Question
Implement your interface from Chapter 7 using event handling In Chapter 7 your assignment was to Design a universal remote control for an entertainment system
Implement your interface from Chapter 7 using event handling In Chapter 7 your assignment was to Design a universal remote control for an entertainment system (cable / TV, etc). Create the interface as a NetBeans project. Or implement the design of the telephone interface for a smartphone.
The assignment cannot be created using the GUI Drag and Drop features in NetBeans. You must actually code the GUI application using a coding framework similar to one described and illustrated in chapter on GUI development in the courses textbook.
In this assignment you need to have event listeners associated with the components on the GUI. This assignment is about creating a few event handlers to acknowledge activity on you interface. Acknowledgment of activity can be as simple as displaying a text message when an event occurs with a component on your interface such as; a button is pressed, a check box or radio button is clicked, a slider is moved or a combo box item is selected.
package cellphone;
import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.text.*;
public class CellPhone extends JFrame {
private final String WINDOW_TITLE = "CellPhone"; private final int WINDOW_WIDTH = 200; private final int WINDOW_HEIGHT = 300; String input;
private final JPanel textPanel = new JPanel(); private final JPanel digitPanel = new JPanel(); private final JPanel buttonPanel = new JPanel();
private final JLabel displayLabel = new JLabel (" Android ");
private final JTextField displayTextField = new JTextField(20); private final JButton sendButton = new JButton("Send"); private final JButton clearButton = new JButton("Clear"); private final JButton endButton = new JButton(" End "); private final JButton Button1 = new JButton("1"); private final JButton Button2 = new JButton("2"); private final JButton Button3 = new JButton("3"); private final JButton Button4 = new JButton("4"); private final JButton Button5 = new JButton("5"); private final JButton Button6 = new JButton("6"); private final JButton Button7 = new JButton("7"); private final JButton Button8 = new JButton("8"); private final JButton Button9 = new JButton("9"); private final JButton Button0 = new JButton("0"); private final JButton astrButton = new JButton("*"); private final JButton boundButton = new JButton("#"); private final DecimalFormat df2 = new DecimalFormat("#,###.00");
public static void main(String[] args) { new CellPhone(); }
public CellPhone() {
setTitle(WINDOW_TITLE); setSize(WINDOW_WIDTH, WINDOW_HEIGHT); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); buildTextPanel(); buildButtonPanel(); buildDigitPanel(); setLayout(new BorderLayout()); add(textPanel,BorderLayout.NORTH); add(buttonPanel,BorderLayout.CENTER); add(digitPanel,BorderLayout.SOUTH); clearDisplay(); setVisible(true);
}
public void buildTextPanel () { textPanel.setLayout(new BorderLayout()); textPanel.add(displayLabel,BorderLayout.NORTH); textPanel.add(displayTextField,BorderLayout.CENTER); textPanel.setBackground(Color.GREEN); }
public void buildButtonPanel () { buttonPanel.setLayout(new FlowLayout()); buttonPanel.add(sendButton); buttonPanel.add(clearButton); buttonPanel.add(endButton); sendButton.addActionListener(new SendButtonListener()); clearButton.addActionListener(new ClearButtonListener()); endButton.addActionListener(new EndButtonListener()); buttonPanel.setBackground(Color.GREEN); }
public void buildDigitPanel () { digitPanel.setLayout(new GridLayout(4,3)); digitPanel.add(Button1); digitPanel.add(Button2); digitPanel.add(Button3); digitPanel.add(Button4); digitPanel.add(Button5); digitPanel.add(Button6); digitPanel.add(Button7); digitPanel.add(Button8); digitPanel.add(Button9); digitPanel.add(astrButton); digitPanel.add(Button0); digitPanel.add(boundButton);
InnerListener listener= new InnerListener(); Button1.addActionListener(listener); Button2.addActionListener(listener); Button3.addActionListener(listener); Button4.addActionListener(listener); Button5.addActionListener(listener); Button6.addActionListener(listener); Button7.addActionListener(listener); Button8.addActionListener(listener); Button9.addActionListener(listener); Button0.addActionListener(listener); astrButton.addActionListener(listener); boundButton.addActionListener(listener); }
private class SendButtonListener implements ActionListener {
@Override public void actionPerformed(ActionEvent e) {
input= displayTextField.getText(); sendCalling(); } }
private class ClearButtonListener implements ActionListener {
@Override public void actionPerformed(ActionEvent e) {
clearDisplay(); } }
private class EndButtonListener implements ActionListener {
@Override public void actionPerformed(ActionEvent e) {
endCalling(); } }
public void clearDisplay() { displayTextField.setText(" "); }
public void sendCalling() { displayTextField.setText("Calling " + input+ input+ input+ "-" + input+ input+input+ "-" +input+ input+ input+ input); }
public void endCalling() { displayTextField.setText("Call Ended"); }
private class InnerListener implements ActionListener {
@Override public void actionPerformed(ActionEvent e) { if(e.getSource()==Button1) { displayTextField.setText("1"); } else if (e.getSource()==Button2) { displayTextField.setText("2"); } else if (e.getSource()==Button3) { displayTextField.setText("3"); } else if (e.getSource()==Button4) { displayTextField.setText("4"); } else if (e.getSource()==Button5) { displayTextField.setText("5"); } else if (e.getSource()==Button6) { displayTextField.setText("6"); } else if (e.getSource()==Button7) { displayTextField.setText("7"); } else if (e.getSource()==Button8) { displayTextField.setText("8"); } else if (e.getSource()==Button9) { displayTextField.setText("9"); } else if (e.getSource()==Button0) { displayTextField.setText("0"); } else if (e.getSource()==astrButton) { displayTextField.setText("*"); } else if (e.getSource()==boundButton) { displayTextField.setText("#"); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
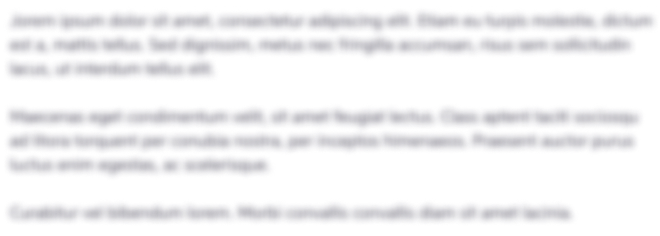
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started