Question
implement the following methods for the Binary Search Tree where indicated in the java code: import java.util.Scanner; public class BST { private Node root; //
implement the following methods for the Binary Search Tree where indicated in the java code:
import java.util.Scanner;
public class BST
private Node root; // root of binary search tree
private class Node {
private Key key; // sorted by key
private Value value; // data stored in the node
private Node left, right; // pointers to left and right subtrees
private int size; // number of nodes in subtree
public Node(Key k, Value v) {
this.key = k;
this.value = v;
}
}
// Insert key-value pairs in BST, if a key already exists update the value
public void insert(Key key, Value value){
if (root == null)
root = node;
left = null;
right = null;
else
cur = root;
while(cur != null)
{
if(node.compareTo(cur)
if(left = null)
left = node;
cur = null;
else
cur = left;
else
if(right = null)
right = node;
cur = null;
else
cur = right;
left = null;
right = null;return root;
// Implement insertion of a key-value pair in a BST.
}
// Search BST for a given key
public Value get(Key key) {
// Implement search for a given key
return null;
}
public void inorder(Node root){
// Implement Inorder Traversal
}
public static void main(String[] args){
Scanner input = new Scanner(System.in);
BST
while(input.hasNext()){
int key = input.nextInt();
if(key == -1) break;
String value = input.next();
bst.insert(key, value);
}
System.out.println("Search for key: 20");
System.out.println(bst.get(20));
System.out.println("Inorder Traversal prints:");
bst.inorder(bst.root);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
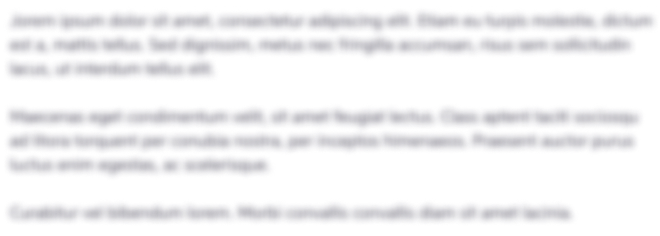
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started