Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Implement Graph algorithm based on the starter code provided and test your algorithms on multiple graphs. You must implement all the public functions in the
Implement Graph algorithm based on the starter code provided and test your algorithms on multiple graphs.
You must implement all the public functions in the starter code. You can and should write private functions to support the public functions. Some of the critical functions are:
Constructors: Graph
Destructor: ~Graph
Helpers: contains, verticesSize, edgesSize, vertexDegree, getEdgesAsString
Modifiers: add, connect, disconnect, readFile
Algorithms: dfs bfs dijkstra, mstPrim
#ifndef GRAPHH
#define GRAPHH
#include
#include
#include
#include
using namespace std;
class Graph
private:
public:
constructor, empty graph
explicit Graphbool directionalEdges true;
copy not allowed
Graphconst Graph &other delete;
move not allowed
GraphGraph &&other delete;
assignment not allowed
Graph &operatorconst Graph &other delete;
move assignment not allowed
Graph &operatorGraph &&other delete;
destructor, delete all vertices and edges
~Graph;
@return true if vertex added, false if it already is in the graph
bool addconst string &label;
@return true if vertex is in the graph
bool containsconst string &label const;
@return total number of vertices
int verticesSize const;
Add an edge between two vertices, create new vertices if necessary
A vertex cannot connect to itself, cannot have PP
For digraphs directed graphs only one directed edge allowed, PQ
Undirected graphs must have PQ and QP with same weight
@return true if successfully connected
bool connectconst string &from, const string &to int weight ;
Remove edge from graph
@return true if edge successfully deleted
bool disconnectconst string &from, const string &to;
@return total number of edges
int edgesSize const;
@return number of edges from given vertex, if vertex not found
int vertexDegreeconst string &label const;
@return string representing edges and weights, if vertex not found
AB AC should return BC
string getEdgesAsStringconst string &label const;
Read edges from file
first line of file is an integer, indicating number of edges
each line represents an edge in the form of "string string int"
vertex labels cannot contain spaces
@return true if file successfully read
bool readFileconst string &filename;
depthfirst traversal starting from given startLabel
void dfsconst string &startLabel, void visitconst string &label;
breadthfirst traversal starting from startLabel
call the function visit on each vertex label
void bfsconst string &startLabel, void visitconst string &label;
dijkstra's algorithm to find shortest distance to all other vertices
and the path to all other vertices
Path cost is recorded in the map passed in eg weightF
How to get to the vertex is recorded previousFC
@return a pair made up of two map objects, Weights and Previous
pair, map
dijkstraconst string &startLabel const;
minimum spanning tree using Prim's algorithm
ONLY works for NONDIRECTED graphs
ASSUMES the edge PQ has the same weight as QP
@return length of the minimum spanning tree or if start vertex not
int mstPrimconst string &startLabel,
void visitconst string &from, const string &to
int weight const;
minimum spanning tree using Kruskal's algorithm
ONLY works for NONDIRECTED graphs
ASSUMES the edge PQ has the same weight as QP
@return length of the minimum spanning tree or if start vertex not
int mstKruskalvoid visitconst string &from, const string &to
int weight const;
;
#endif GRAPHH
Step by Step Solution
There are 3 Steps involved in it
Step: 1
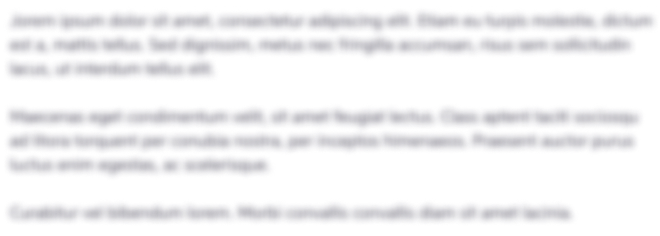
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started