Question
Implementing and testing all three sort algorithms: Bubble Sort , Selection Sort , and Insertion Sort . In additions, you will also be writing a
Implementing and testing all three sort algorithms: Bubble Sort , Selection Sort , and Insertion Sort . In additions, you will also be writing a driver to test the search algorithms and you will be measuring the run times of each search. You will also be using the RunTime class that you created for Homework 1. Finally, you will be analysing and comparing the performance of the three sort algorithms based on the type of array that was being sorted and the run times you computed.
Details
RunTime Class
import java.util.Arrays;
public class RunTime implements RuntimeInterface {
private static final int MAX = 10; private long[] runtimes; private int count; public RunTime() { this.runtimes = new long[MAX]; this.count += 0; }
@Override public void addRuntime(long runTime) { if (this.count == MAX) { for (int i = 0; i < (MAX - 1); i++) { this.runtimes[i] = this.runtimes[i + 1]; } this.runtimes[MAX - 1] = runTime; } else {
this.runtimes[count] = runTime; this.count += 1; } }
@Override public double getAverageRunTime() { double sum = 0; for (int i = 0; i < this.count; i++) { sum += this.runtimes[i]; } return (sum / this.count); }
@Override public long getLastRunTime() { return this.runtimes[this.count - 1]; }
@Override public long[] getRunTimes() { return Arrays.copyOf(this.runtimes, this.count); }
@Override public void resetRunTimes() { for (int i = 0; i < MAX; i++) { this.runtimes[i] = 0; } } }
BubbleSort Class
You will write the BubbleSort.java class which will inherit from RunTime.java and implement the Sort Interface using the Bubble Sort algorithm. The interface may be downloaded from SortInterface.java
Please note that your sort method must measure the run time and add it to the RunTime class by using the addRunTime() method.
Sort Interface
/** * * This interface will be used by various classes to sort an array Integer
objects. * You will write three classes that implement this interface: * *
- *
- BubbleSort *
- InsertionSort *
- SelectionSort *
public interface SortInterface {
/** * * This method is called to sort the given array of Integer
objects. At the * completion of this method, the array will be sorted. * * @param arrayToSort This is the array that contains all the Integer
objects * that need to be sorted. */ public void sort(Integer[] arrayToSort); }
SelectionSort Class
You will write the SelectionSort.java class which will inherit from RunTime.java and implement the Sort Interface using the Selection Sort algorithm. The interface may be downloaded from SortInterface.java
Please note that your sort method must measure the run time and add it to the RunTime class by using the addRunTime() method.
InsertionSort Class
You will write the InsertionSort.java class which will inherit from RunTime.java and implement the Sort Interface using the Insertion Sort algorithm. The interface may be downloaded from SortInterface.java
Please note that your sort method must measure the run time and add it to the RunTime class by using the addRunTime() method.
Driver Class
You will write the Driver.java class which will implement the Driver Interface . The interface may be downloaded from DriverInterface.java
Output From Driver Main Method
Please note that, in addition to implementing the DriverInterface, you are also required to write your own public static main(String[] args) method in Driver.java.
Driver Interface
/** * * @author Sameh A. Fakhouri * */ public interface DriverInterface {
/** * This enum
is used to specify the type of Array. All arrays used in this * assignment will be arrays of Integer: * *
- *
- Equal - The elements in the array are all equal. *
- Random - The elements in the array are randomly generated. *
- Increasing - The elements of the array are arranged in increasing order. *
- Decreasing - The elements of the array are arranged in decreasing order. *
- IncreasingAndRandom - The first 90% of the elements are arranged in increasing order and the last 10% * of the elements are randomly generated. *
enum
is used to specify the desired sort algorithm: * * - *
- BubbleSort - Indicates the Bubble Sort algorithm. *
- SelectionSort - Indicates the Selection Sort algorithm. *
- InsertionSort - Indicates the Insertion Sort algorithm. *
}
Your main() method will have to call the runSort() method to sort each of the following array types ten times for each sort algorithm:
1,000 equal Integers.
1,000 random Integers.
1,000 increasing Integers.
1,000 decreasing Integers.
1,000 increasing and random Integers.
10,000 equal Integers.
10,000 random Integers.
10,000 increasing Integers.
10,000 decreasing Integers.
10,000 increasing and random Integers.
For each call to the runSort() method to sort an ArrayType using a SortType ten times, your main() method will produce the following output:
SortType, ArrayType, Array Size -------------------------------------------------------------------------------------------------------------- runTime1 runTime2 runTime3 runTime4 runTime5 runTime6 runTime7 runTime8 runTime9 runTime10 --- Average runTime
Step by Step Solution
There are 3 Steps involved in it
Step: 1
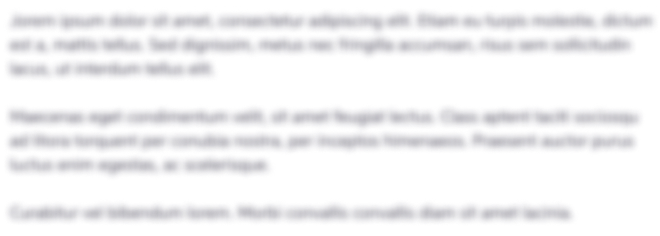
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started