Question
Implement with the PriorityQueue structure for the solution of the Processing customers in a bank row. a. Implement a client class with the following attributes
Implement with the PriorityQueue structure for the solution of the Processing customers in a bank row. a. Implement a client class with the following attributes Yo. Status: Arrival (A) or Departure (D) ii. Time of arrival iii. processing time iv. Priority : People with special needs (1), Women pregnant women (2), people of legal age (3), and the rest of the people that make up the row (4). These will be randomly generated b. Use the data generated from problem 6 p. 393 Cap 11. To show the execution of its implementation.
all the code need it
#include #include using namespace::std; //#include "LinkedSortedList.h" #include "SL_PriorityQueue.h" int main(){ //What is the output of the following pseudocode, where num1 , num2 , and num3 are integer variables? SL_PriorityQueue aQueue; //LinkedSortedList l; int num1 = 5; int num2 = 1; int num3 = 4; aQueue.add(num2); aQueue.add(num3); aQueue.remove(); aQueue.add(num1 - num2); num1 = aQueue.peek(); aQueue.remove(); num2 = aQueue.peek(); aQueue.remove(); cout class SL_PriorityQueue : public PriorityQueueInterface { private: LinkedSortedList* slistPtr; // Pointer to sorted list of // items in the priority queue LinkedSortedList* copyChain(const LinkedSortedList* origChainPtr); public: SL_PriorityQueue(); SL_PriorityQueue(const SL_PriorityQueue& pq); ~SL_PriorityQueue(); bool isEmpty() const; bool add(const ItemType& newEntry); bool remove(); /** @throw PrecondViolatedExcep if priority queue is empty. */ ItemType peek() const throw(PrecondViolatedExcep); void display() const; }; // end SL_PriorityQueue // Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2013 __Pearson Education__. All rights reserved. // PARITALLY COMPLETE. /** ADT priority queue: ADT sorted list implementation. @file SL_PriorityQueue.cpp */ //#include "SL_PriorityQueue.h" // Header file template SL_PriorityQueue::SL_PriorityQueue() : slistPtr(nullptr) { slistPtr = new LinkedSortedList(); } template SL_PriorityQueue::SL_PriorityQueue(const SL_PriorityQueue& pq) { slistPtr = copyChain(pq->slistPtr); } template SL_PriorityQueue::~SL_PriorityQueue() { } template bool SL_PriorityQueue::isEmpty() const { return slistPtr == 0; } template bool SL_PriorityQueue::add(const ItemType& newEntry) { slistPtr->insertSorted(newEntry); return true; } // end add template bool SL_PriorityQueue::remove() { // The highest priority item is at the end of the sorted list return slistPtr->remove(slistPtr->getLength()); } // end remove template ItemType SL_PriorityQueue::peek()const throw(PrecondViolatedExcep) { int position = slistPtr->getLength(); // Enforce precondition if (isEmpty()) throw PrecondViolatedExcep("peekFront() called with empty queue"); else{ return slistPtr->getEntry(position); } } // end peekFront //Private Methods: template LinkedSortedList* SL_PriorityQueue::copyChain(const LinkedSortedList* origChainPtr) { LinkedSortedList* copiedChainPtr; if (origChainPtr == nullptr) { copiedChainPtr = nullptr; } else { // Build new chain from given one copiedChainPtr = new LinkedSortedList(origChainPtr->getItem()); copiedChainPtr->setNext(copyChain(origChainPtr->getNext())); } // end if return copiedChainPtr; } // end copyChain template void SL_PriorityQueue::display() const { slistPtr->display(); } #endif +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ #ifndef _PRIORITY_QUEUE_INTERFACE #define _PRIORITY_QUEUE_INTERFACE template class PriorityQueueInterface { public: virtual bool isEmpty() const = 0; virtual bool add(const ItemType& newEntry) = 0; virtual bool remove() = 0; virtual ItemType peek() const = 0; virtual void display() const = 0; }; #endif ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ // Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2013 __Pearson Education__. All rights reserved. // Listing 12-2 /** ADT sorted list: Link-based implementation. //@file LinkedSortedList.h */ #ifndef _LINKED_SORTED_LIST #define _LINKED_SORTED_LIST #include #include using namespace::std; #include "SortedListInterface.h" #include "Node.h" #include "PrecondViolatedExcep.h" template class LinkedSortedList : public SortedListInterface { private: Node* headPtr; // Pointer to first node in the chain int itemCount; // Current count of list items // Locates the node that is before the node that should or does // contain the given entry. // @param anEntry The entry to find. // @return Either a pointer to the node before the node that contains // or should contain the given entry, or nullptr if no prior node exists. Node* getNodeBefore(const ItemType& anEntry) const; // Locates the node at a given position within the chain. Node* getNodeAt(int position) const; // Returns a pointer to a copy of the chain to which origChainPtr points. Node* copyChain(const Node* origChainPtr); Node* getPointerTo(const ItemType& anEntry) const; public: LinkedSortedList(); LinkedSortedList(const LinkedSortedList& aList); virtual ~LinkedSortedList(); void insertSorted(const ItemType& newEntry); bool removeSorted(const ItemType& anEntry); int getPosition(const ItemType& newEntry); // The following methods are the same as given in ListInterface: bool isEmpty() const; int getLength() const; bool remove1(int position); bool remove(const ItemType& anEntry); void clear(); ItemType getEntry(int position) const throw(PrecondViolatedExcep); void display() const; }; // end LinkedSortedList //#include "LinkedSortedList.cpp" template void LinkedSortedList::display() const { Node* currentPtr = headPtr; while (currentPtr != nullptr){ cout getItem() getNext(); }//end while } template LinkedSortedList::LinkedSortedList() : itemCount(0), headPtr(nullptr) { } template LinkedSortedList::LinkedSortedList(const LinkedSortedList& aList) { headPtr = copyChain(aList.headPtr); } // end copy constructor template void LinkedSortedList::insertSorted(const ItemType& newEntry) { Node* newNodePtr = new Node(newEntry); Node* prevPtr = getNodeBefore(newEntry); if (isEmpty() || (prevPtr == nullptr)) // Add at beginning { newNodePtr->setNext(headPtr); headPtr = newNodePtr; } else // Add after node before { Node* aftPtr = prevPtr->getNext(); newNodePtr->setNext(aftPtr); prevPtr->setNext(newNodePtr); } // end if itemCount++; } // end insertSorted // Private Methods: template LinkedSortedList::~LinkedSortedList() { clear(); } // end destructor template Node* LinkedSortedList::copyChain(const Node* origChainPtr) { Node* copiedChainPtr; if (origChainPtr == nullptr) { copiedChainPtr = nullptr; } else { // Build new chain from given one copiedChainPtr = new Node(origChainPtr->getItem()); copiedChainPtr->setNext(copyChain(origChainPtr->getNext())); } // end if return copiedChainPtr; } // end copyChain template Node* LinkedSortedList::getNodeBefore(const ItemType& anEntry) const { Node* curPtr = headPtr; Node* prevPtr = nullptr; while ((curPtr != nullptr) && (anEntry > curPtr->getItem())) { prevPtr = curPtr; curPtr = curPtr->getNext(); } // end while return prevPtr; } // end getNodeBefore template bool LinkedSortedList ::isEmpty() const{ return itemCount == 0; } template int LinkedSortedList ::getLength() const{ return itemCount; } template bool LinkedSortedList::remove(const ItemType& anEntry) { Node* entryNodePtr = getPointerTo(anEntry); bool canRemoveItem = !isEmpty() && (entryNodePtr != nullptr); if (canRemoveItem) { // Copy data from first node to located node entryNodePtr->setItem(headPtr->getItem()); // Delete first node Node* nodeToDeletePtr = headPtr; headPtr = headPtr->getNext(); // Return node to the system nodeToDeletePtr->setNext(nullptr); delete nodeToDeletePtr; nodeToDeletePtr = nullptr; itemCount--; } // end if return canRemoveItem; } template bool LinkedSortedList ::remove1(int position){ bool ableToRemove = (position >= 1) && (position * curPtr = nullptr; if (position == 1) { // Remove the first node in the chain curPtr = headPtr; // Save pointer to node headPtr = headPtr->getNext(); } else { // Find node that is before the one to delete Node* prevPtr = getNodeAt(position - 1); // Point to node to delete curPtr = prevPtr->getNext(); // Disconnect indicated node from chain by connecting the // prior node with the one after prevPtr->setNext(curPtr->getNext()); } // end if // Return node to system curPtr->setNext(nullptr); delete curPtr; curPtr = nullptr; itemCount--; // Decrease count of entries } // end if return ableToRemove; } template void LinkedSortedList ::clear(){ while (!isEmpty()) remove1(1); } template ItemType LinkedSortedList ::getEntry(int position) const throw(PrecondViolatedExcep){ // Enforce precondition bool ableToGet = (position >= 1) && (position * nodePtr = getNodeAt(position); return nodePtr->getItem(); } else { string message = "getEntry() called with an empty list or "; message = message + "invalid position."; throw(PrecondViolatedExcep(message)); } // end if } // Locates the node at a given position within the chain. template Node*LinkedSortedList ::getNodeAt(int position) const{ // Debugging check of precondition assert((position >= 1) && (position * curPtr = headPtr; for (int skip = 1; skip getNext(); return curPtr; } template Node* LinkedSortedList::getPointerTo(const ItemType& anEntry) const { bool found = false; Node* curPtr = headPtr; while (!found && (curPtr != nullptr)) { if (anEntry == curPtr->getItem()) found = true; else curPtr = curPtr->getNext(); } // end while return curPtr; } // end getPointerTo template bool LinkedSortedList::removeSorted(const ItemType& anEntry) { bool ableToRemove = false; if (!isEmpty()) { int position = getPosition(anEntry); ableToRemove = position > 0; if (ableToRemove) { ableToRemove = remove1(position); } // end if } // end if return ableToRemove; } // end removeSorted template int LinkedSortedList::getPosition(const ItemType& anEntry) { int position = 1; int length = LinkedSortedList::getLength(); while ((position LinkedSortedList::getEntry(position))) { position++; } // end while if ((position > length) || (anEntry != LinkedSortedList::getEntry(position))) { position = -position; } // end if return position; } // end getPosition #endif ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ #ifndef _SORTED_LIST_INTERFACE #define SORTED_LIST_INTERFACE template class SortedListInterface { public: /** Inserts an entry into this sorted list in its proper order so that the list remains sorted. @pre None. @post newEntry is in the list, and the list is sorted. @param newEntry The entry to insert into the sorted list. */ virtual void insertSorted(const ItemType& newEntry) = 0; /** Removes the first or only occurrence of the given entry from this sorted list. @pre None. @post If the removal is successful, the first occurrence of the given entry is no longer in the sorted list, and the returned value is true. Otherwise, the sorted list is unchanged and the returned value is false. @param anEntry The entry to remove. @return True if removal is successful, or false if not. */ virtual bool removeSorted(const ItemType& anEntry) = 0; /** Gets the position of the first or only occurrence of the given entry in this sorted list. In case the entry is not in the list, determines where it should be if it were added to the list. @pre None. @post The position where the given entry is or belongs is returned. The sorted list is unchanged. @param anEntry The entry to locate. @return Either the position of the given entry, if it occurs in the sorted list, or the position where the entry would occur, but as a negative integer. */ virtual int getPosition(const ItemType& anEntry) = 0; // The following methods are the same as those given in ListInterface // in Listing 8-1 of Chapter 8 and are completely specified there. /** Sees whether this list is empty. */ virtual bool isEmpty() const = 0; /** Gets the current number of entries in this list. */ virtual int getLength() const = 0; /** Removes the entry at a given position from this list. */ virtual bool remove1(int position) = 0; virtual bool remove(const ItemType& anEntry) = 0; /** Removes all entries from this list. */ virtual void clear() = 0; /** Gets the entry at the given position in this list. */ virtual ItemType getEntry(int position) const = 0; virtual void display() const = 0; }; // end SortedListInterface #endif +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ // Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2013 __Pearson Education__. All rights reserved. /** @file Node.h Listing 4-1 */ #ifndef _NODE #define _NODE template class Node { private: ItemType item; // A data item Node* next; // Pointer to next node public: Node(); Node(const ItemType& anItem); Node(const ItemType& anItem, Node* nextNodePtr); void setItem(const ItemType& anItem); void setNext(Node* nextNodePtr); ItemType getItem() const; Node* getNext() const; }; // end Node // Created by Frank M. Carrano and Tim Henry. // Copyright (c) 2013 __Pearson Education__. All rights reserved. /** @file Node.cpp Listing 4-2 */ #include "Node.h" #include template Node::Node() : next(nullptr) { } // end default constructor template Node::Node(const ItemType& anItem) : item(anItem), next(nullptr) { } // end constructor template Node::Node(const ItemType& anItem, Node* nextNodePtr) : item(anItem), next(nextNodePtr) { } // end constructor template void Node::setItem(const ItemType& anItem) { item = anItem; } // end setItem template void Node::setNext(Node* nextNodePtr) { next = nextNodePtr; } // end setNext template ItemType Node::getItem() const { return item; } // end getItem template Node* Node::getNext() const { return next; } // end getNext #endif +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ #ifndef _PRECOND_VIOLATED_EXCEP #define _PRECOND_VIOLATED_EXCEP #include #include using namespace std; class PrecondViolatedExcep : public logic_errora { public: PrecondViolatedExcep(const string& message = ""); }; // end PrecondViolatedExcep #include "PrecondViolatedExcep.h" PrecondViolatedExcep::PrecondViolatedExcep(const string& message) : logic_error("Precondition ViolatedException: " + message) { } // end constructor // End of implementation file. #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
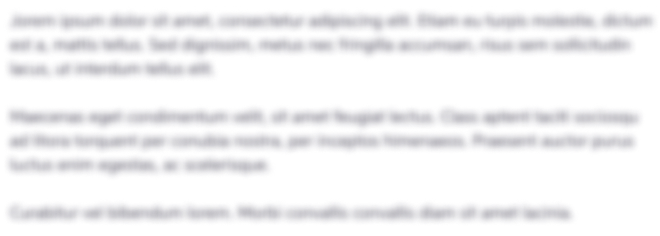
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started