Question
import java.awt.Container; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JTextField; public class Calculator extends JFrame implements ActionListener { private
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTextField;
public class Calculator extends JFrame implements ActionListener
{
private JTextField displayText = new JTextField(30);
private JButton[] button = new JButton[19];
private String[] keys = { "7","8","9","/","4","5","6","*","1","2","3","-","0","C","=","+","M","R","E"};
private String numStr1 = "";
private String numStr2 = "";
private String memory = "";
private char op;
private boolean firstInput = true;
public Calculator()
{
setTitle("Calculator");
setSize(230,230);
Container pane = getContentPane();
pane.setLayout(null);;
displayText.setSize(200,30);;
displayText.setLocation(10,10);
pane.add(displayText);
int x,y;
x=10;
y=40;
for(int ind=0;ind<19;ind++)
{
button[ind] = new JButton(keys[ind]);
button[ind].addActionListener(this);
button[ind].setSize(50, 30);
button[ind].setLocation(x,y);
pane.add(button[ind]);
x = x + 50;
if((ind +1) % 4 == 0)
{
x=10;
y=y+30;
}
}
this.addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent e)
{
System.exit(0);
}
}
);
//java.awt.event.ActionListener.actionPerformed()
setVisible(true);
}
public void actionPreformed(ActionEvent ae)
{
String resultStr;
String str = String.valueOf(ae.getActionCommand());
char ch = str.charAt(0);
switch(ch)
{
case'0':
case'1':
case'2':
case'3':
case'4':
case'5':
case'6':
case'7':
case'8':
case'9':
if(firstInput)
{
numStr1 = numStr1 +ch;
displayText.setText(numStr1);
}
else
{
numStr2 = numStr2 + ch;
displayText.setText(numStr2);
}
break;
case'+':
case'-':
case'*':
case'/':
op = ch;
firstInput = false;
break;
case'=':
resultStr = evaluate();
displayText.setText(resultStr);
numStr1 = resultStr;
numStr2 = "";
firstInput = false;
break;
case'C':
displayText.setText("");
numStr1 = "";
numStr2 = "";
firstInput = true;
case 'M':
memory = displayText.getText();
break;
case'R':
displayText.setText(memory);
numStr1 = memory;
break;
case 'E':
System.exit(0);
}
}
private String evaluate()
{
final char beep = '\u0007';
try
{
int num1 = Integer.parseInt(numStr1);
int num2 = Integer.parseInt(numStr2);
int result = 0;
switch(op)
{
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
}
return String.valueOf(result);
}
catch(ArithmeticException ae)
{
System.out.print(beep);
return "E R R O R: " + ae.getMessage();
}
catch(NumberFormatException nfe)
{
System.out.print(beep);
if(numStr1.equals(""))
return "E R R O R: Invalid First Number";
else
return "E R R O R: Invalid Second Number";
}
catch(Exception e)
{
System.out.println(beep);
return " E R R O R";
}
}
public static void main(String[] args)
{
Calculator C = new Calculator();
}
}
Can you help me fix my JAVA program?
I beleive i need to implement java.awt.event.ActionListener.actionPerformed()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
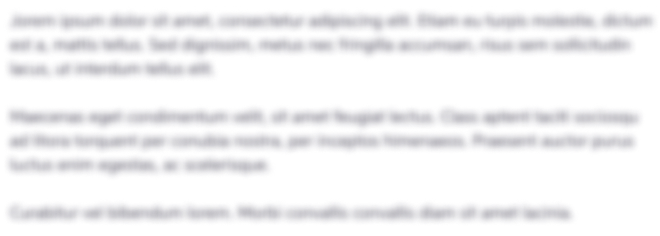
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started