Question
implement in java programing. this is banking system usecase . you have to codding in MVC(model,view and control) style entityclass: Account,savingaccount,checking account,Transaction Boundary class:- loginBo,SignUPBo,OpenBankAccountBO,TransferBO
implement in java programing. this is banking system usecase . you have to codding in MVC(model,view and control) style
entityclass: Account,savingaccount,checking account,Transaction
Boundary class:- loginBo,SignUPBo,OpenBankAccountBO,TransferBO
Control class:LoginControl,SignUpControl,OpenBankAccountcontrool,TransferControl.
Project #1
Use Case Name Login
Participating Actor Initiated by a customer
Flow of Events 1. A customer enters a username, password and clicks on Login contained in LoginBO.
2. LoginControl is created by LoginBO.
3. The control object creates Account object and invokes an operation of Account to verify the correctness of the username and password.
4. LoginControl creates OpenBankAccountBO and shows it if login is successful; otherwise, it shows Login failed because of invalid username or password.
Entry Conditions Login window is shown to the user.
Exit Conditions Login is confirmed of success or failure.
Quality Requirements The confirmation must be received in 0.1 seconds.
Develop your signIn() method in class Account to implement the use case. It is a continuation
from the given examples of signing up. OpenBankAccountBO.java is given.
Project #2
Use Case Name OpenBankAccount
Participating Actor Initiated by a customer
Flow of Events 1. A customer chooses Checking or Savings to open, verifies username and name, enters an account number and opening deposit amount, and clicks on Open contained in OpenBankAccountBO.
2. OpenBankAccountControl is created by OpenBankAccountBO.
3. The control object creates CheckingAccount(or SavingsAccount)object and invokes an operation of CheckingAccount(or SavingsAccount) to open the CheckingAccount(or SavingsAccount). If opening is successful, the control object creates Transaction object and invokes operation of recording this first deposit as a transaction.
4. OpenBankAccountControl uses JOptionPane.showMessageDialog() to show Account opening is successful. if the information provided is correct; otherwise, to show Opening failed because of invalid Account Number.
Entry Conditions Opening account window is shown to the user.
Exit Conditions Opening is confirmed of success or failure.
Quality Requirements Account Numbers length is exactly 8 digits; Confirmation must be received in 0.1 seconds.
Based on the use case above, do the follows so that a customer can open a checking account and/or a savings account.
Create tables CheckingAccount(CheckingAccountNumber, CustomerName, Balance, CustomerID), SavingsAccount(SavingsAccountNumber, CustomerName, Balance, InterestRate, CustomerID), Transactions(TransactionNumber, TransactionAmount, TransactionType, TransactionTime, TransactionDate, FromAccount, ToAccount, CustomerID). Use varchar(50) for any non-numerical value including AccountNumber and TransactionNumber, and float for any numerical value. CustomerID here means Username.
Study entity class CheckingAccount.java, and then develop your entity classes SavingsAccount.java and Transaction.java that has a method for recording a transaction.
Add necessary statements to OpenBankAccountControl.java to open both Checking and Savings accounts, and record the transactions of opening deposits.
Note that the provided OpenBankAccountControl.java can only open a Checking account if user inputs are correct and does not record transaction.
Project #3
Use Case Name Transfer
Participating Actor Initiated by a customer
Flow of Events 1. The customer selects either Checking Account or Savings Account as source, and Savings Account as destination if selected source is Checking Account(or Checking Account as destination if selected source is Savings Account.), and enters amount of money, and then clicks on Transfer contained in TransferBO.
2. TransferControl is created by TransferBO.
3. The control object creates CheckingAccount (or the selected source) object and invokes an operation of withdraw, and SavingsAccount (or selected destination) object and invokes an operation of deposit. If the transfer is successful, it creates Transaction object to keep track of the transfer.
4. TransferControl creates TransactionConfirmationBO to show Transfer Completed! if the transfer is successful; otherwise to show Transfer Failed!
Entry Conditions The customer has logged in to the system.
Exit Conditions Transfer is confirmed of success or failure.
Quality Requirements The transfer amount must be less than or equal to the current balance of the selected source; The selected source must be different from the selected destination.
Above is the use case of transferring money. Similarly develop your use cases of Account Overview, Inquire Transactions, Deposit, and Withdraw by using the services from your bank as examples. And develop your Java programs to fully implement the functionalities with access to database. Assume that a customer has two accounts, Savings Account and Checking Account. Account Overview shows the current balances of customers Savings Account and Checking Account. Inquire Transactions allow customers to search specific transactions by entering a starting date and ending date.
Develop methods deposit(), withdraw() in your classes CheckingAccount and SavingsAccount, getBalance() and calculateInterests() in class SavingsAccount, searchTransaction() in class Transactions. Then implement Transfer, Deposit, and Withdraw functionalities. It means that your program shows Transfer, Deposit, or Withdraw after successful login. Your current program is to show Open Bank Account window after successful login. Modifications are necessary. Demo your implementation in our class on Thursday, 10/18/2018.
Organize these functionalities as tabs in one window. The default tab is Account Overview. Please combine Open Account into this application. Show the window after successful login. These tabs must be in your window, Account Overview, Open Account, Deposit, Withdraw, Transfer and Inquire Transactions subtabs. Demo your program in our class on Thursday, October 25, 2018, and your .java files and your use cases.
Account.java
package Com.Patel;
import java.lang.*; //including Java packages used by this program import java.sql.*; import Com.Patel.*;
public class Account { private String Username, Password, Password1, Name;
public Account(String UN, String PassW, String PassW1, String NM) { Username = UN; Password = PassW; Password1 = PassW1; Name = NM; }
public Account(String UN, String PassW) { Username = UN; Password = PassW; }
public boolean signUp() { boolean done = !Username.equals("") && !Password.equals("") && !Password1.equals("") && Password.equals(Password1); try { if (done) { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Username FROM Account WHERE Username ='"+Username+"'"; //SQL query command ResultSet Rslt = Stmt.executeQuery(SQL_Command); //Inquire if the username exsits. done = done && !Rslt.next(); if (done) { SQL_Command = "INSERT INTO Account(Username, Password, Name) VALUES ('"+Username+ "','"+Password+"','"+Name+"')"; //Save the username, password and Name Stmt.executeUpdate(SQL_Command); } Stmt.close(); ToDB.closeConn(); } } catch(java.sql.SQLException e) { done = false; System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { done = false; System.out.println("Exception: " + e); e.printStackTrace (); } return done; }
public boolean changePassword(String NewPassword) { //5 boolean done = false; try { //20 DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT * FROM Account WHERE Username ='"+Username+ "'AND Password ='"+Password+"'"; //SQL query command ResultSet Rslt = Stmt.executeQuery(SQL_Command); //Inquire if the username exsits. if (Rslt.next()) { SQL_Command = "UPDATE Account SET Password='"+NewPassword+"' WHERE Username ='"+Username+"'"; //Save the username, password and Name Stmt.executeUpdate(SQL_Command); Stmt.close(); ToDB.closeConn(); done=true; } } catch(java.sql.SQLException e) //5 { done = false; System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { done = false; System.out.println("Exception: " + e); e.printStackTrace (); } return done; }
public String signIn() { String Name= ""; try {
DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); // create statement object String SQL_Command = "SELECT Name FROM Account WHERE Username ='"+Username+ "'AND Password ='"+Password+"'"; //SQL query command ResultSet Rslt = Stmt.executeQuery(SQL_Command); //Inquire if the username and password exsits. if(Rslt.next()) { Name=Rslt.getString(1); Stmt.close(); ToDB.closeConn(); } } catch (java.sql.SQLException e) {
System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) {
System.out.println("Exception: " + e); e.printStackTrace ();
}
return Name; } }
DBConnection.java
package Com.Patel;
import java.util.*; import java.sql.*;
public class DBConnection {
private Connection connection; private String URL;
public DBConnection() { //URL = "jdbc:odbc:JavaClass"; URL ="jdbc:sqlserver://127.0.0.1:1433;databaseName=JavaClass;integratedSecurity=true;";//"user=tang;password=; connection = null; } public Connection openConn() { try { //Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver"); connection = DriverManager.getConnection(URL); //connection = DriverManager.getConnection(URL, "tang", "xxxxxx"); } catch ( Exception e ) { e.printStackTrace(); connection = null; } return connection; }
public void closeConn() { try { connection.close(); } catch( Exception e ) { System.err.println ("Can't close the database connection."); } }
public Vector getNextRow(ResultSet rs,ResultSetMetaData rsmd) throws SQLException { Vector currentRow = new Vector();
for(int i=1;i
return currentRow; } }
CheckingAccount.java
package Com.Patel;
import java.lang.*; //including Java packages used by this program import java.sql.*; import Com.Patel.*;
public class CheckingAccount { //Instance Variables private String CheckingAccountNumber, CustomerName, CustomerID; private float Balance = -1;
public CheckingAccount(String CA_Num, String Cust_Name, String Cust_ID, float Bal) { //Constructor One with three parameters CheckingAccountNumber = CA_Num; CustomerName = Cust_Name; CustomerID = Cust_ID; Balance = Bal; //Balance = Float.parseFloat(Bal); }
//public CheckingAccount(String CA_Num) { //Constructor Two with one parameter // CheckingAccountNumber = CA_Num; // } public CheckingAccount(String Cust_ID) { CustomerID=Cust_ID; } /* method */ public boolean openAcct() { boolean done = false; try { if (!done) { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT CheckingAccountNumber FROM CheckingAccount WHERE CheckingAccountNumber ='"+CheckingAccountNumber+"'"; //SQL query command ResultSet Rslt = Stmt.executeQuery(SQL_Command); //Inquire if the username exsits. done = !Rslt.next(); if (done) { SQL_Command = "INSERT INTO CheckingAccount(CheckingAccountNumber, CustomerName, Balance, CustomerID)"+ " VALUES ('"+CheckingAccountNumber+"','"+CustomerName+"',"+Balance+", '"+CustomerID+"')"; //Save the username, password and Name Stmt.executeUpdate(SQL_Command); } Stmt.close(); ToDB.closeConn(); } } catch(java.sql.SQLException e) { done = false; System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { done = false; System.out.println("Exception: " + e); e.printStackTrace (); } return done; } public float getBalance() { //Method to return a CheckingAccount balance try { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM CheckingAccount WHERE CheckingAccountNumber ='"+CheckingAccountNumber+"'"; //SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command);
while (Rslt.next()) { Balance = Rslt.getFloat(1); //System.out.println("Balance:"+Balance); } Stmt.close(); ToDB.closeConn(); } catch(java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace (); } return Balance; }
public float getBalance(String ChkAcctNumber) { //Method to return a CheckingAccount balance try { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM CheckingAccount WHERE CheckingAccountNumber ='"+ChkAcctNumber+"'"; //SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command);
while (Rslt.next()) { Balance = Rslt.getFloat(1); } Stmt.close(); ToDB.closeConn(); } catch(java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace (); } return Balance; } public boolean Withdraw(String ChkAcctNumber, float amount) { boolean done = !ChkAcctNumber.equals(""); float balance = (float)getBalance(ChkAcctNumber); System.out.println(balance >= amount); if ((balance - amount)
} Stmt.close(); ToDB.closeConn(); } } catch (java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace(); } return done; }
public boolean deposit(String ChkAcctNumber, float amount) { try { DBConnection ToDB = new DBConnection(); Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM CheckingAccount WHERE CheckingAccountNumber ='"+ ChkAcctNumber + "'"; // SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command);
while (Rslt.next()) { Balance = Rslt.getFloat(1); System.out .println("The Current Balance in Checking Account is %f" + Balance); Balance = Balance + amount; System.out.println("Bal deposit: " + Balance); Statement Stmt2 = DBConn.createStatement(); String sqlcmd = "update CheckingAccount set Balance=" + Balance + " where CheckingAccountNumber=" + ChkAcctNumber + ""; Stmt2.executeUpdate(sqlcmd); System.out .println("The Current Balance in Checking Account after Depositing %f is %f" + Balance); } Stmt.close(); ToDB.closeConn(); } catch (java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace(); }
return true; } }
SavingsAccount.java
package Com.Patel;
import java.lang.*; //including Java packages used by this program import java.sql.*; import Com.Patel.*;
public class SavingsAccount
{ //Instance Variables private String SavingsAccountNumber, CustomerName, CustomerID; private float Balance, Interest;
/*Constructors*/ public SavingsAccount(String SA_Num, String Cu_Name, String Cu_ID, float Bal,float InterestRate) { SavingsAccountNumber = SA_Num; CustomerName = Cu_Name; CustomerID = Cu_ID; Balance =Bal; Interest =InterestRate;
} ///public SavingsAccount(String SA_Num) //{ // SavingsAccountNumber = SA_Num; //} public SavingsAccount(String Cust_ID) { CustomerID=Cust_ID; } /* method */ public boolean openAcct() { boolean done = false; try { if (!done) { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT SavingsAccountNumber FROM SavingsAccount WHERE SavingsAccountNumber ='"+SavingsAccountNumber+"'"; //SQL query command ResultSet Rslt = Stmt.executeQuery(SQL_Command); //Inquire if the username exsits. done = !Rslt.next(); if (done) { SQL_Command = "INSERT INTO SavingsAccount(SavingsAccountNumber, CustomerName, Balance, InterestRate, CustomerID)"+ " VALUES ('"+SavingsAccountNumber+"','"+CustomerName+"',"+Balance+",'"+ Interest +"', '"+CustomerID+"')"; //Save the username, password and Name Stmt.executeUpdate(SQL_Command); } Stmt.close(); ToDB.closeConn(); } } catch(java.sql.SQLException e) { done = false; System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { done = false; System.out.println("Exception: " + e); e.printStackTrace (); } return done; }
public float calculateInterests(String SavngsAcct,float InterestRate) {
try { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM SavingsAccount WHERE SavingsAccountNumber ='"+SavingsAccountNumber+"'"; //SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command); while (Rslt.next()) { float interest = getBalance() * Interest/100; // deposit(interest);
InterestRate=Rslt.getFloat(1); } Stmt.close(); ToDB.closeConn(); } catch(java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace (); } return Balance; }
public float getBalance() { //Method to return a SavingsAccount balance try { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM SavingsAccount WHERE SavingsAccountNumber ='"+SavingsAccountNumber+"'"; //SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command); while (Rslt.next()) { Balance = Rslt.getFloat(1); } Stmt.close(); ToDB.closeConn(); } catch(java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace (); } return Balance; } public float getBalance(String SavngsAcct) { //Method to return a SavingsAccount balance try { DBConnection ToDB = new DBConnection(); //Have a connection to the DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM SavingsAccount WHERE SavingsAccountNumber ='"+SavngsAcct+"'"; //SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command); while (Rslt.next()) { Balance = Rslt.getFloat(1); } Stmt.close(); ToDB.closeConn(); } catch(java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace (); } return Balance; } public boolean Withdraw(String SAcctNumber, double amount) { boolean done = !SAcctNumber.equals(""); double balance = getBalance(SAcctNumber); System.out.println("The current balance in saving account is " + Balance); if (Double.compare(balance - amount, amount)
public boolean deposit(String SAcctNumber, float amount) { float newBalance; try { DBConnection ToDB = new DBConnection(); // Have a connection to the // DB Connection DBConn = ToDB.openConn(); Statement Stmt = DBConn.createStatement(); String SQL_Command = "SELECT Balance FROM SavingsAccount WHERE SavingsAccountNumber ='"+ SAcctNumber + "'"; // SQL query command for Balance ResultSet Rslt = Stmt.executeQuery(SQL_Command);
while (Rslt.next()) { Balance = Rslt.getFloat(1); System.out.println("The current balancein Saving account is " + Balance); Balance = Balance + amount; Statement Stmt2 = DBConn.createStatement(); String sqlcmd = "update SavingsAccount set Balance = " + Balance + " where SavingsAccountNumber='" + SAcctNumber + "'"; Stmt2.executeUpdate(sqlcmd); System.out .println("The present balancein Saving account after depositing is " + Balance);
} Stmt.close(); ToDB.closeConn(); } catch (java.sql.SQLException e) { System.out.println("SQLException: " + e); while (e != null) { System.out.println("SQLState: " + e.getSQLState()); System.out.println("Message: " + e.getMessage()); System.out.println("Vendor: " + e.getErrorCode()); e = e.getNextException(); System.out.println(""); } } catch (java.lang.Exception e) { System.out.println("Exception: " + e); e.printStackTrace(); }
return true; } }
this form is login form,sign up form, open bank form.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
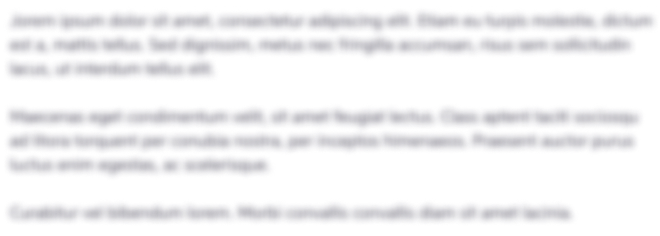
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started