Question
Implement member functions of a Matrix class in a file named Matrix.cpp that stores elements of a matrix and is capable of performing basic operations.The
Implement member functions of a Matrix class in a file named "Matrix.cpp" that stores elements of a matrix and is capable of performing basic operations.The code listing Matrix.h is the header file that describes the matrix class.
Required Matrix interface (Matrix.h)
#include#include #include using namespace std; class Matrix { private: vector > M; unsigned int ROWS; unsigned int COLS; bool operationIsValid(const Matrix &, const string&) const; public: //Constructors Matrix(); Matrix(const string&); Matrix(int,int,int); //Accessors const Matrix transpose() const; const Matrix add(const Matrix &m) const; const Matrix subtract(const Matrix &m) const; const Matrix multiply(const Matrix &m) const; const void saveMatrix(const string&) const; //Friend function friend ostream & operator<<(ostream &, const Matrix &); };
Encapsulated (Private) Data Fields
-vector
-ROWS: stores the number of rows.
-COLS: stores the number of columns.
-bool operationIsValid(const Matrix &, const string&) const: passes in a matrix and a string that contains one of the following values: "ADD", "SUBTRACT" or "MULTIPLY". Based on the operation that is indicated by string parameter and sizes of matrices, the function returns true if the operation is valid otherwise returns false.
For example: if A and B are matrices of the sizes 3 X 3 and 2 X 3 then, A.operationIsValid(B,"ADD") returns false.
Public Interface (Public Member Functions)
Note: in following examples A, B and C are objects of type Matrix and a[i][j], b[i][j], c[i][j] denote the actual elements located at ith row and jth column of matrix A, B and C, respectively.
-Matrix(): creates a 3 by 3 matrix and assigns 0 to each element of the matrix.
-Matrix(const string&): passes in a string containing the path to the matrix file (e. g. "input.dat"), reads the file and populates values to the private members (COLS, ROWS, M) an example of a file is described in GLOBAL FUNCTION section. If the function cannot open the file it should print to the console the following error message: "An error occurred in opening the input.dat file" and exit the program with following command: exit(1).
-Matrix(int r,int c,int val) passes in three integers and creates a r X c matrix (matrix containing r rows and c columns). Also, each element should be set to val.
-const Matrix transpose() const: returns an object of type Matrix which is a transpose of the matrix. By transpose, we mean interchanging the rows and columns of a matrix.
For example: if A = B.transpose(), then a[i][j] = b[j][i].
-const Matrix add(const Matrix &m) const: adds the corresponding elements of the two matrices and returns result.
For example: if C = A.add(B), then c[i][j] = a[i][j] + b[i][j].
-const Matrix subtract(const Matrix &m) const: subtracts the elements of the two matrices and returns result.
For example: if C = A.subtract(B), then c[i][j] = a[i][j] - b[i][j].
-const Matrix multiply(const Matrix &m) const: multiplies the elements of the two matrices and returns result.
For example: (A and B are 2 X 2 matrices) if C = A.multiply(B) and , then c[0][0] = a[0][0] * b[0][0] + a[0][1] * b[1][0].
-const void saveMatrix(const string&) const: passes in a filename (e. g. "matrix.dat") and saves its elements into the file. The format is described in GLOBAL FUNCTION section.
Global Function
-friend ostream & operator<<(ostream &, const Matrix &): implement global function in "main.cpp" that passes in a Matrix and outputs to the stream the elements of matrix in a certain format as follows: The number of characters for outputting each element should be set to 5 (check section 9.3 for output formatting) and elements should be aligned left. For example: if Matrix contains two rows and five columns. Elements in first row are equal to 3 and elements in second row are equal to 7 then the output would be as follows:
2 5 3 3 3 3 3 7 7 7 7 7
You should use the following "main.cpp" file. You should not make any changes to the "main.cpp" other than declaring and defining "operator<<" function as described above.
#include "Matrix.h" #include#include #include //declare operator<< function in here ostream & operator<<(ostream &, const Matrix &); int main(){ int operationChoice = -1; int displayChoice = -1; string matFile1; string matFile2; string resFile; Matrix res; cout << "Enter operation (1-4):" << endl << "1 - Addition (A + B)" << endl << "2 - Subtraction (A - B)" << endl << "3 - Multiplication (A * B)" << endl << "4 - Transpose A" << endl; cin >> operationChoice; if(operationChoice == 1 || operationChoice == 2 || operationChoice == 3) { cout << "Enter the filename for the first matrix: " << endl; cin >> matFile1; cout << "Enter the filename for the second matrix: " << endl; cin >> matFile2; Matrix A(matFile1); Matrix B(matFile2); if(operationChoice == 1) res = A.add(B); if(operationChoice == 2) res = A.subtract(B); if(operationChoice == 3) res = A.multiply(B); } else if(operationChoice == 4) { cout << "Enter the file name for matrix: " << endl; cin >> matFile1; Matrix A(matFile1); res = A.transpose(); } cout << "Display result (1-2):" << endl << "1 - Save into a file" << endl << "2 - Display in console" << endl; cin >> displayChoice; if(displayChoice == 1){ cout << "Enter the file name for saving result: " << endl; cin >> resFile; res.saveMatrix(resFile); } else if(displayChoice == 2){ cout << res; } return 0; } //define operator<< function in here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
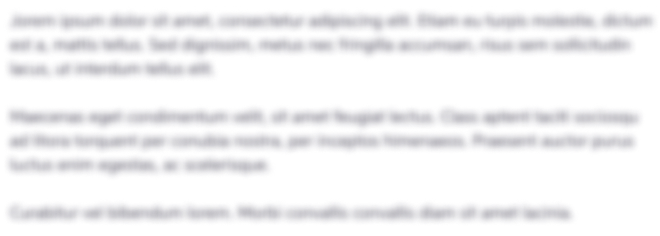
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started