + 4
Result: Invalid operand type
this is what I have so far but its not working
import java.util.*;
public class Calculator {
public static void main (String[] args) throws NumberFormatException{
@SuppressWarnings("resource")
Scanner keyboard = new Scanner(System.in);
do {
double result = 0;
int operand1 = 0;
int operand2 = 0;
String operator = "";
char op = ' ';
try{
System.out.print("Input first number: ");
operand1 =keyboard.nextInt();
System.out.print("Enter the operator ( + , - , * , / ): ");
operator = keyboard.next();
op = operator.charAt(0);
System.out.print("Input second number: ");
operand2 = keyboard.nextInt();
switch (op)
{
case '+':result = operand1 + operand2;
System.out.println("Answer: " + operand1 + ' ' + op + ' ' + operand2 + " = " + result );
break;
case '-': result = operand1 - operand2;
System.out.println("Answer: " + operand1 + ' ' + op + ' ' + operand2 + " = " + result );
break;
case '*': result = operand1 * operand2;
System.out.println("Answer: " + operand1 + ' ' + op + ' ' + operand2 + " = " + result );
break;
if(operand2==0)
{
throw new IllegalArgumentException();
}
case '/': result = operand1/(double)operand2;
System.out.println("Answer: " + operand1 + ' ' + op + ' ' + operand2 + " = " + result );
break;
default:
System.out.println("Invalid operator");
break;
}
}
catch(IllegalArgumentException e){
System.out.println("Cannot divide by zero ");
}
catch(InputMismatchException ex){
System.out.println("The input is not an integer.");
System.out.println("Please reenter everything ");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
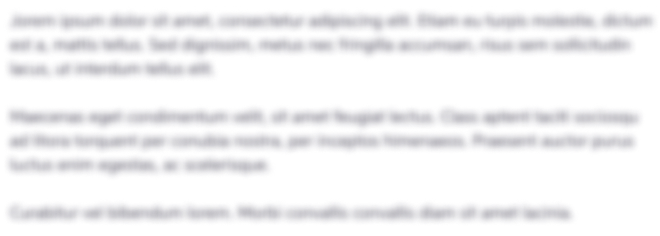
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started