Question
Implement the AVL class to store the simplified resumes of all applicants. Member Functions: Implement the following member functions: AVL(bool isAVL) Simple default constructor to
Implement the AVL class to store the simplified resumes of all applicants. Member Functions: Implement the following member functions:
AVL(bool isAVL) Simple default constructor to initialize the isAVL flag. If the isAVL flag is set, insertions and deletions will follow AVL property. Otherwise, it will be a simple BST.
void insertNode(shared_ptr> N) Inserts the given node into the tree such that the AVL (or BST) property holds.
void deleteNode(T k) Deletes the node with the given key such that the AVL (or BST) property holds. As convention, while deleting a node with 2 children, the new root should be selected from the right subtree.
shared_ptr> searchNode(T k) Returns the pointer to the node with the given key. NULL is returned if the key doesnt exist.
shared_ptr> getRoot() Returns the pointer to the root node of the tree.
int height(shared_ptr> p) Returns the height of the tree. the height of a tree is the number of nodes in the path from the root to its deepest descendant. For example, a tree with only two nodes (a root and a child) will have height 2 and so on.
Starter code:
template
struct node {
S fullName;
S gender;
T workExperience;
shared_ptr
shared_ptr
int height;
node(S n, S g, T w) {
this->fullName = n;
this->gender = g;
this->workExperience = w;
left = NULL;
right = NULL;
height = 1;
}
};
// AVL Class (This will be used for both BST and AVL Tree implementation)
template
class AVL {
shared_ptr
bool isAVL;
public:
AVL(bool);
void insertNode(shared_ptr
void deleteNode(T k);
shared_ptr
shared_ptr
int height (shared_ptr
// Declare helper functions here
Code all functions of Task 1 in c++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
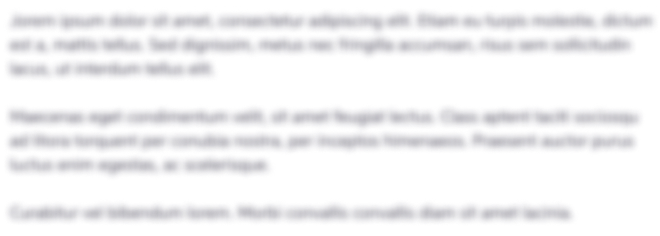
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started