Question
Implement class Vector that provides the vector functionality needed by the test code Vector_test.cpp given below. When you do this, you should not use C++
Implement class Vector that provides the vector functionality needed by the test code Vector_test.cpp given below. When you do this, you should not use C++ vector class.
Since Vector is a template container class, it should be implemented in one file: Vector.h. Complete Vector.h given below.
A few points regarding capacity versus size:
capacity: amount of memory allocated to the container in terms of number of elements. Number of bytes = capacity * length of T
size: number of elements in the container.
capacity is always >= size
capacity - size = number of elements that can be added without allocating more memory.
reserve(): increases capacity by allocating more memory.
resize(): could increase or decrease size. When increasing size, if necessary (when size > capacity) increase capacity to match size.
In your implementation when size = capacity and push_back() is called, allocate 5 more memory locations first (increase capacity by 5), then insert the new element (increase size by 1).
Take a look at capsize.cpp. Compile and run it and make sure you understand what it is doing.
#ifndef VECTOR_H #define VECTOR_H // Vector.h using namespace std; templateclass Vector { public: typedef T * iterator; Vector(); Vector(unsigned int size); Vector(unsigned int size, const T & initial); Vector(const Vector & v); // copy constructor ~Vector(); unsigned int capacity() const; // return capacity of vector (in elements) unsigned int size() const; // return the number of elements in the vector bool empty() const; iterator begin(); // return an iterator pointing to the first element iterator end(); // return an iterator pointing to one past the last element T & front(); // return a reference to the first element T & back(); // return a reference to the last element void push_back(const T & value); // add a new element void pop_back(); // remove the last element void reserve(unsigned int capacity); // adjust capacity void resize(unsigned int size); // adjust size T & operator[](unsigned int index); // return reference to numbered element Vector operator=(const Vector &); private: unsigned int my_size; unsigned int my_capacity; T * buffer; }; // Two sample methods follow template Vector ::Vector() { my_size = 0; my_capacity = 0; buffer = 0; } template // This line doesn't compile: Vector ::iterator Vector ::begin() // This line compiles: T * Vector ::begin() // But the follwoing line is the better solution: typename Vector ::iterator Vector ::begin() { return buffer; } // Rest of your code goes here ... #endif
#include#include #include #include "Vector.h" using namespace std; int main() { Vector v; v.reserve(2); assert(v.capacity() == 2); Vector v1(2); assert(v1.capacity() == 2); assert(v1.size() == 2); assert(v1[0] == ""); assert(v1[1] == ""); v1[0] = "hi"; assert(v1[0] == "hi"); Vector v2(2, 7); assert(v2[1] == 7); Vector v10(v2); assert(v10[1] == 7); Vector v3(2, "hello"); assert(v3.size() == 2); assert(v3.capacity() == 2); assert(v3[0] == "hello"); assert(v3[1] == "hello"); v3.resize(1); assert(v3.size() == 1); assert(v3[0] == "hello"); Vector v4 = v3; assert(v4.size() == 1); assert(v4[0] == v3[0]); v3[0] = "test"; assert(v4[0] != v3[0]); // fails when assignment results in shallow copy assert(v4[0] == "hello"); v3.pop_back(); assert(v3.size() == 0); Vector v5(7, 9); Vector ::iterator it = v5.begin(); while (it != v5.end()) { assert(*it == 9); ++it; } Vector v6; v6.push_back(100); assert(v6.size() == 1); assert(v6[0] == 100); v6.push_back(101); assert(v6.size() == 2); assert(v6[0] == 100); v6.push_back(101); cout << "SUCCESS "; }
so far i have done the skeleton but need help filling in some classes
#ifndef VECTOR_H #define VECTOR_H #include "Vector.h"
// Vector.h
using namespace std;
template
typedef T * iterator;
Vector(); Vector(unsigned int size); Vector(unsigned int size, const T & initial); Vector(const Vector
unsigned int capacity() const; // return capacity of vector (in elements) unsigned int size() const; // return the number of elements in the vector bool empty() const;
iterator begin(); // return an iterator pointing to the first element iterator end(); // return an iterator pointing to one past the last element T & front(); // return a reference to the first element T & back(); // return a reference to the last element void push_back(const T & value); // add a new element void pop_back(); // remove the last element
unsigned int size() const; // return the number of elements in the vector bool empty() const;
iterator begin(); // return an iterator pointing to the first element iterator end(); // return an iterator pointing to one past the last element T & front(); // return a reference to the first element T & back(); // return a reference to the last element void push_back(const T & value); // add a new element void pop_back(); // remove the last element
void reserve(unsigned int capacity); // adjust capacity void resize(unsigned int size); // adjust size
T & operator[](unsigned int index); // return reference to numbered element Vector
private: unsigned int my_size; unsigned int my_capacity; T * buffer; };
template
template
template
template
template
template
template
template
template
template
template
template
template
template
template
template
template
template
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
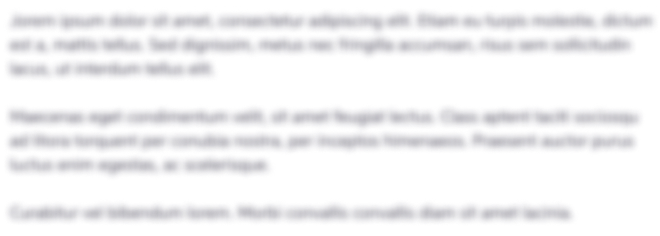
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started