Question
Implement refactored classes and draw the UML class Diagram for the following 6 classes in Java : -------------------------------------------------------------------------------- 1. university class: import java.util.*; public class
Implement refactored classes and draw the UML class Diagram for the following 6 classes in Java :
--------------------------------------------------------------------------------
1. university class:
import java.util.*;
public class university
{
public static void main(String[] args)
{
School engSchool = new School("Computing School");
Student s = new Student("Jason", "Fisherman", "Freshman", "CS/CSE", 3.5);
Faculty f = new Faculty("Mick", "Armstrong", "ENG", "SOA", false, 1000.00, 0);
OfficeStaff os= new OfficeStaff("Bella", "Broudy", true , 0, 20.00);
TechnicalSupportStaff ts = new TechnicalSupportStaff("Clara", "Ray" , "Database", false, 12000.50, 0);
engSchool.addstudent(s);
engSchool.addfaculty(f);
engSchool.addofficeStaff(os);
engSchool.addTSS(ts);
engSchool.displaySchoolInfo();
}
}
--------------------------------------------------------------------------------
2. School:
import java.util.*;
public class School
{
private String schoolName;
private ArrayList
private ArrayList
private ArrayList
private ArrayList
public School(String name)
{
schoolName = name;
students = new ArrayList();
faculty = new ArrayList();
o_staff = new ArrayList();
ts_staff = new ArrayList();
}
public void addstudent(Student s)
{
students.add(s);
}
public void addfaculty(Faculty f)
{
faculty.add(f);
}
public void addofficeStaff(OfficeStaff os)
{
o_staff.add(os);
}
public void addTSS(TechnicalSupportStaff tss)
{
ts_staff.add(tss);
}
public void displaySchoolInfo()
{
System.out.println("\t\t"+ schoolName);
System.out.println("\t\t\t =============");
for(int i=0; i students.get(i).viewStudent(); for(int i=0; i faculty.get(i).viewFaculty(); for(int i=0; i o_staff.get(i).viewOfficeStaff(); for(int i=0; i ts_staff.get(i).viewTechnicalSupportStaff(); } } -------------------------------------------------------------------------------- 3.Student public class Student { private String firstName; private String lastName; private String major; private double gpa; private String academicLevel; public Student(String fName, String lName, String al, String m, double gp) { firstName = fName; lastName = lName; major = m; gpa = gp; academicLevel = al; } public void viewStudent() { System.out.println(); System.out.println("First Name :" + firstName); System.out.println("Last Name :" + lastName); System.out.println("Major:" + major); System.out.println("GPA :" + gpa); System.out.println("academic Level :" + academicLevel); } } -------------------------------------------------------------------------------- 4. Faculty public class Faculty { private String firstName; private String lastName; private String department; private String researchArea; private double monthly_salary; private boolean hourlyPaid; private double hourlyRate; public Faculty(String fName, String lName, String dep, String res, boolean hp, double ms, double hr) { firstName= fName; lastName = lName; department = dep; researchArea = res; hourlyPaid = hp; monthly_salary = ms; hourlyRate = hr; } public void viewFaculty() { System.out.println(); System.out.println("First Name:" + firstName); System.out.println("Last Name:" + lastName); System.out.println("Department:" + department); System.out.println("Research Area:" + researchArea); if(hourlyPaid) System.out.println("Hourly Rate:" + hourlyRate); else System.out.println("Monthly Salary:" + monthly_salary); } } -------------------------------------------------------------------------------- 5. OfficeStaff public class OfficeStaff { private String firstName; private String lastName; private boolean hourlyPaid; private double hourlyRate; private double monthly_salary; public OfficeStaff(String fName, String lName, boolean hp, double ms, double hr) { firstName= fName; lastName = lName; hourlyPaid = hp; monthly_salary = ms; hourlyRate = hr; } public void viewOfficeStaff(){ System.out.println(); System.out.println("First Name:" + firstName); System.out.println("Last Name:" + lastName); if(hourlyPaid) System.out.println("Hourly Rate:" + hourlyRate); else System.out.println("Monthly Salary:" + monthly_salary); } } -------------------------------------------------------------------------------- 6.TechnicalSupportStaff public class TechnicalSupportStaff { private String firstName; private String lastName; private String technicalArea; private boolean hourlyPaid; private double hourlyRate; private double monthly_salary; public TechnicalSupportStaff(String fName, String lName, String ta, boolean hp, double ms, double hr) { firstName= fName; lastName = lName; hourlyPaid = hp; monthly_salary = ms; hourlyRate = hr; technicalArea = ta; } public void viewTechnicalSupportStaff() { System.out.println(); System.out.println("First Name:" + firstName); System.out.println("Last Name:" + lastName); System.out.println("Technical Area:" + technicalArea); if(hourlyPaid) System.out.println("Hourly Rate:" + hourlyRate); else System.out.println("Monthly Salary:" + monthly_salary); } } --------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
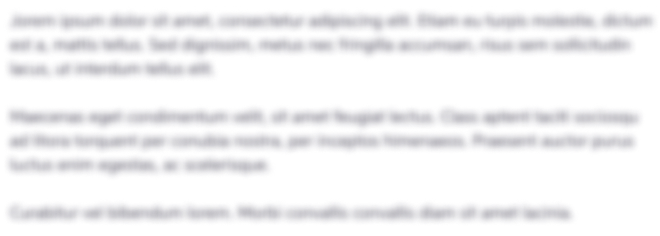
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started