Question
Implementing functions FOR PYTHON 3. How would I complete functions such as these? make_empty_board(n): make an empty n by n board. Empty boards are filled
Implementing functions FOR PYTHON 3. How would I complete functions such as these?
make_empty_board(n): make an "empty" n by n board. Empty boards are filled with None. Assume n > 0. Note: you must create a board with n separate rows instead of n shallow copies of one row. o make_empty_board(1) [[None]] o make_empty_board(2) [[None,None],[None,None]] o make_empty_board(4) [[None,None,None,None], [None,None,None,None], [None,None,None,None], [None,None,None,None]]
is_valid_location(loc, puzzle): given a location tuple (row, col) determine if those coordinates are valid locations in the puzzle. You do not need to check if value is valid. Note only non-negative indexes are allowed to use. o is_valid_location((1,1), [[None]]) False # (0,0) is the only valid location o is_valid_location((1,1), [[1,2],[2,1]]) True # 2x2 puzzle has a row 1 and col 1 o is_valid_location((1,2), [[1,2],[2,1]]) False # 2x2 puzzle has a row 1, but not a col 2
is_complete(puzzle): determine if the puzzle has any remaining None locations. You do not need to check if the puzzle is a valid solution. o is_complete([[1,2,3],[3,1,2],[2,3,1]]) True o is_complete([[1,1,1],[1,1,1],[1,1,1]]) True o is_complete([[1,2],[None,1]]) False o is_complete([[None,None],[None,None]]) False
get_value_at_location(puzzle, loc): given a location as a tuple (row, col), return the value at that location of the given puzzle. You may assume that the location provided is a valid location. o get_value_at_location([[1]], (0,0)) 1 o get_value_at_location([[1,2],[None,1]], (1,0)) None o get_value_at_location([[1,2],[2,1]], (1,1)) 1 o get_value_at_location([[1,2,3],[2,3,1],[3,1,2]], (2,1)) 1
set_location(puzzle, value, loc): given a puzzle, a value, and a location (row, col), try to set the puzzle location to that value. If the puzzle location is empty, set the value and return True. If the location is already set, or the location is invalid, do not modify the puzzle and return False. You do not need to check if value is valid. (HINT: use is_valid_location() to check for valid locations.) o set_location([[1]], 1, (0,0)) False o set_location([[None]], 1, (0,0)) True # puzzle is now [[1]] o set_location([[None]], 1, (1,0)) False # invalid location o set_location([[None]], 3, (0,0)) True # puzzle is now [[3]] o set_location([[1,2],[None,1]], 2, (1,0)) True # puzzle is now [[1,2],[2,1]]
unset_location(puzzle, loc): given a puzzle and a location (row, col), try to remove the value at that location (change to None). Return True if the puzzle was successfully changed (the value at that location is removed). Return False if the location is not valid, or there is currently no value at that location. (HINT: use is_valid_location() to check for valid locations.) o unset_location([[1]], (0,0)) True # puzzle is now [[None]] o unset_location([[1]], (0,1)) False # location is invalid o unset_location([[None]], (0,0)) False # location is already None o unset_location([[1,2],[1,1]], (1,0)) True # puzzle is now [[1,2],[None,1]]
get_size(puzzle): given a puzzle, return its size as an integer. You do not need to check whether the given puzzle is valid. o get_size([[None,None],[None,None]]) 2 #2x2 puzzle o get_size([[1,2,3],[2,1,3],[None,None,3]]) 3 #3x3 puzzle o get_size([[5]]) 1 #1x1 puzzle
get_valid_numbers(size): given a positive size, return a list of the numbers which can be validly placed in a puzzle of that size. o get_valid_numbers(2) [1,2] #2x2 puzzle o get_valid_numbers(3) [1,2,3] #3x3 puzzle o get_valid_numbers(1) [1] #1x1 puzzle
contains_only_valid_symbols(puzzle, complete): check if a puzzle contains only valid numbers. If the puzzle is incomplete, also allow None in the puzzle. complete will be a boolean indicating whether or not the puzzle is complete. You do not need to check that the puzzle is valid, only if the numbers used are correct. (HINT: use get_valid_numbers().) o contains_only_valid_symbols([[1]], True) True # contains 1, the only valid value o contains_only_valid_symbols([[None]], True) False # complete puzzle should not contain None o contains_only_valid_symbols([[None]], False) True # puzzle is incomplete, so None is ok o contains_only_valid_symbols([[1,1],[1,1]], True) True # puzzle contains only valid values (1s and 2s)
has_repeat(xs, v): given a list of numbers xs and a number n, check whether xs has repeated values of n. Return True if v occurs more than once in xs; return False otherwise. You may not use .count()! o has_repeat([None], 1) False o has_repeat([1,2,3], 1) False o has_repeat([1,2,1], 1) True
get_row(puzzle, row_index): return the row at the specified row_index from puzzle as a list. Return None if the row_index is invalid. o get_row([[1,None],[2,None]], 0) [1, None] # row 0 o get_row([[1,None],[2,None]], 1) [2, None] # row 1 o get_row([[1,None],[2,None]], 2) None # invalid index
is_valid_row(puzzle, row_index, complete): given a puzzle, determine if the row at row_index is a valid set of numbers (containing only valid values and unique values). complete is a boolean indicating if the row may contain None values. As before, if the puzzle is incomplete, also allow None, if it is complete, do not allow None. Also note that it is OK to have multiple None values in one row. (HINT: use get_valid_numbers(), get_row() and has_repeat().) board = [[2,4,None,None],[1,2,3,3],[3,1,None,5],[4,3,None,None]] o is_valid_row(board, 0, False) True # ok to have None if incomplete o is_valid_row(board, 0, True) False # cannot have None if complete o is_valid_row(board, 1, True) False # two 3s in same row o is_valid_row(board, 2, False) False # invalid value 5 o is_valid_row(board, -2, True) False # not even a valid row
has_valid_rows(puzzle, complete): determine if all the rows in puzzle are valid. complete is a boolean indicating if the rows should not contain None values. If incomplete allow None, if complete, do not allow None. (HINT: use get_row() and is_valid_row() as part of your solution). o has_valid_rows([[1,2],[1,2]], True) True o has_valid_rows([[1,None],[1,2]], False) True o has_valid_rows([[1,None],[1,2]], True) False # cannot have None if complete o has_valid_rows([[1,4],[1,2]], True) False # row 0 has a 4 in a 2x2 puzzle
get_column(puzzle, col_index): return the column with specified col_index from puzzle as a list. Return None if col_index is invalid. o get_column([[1,None],[2,None]], 0) [1, 2] # col 0 o get_column([[1,None],[2,None]], 1) [None, None] # col 1 o get_column([[1,None],[2,None]], 2) None # invalid index
is_valid_col(puzzle, col_index, complete): similar to is_valid_row but checking the validity of specified column instead. (HINT: use get_valid_numbers(), get_column() and has_repeat().) board = [[2,1,None,None],[None,2,3,3],[3,1,None,5],[4,3,None,None]] o is_valid_col(board, 0, False) True # ok to have None if incomplete o is_valid_col(board, 0, True) False # cannot have None if complete o is_valid_col(board, 1, True) False # two 1s in same column o is_valid_col(board, 3, False) False # invalid value 5 o is_valid_col(board, 7, True) False # not even a valid column
has_valid_cols(puzzle, complete): determine if all the columns in puzzle are valid. complete is a boolean indicating if the columns may contain None values. If incomplete allow None, if complete, do not allow None. (HINT: use is_valid_col() and get_column() as part of your solution). o has_valid_cols([[1,2],[1,1]], True) False # two 1s in col 0 o has_valid_cols([[1,None],[2,1]], False) True o has_valid_cols([[1,None],[2,1]], True) False # cannot have None if complete o has_valid_cols([[1,4],[2,1]], True) False # col 1 has a 4 in a 2x2 puzzle
is_valid_cage_solution(puzzle, op, expected_total, locations): given a puzzle, an operation op, an integer expected_total, and a list of location tuples(e.g. a "cage" in a KenkKen puzzle), determine if the puzzle locations total correctly for the operation. You do not need to check if the rows/columns of the puzzle are valid. 7 Assumptions: o the puzzle is complete o the operation will be one of: '+', '-', or 'x' o subtraction operations will always involve two (and only two) locations o subtraction operations pass in the absolute value of the operation (see examples) o the locations will be valid and in a list in the form: [(row, col), (row, col), ..., (row, col)] Examples: o is_valid_cage_solution([[1]], '+', 1, [(0,0)]) True # location (0,0) sums to 1 o is_valid_cage_solution([[1,2],[2,1]], '-', 1, [(0,0),(1,0)]) True # the absolute value of location (0,0) location (1,0) is 1 o is_valid_cage_solution([[1,2],[2,1]], '-', 1, [(1,0),(0,0)]) True # the absolute value of location (1,0) location (0,0) is 1 o is_valid_cage_solution([[1,2],[2,1]], 'x', 4, [(0,0),(0,1),(1,0),(1,1)]) True # all values in the puzzle multiplied together = 4 o is_valid_cage_solution([[1,1],[1,1]], 'x', 1, [(0,0)]) True # location (0,0) multiplies to 1 o is_valid_cage_solution([[1,2],[2,1]], '+', 4, [(0,0),(0,1)]) False # location (0,0) and (0,1) do not sum to 4
is_valid(puzzle, cages, complete): determine if the given puzzle is currently in a valid state: if incomplete, check whether all rows/columns are valid; if complete also check cages and determine if the puzzle locations total correctly for the operation. (HINT: use has_valid_rows(), has_valid_cols(), and is_valid_cage_solution().) board_1 = [[3,1,2],[2,3,1],[1,2,3]] board_2 = [[3,1,2],[2,2,1],[1,2,3]] o is_valid(board_1, [], False) True o is_valid(board_1, [['+',5,[(0,0),(1,0)]]], True) True o is_valid(board_1, [['+',4,[(0,0),(1,0)]]], True) False # not valid cage o is_valid(board_2, [['+',5,[(0,0),(1,0)]]], True) False # invalid row o is_valid([[None, None],[None,None]], [['+',5,[(0,0),(1,0)]]], False) True
get_board_string(puzzle): return a string representation of a puzzle which, when printed, would display an ascii art version of the puzzle. You may assume a puzzle of size 9x9 or smaller for this method only. Example: For the puzzle [3,1,2],[2,None,None],[None,None,None]] the string would be: " [0] [1] [2] ------------- [0] | 3 | 1 | 2 | [1] | 2 | . | . | [2] | . | . | . | -------------" Which printed would display as: [0] [1] [2] ------------- [0] | 3 | 1 | 2 | [1] | 2 | . | . | [2] | . | . | . | ------------- Notes: (a) Columns are three characters wide (a space, a number, and a space) with a dividers (|). (b) For the dashed lines ------ we need just enough to cover the top and bottom of the puzzle, no matter what size the puzzle is (one dash to start, then four dashes per column). To make the dashes appear in the correct place, there are also four spaces to the left. (c) The column headers ([0], [1], etc.) are centered on the columns and the row headers ([0], [1], etc.) have a single space between them and the first |. There are no leading spaces for the row headers.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
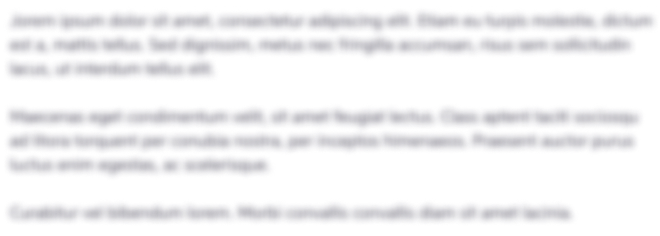
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started